How to Join Two DataFrames in Pandas Using a Full Outer Join
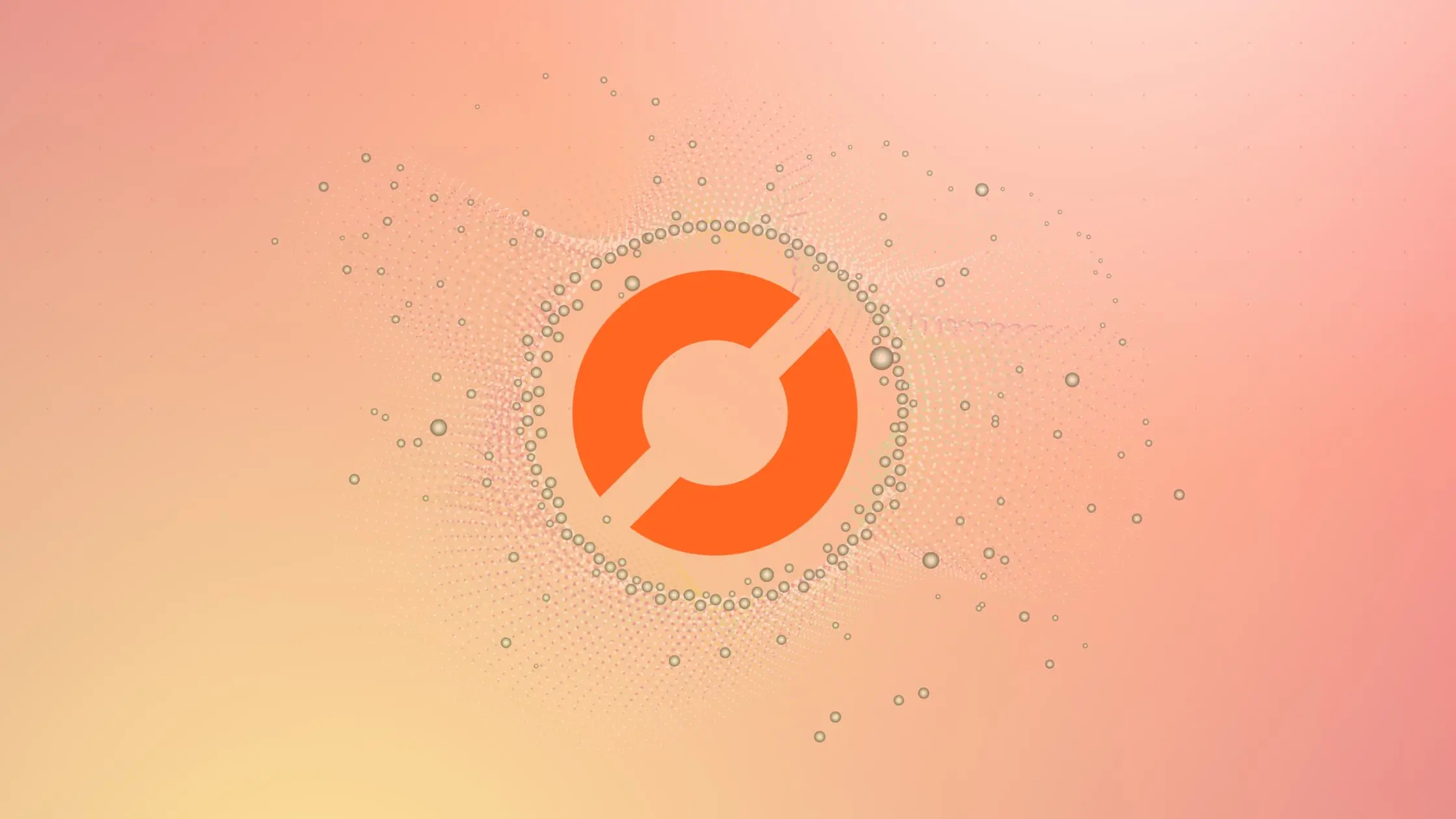
As a data scientist or software engineer, you often find yourself working with data that is spread across multiple tables or spreadsheets. In order to analyze this data, you need to bring it all together into a single table. This process is known as joining, and it is an essential skill for anyone working with data.
There are several different types of joins that you can use to combine two or more tables. In this article, we will focus on the full outer join, which is a type of join that returns all the rows from both tables, and fills in any missing values with NaN (not a number).
What is a Full Outer Join?
A full outer join, also known as a full join, combines the rows from two tables, and includes all the rows from both tables, even if there is no match in the other table. If a row in one table has no matching row in the other table, then the missing values are filled in with NaN.
For example, let’s say you have two tables: a table of customers and a table of orders. The customers table has one row for each customer, and the orders table has one row for each order. Each row in the orders table has a customer ID that matches a customer ID in the customers table.
If you want to combine these two tables into a single table that includes all the information about each customer and their orders, you can use a full outer join. This will include all the rows from both tables, even if there are customers who have not yet placed any orders, or orders that have not yet been assigned to a customer.
How to Perform a Full Outer Join in Pandas
Pandas is a popular data manipulation library in Python that provides powerful tools for working with data. To perform a full outer join in Pandas, you can use the merge()
function.
The merge()
function takes two dataframes as arguments, and joins them on a specified column or index. By default, merge()
performs an inner join, which only includes the rows that have a match in both tables. To perform a full outer join, you need to specify the how
parameter as 'outer'
.
Here is an example of how to perform a full outer join in Pandas:
import pandas as pd
# create two dataframes
customers = pd.DataFrame({
'customer_id': [1, 2, 3, 4],
'name': ['Alice', 'Bob', 'Charlie', 'Dave']
})
orders = pd.DataFrame({
'order_id': [101, 102, 103, 104],
'customer_id': [2, 3, 5, 1],
'order_total': [25.00, 50.00, 10.00, 100.00]
})
# perform a full outer join on the customer_id column
merged = pd.merge(customers, orders, on='customer_id', how='outer')
print(merged)
This will output the following table:
customer_id name order_id order_total
0 1 Alice 104.0 100.00
1 2 Bob 101.0 25.00
2 3 Charlie 102.0 50.00
3 4 Dave NaN NaN
4 5 NaN 103.0 10.00
As you can see, the resulting table includes all the rows from both the customers and orders tables, even though there is no matching customer for order 103, and no matching order for customer 4.
Pros and Cons of Using Full Outer Joins in Pandas:
Pros:
- Inclusive Combination: The full outer join includes all rows from both tables, ensuring that no data is omitted. This is useful when you want a comprehensive view, even if some rows don’t have matches in the other table.
- Missing Value Handling: The full outer join automatically fills in missing values with NaN, providing clear indicators for unmatched rows and allowing for easy identification of gaps in the data.
- Flexible Matching: The full outer join is not restricted by the presence of matching values; it combines data based on existing matches but retains all rows, making it suitable for various scenarios.
- Versatility in Data Structures: The
merge()
function in Pandas supports the full outer join across different types of data structures, making it versatile for joining diverse datasets.
Cons:
- Potential for Large Output: In cases where both tables have a substantial number of rows, the resulting merged table from a full outer join can become large, potentially impacting performance and readability.
- Complexity in Data Exploration: Dealing with NaN values introduced by the full outer join might require additional preprocessing or handling strategies, adding complexity to the subsequent data analysis.
- Memory Consumption: The merged table generated by a full outer join consumes memory proportional to the size of the input tables, and for large datasets, this could lead to increased memory usage.
Common Errors and How to Handle:
- Mismatched Column Names:
Error: Performing a full outer join on columns with different names can result in errors or unexpected output.
Handling: Ensure that the columns used for joining have identical names in both tables, or explicitly specify the left_on
and right_on
parameters if the column names differ.
# Explicitly specifying the columns for joining
merged = pd.merge(customers, orders, left_on='customer_id', right_on='customer_id', how='outer')
- Overlapping Column Names:
Error: If both tables have columns with the same name, the resulting merged table may have ambiguous column labels. Handling: Rename the overlapping columns before performing the join to avoid naming conflicts.
# Rename columns before merging
customers.rename(columns={'name': 'customer_name'}, inplace=True)
orders.rename(columns={'name': 'order_name'}, inplace=True)
merged = pd.merge(customers, orders, on='customer_id', how='outer')
- Missing how Parameter:
Error: Forgetting to specify the how
parameter as 'outer'
in the merge()
function will default to an inner join.
Handling: Ensure the how
parameter is explicitly set for a full outer join.
# Perform a full outer join
merged = pd.merge(customers, orders, on='customer_id', how='outer')
- Handling Ambiguity in Merged Columns:
Error: When both tables have columns with similar names but different meanings, it can lead to confusion in the resulting merged table.
Handling: Use the suffixes parameter to add suffixes
to the overlapping column names, making them distinct.
# Add suffixes to distinguish overlapping columns
merged = pd.merge(customers, orders, on='customer_id', how='outer', suffixes=('_customer', '_order'))
By addressing these common errors and considering the pros and cons, you can effectively utilize a full outer join in Pandas for combining data from multiple sources.
Conclusion
Joining two or more tables is an essential skill for anyone working with data, and the full outer join is a powerful tool for combining data from multiple sources. In this article, we have shown you how to perform a full outer join in Pandas using the merge()
function.
By following the steps outlined in this article, you should now be able to join two dataframes in Pandas using a full outer join, and start analyzing your data more effectively.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.