How to Insert a Row to Pandas DataFrame
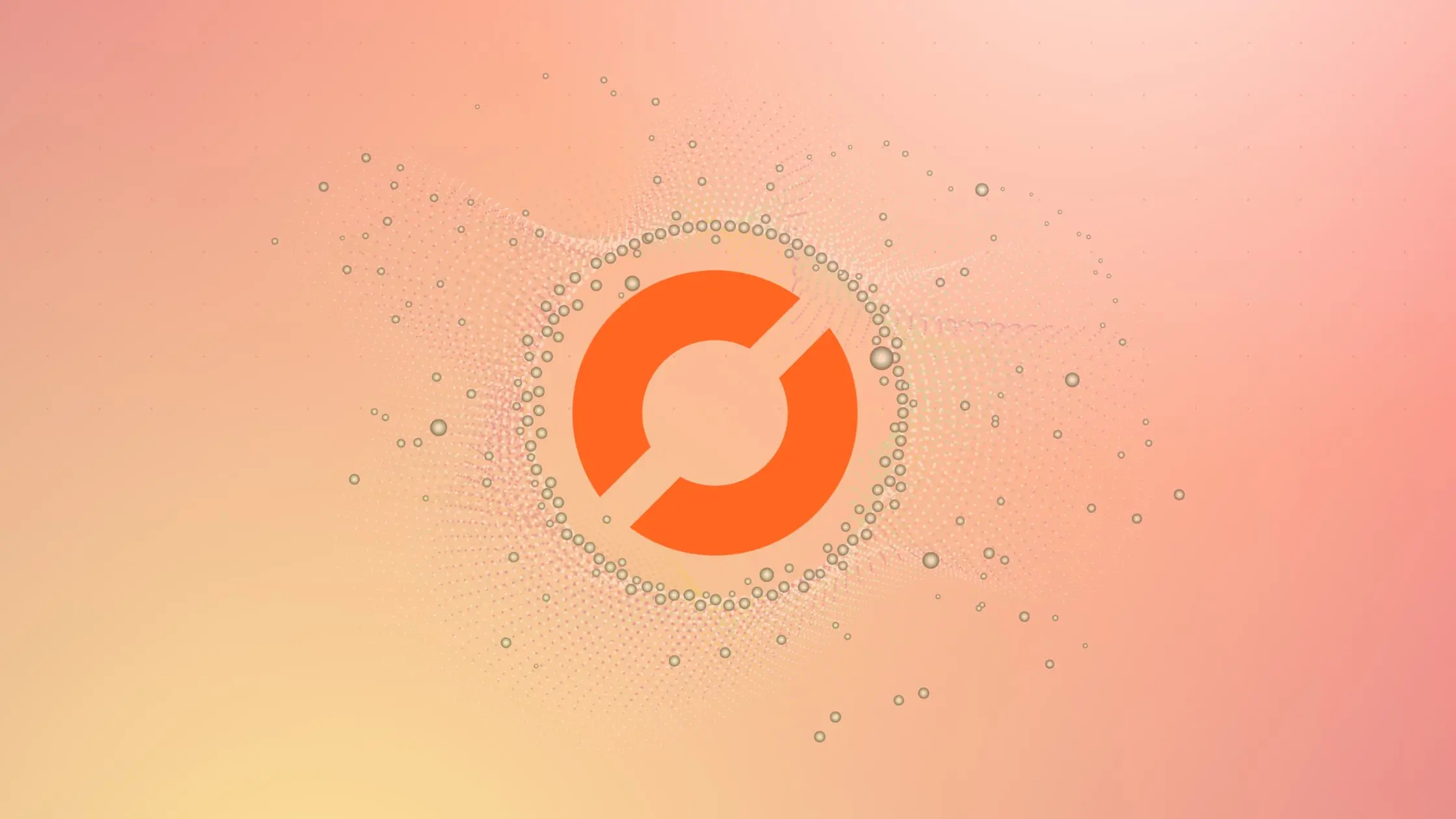
As a data scientist, one of the most common tasks you will encounter is working with pandas DataFrames. Pandas is a powerful library for data manipulation and analysis in Python that provides comprehensive data structures for working with tabular data. In this article, we will discuss how to insert a row to a pandas DataFrame.
What Is a Pandas DataFrame?
Before we dive into inserting a row to a pandas DataFrame, let’s first understand what a DataFrame is. A DataFrame is a two-dimensional labeled data structure with columns of potentially different types. It is similar to a spreadsheet or a SQL table, but with optimized performance and functionality for data analysis.
How to Insert a Row to a Pandas DataFrame
To insert a row to a Pandas DataFrame, the append
or loc
method can be employed. Below are the typical steps for accomplishing this task:
- Create a dictionary with the data for the new row
- Append the dictionary to the DataFrame using the
append
orloc
method - Reset the index if needed
Using append method
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]
})
# Create a dictionary with the data for the new row
new_row = {'Name': 'David', 'Age': 40}
# Append the dictionary to the DataFrame
df = df.append(new_row, ignore_index=True)
# Reset the index
df = df.reset_index(drop=True)
print(df)
Output:
Name Age
0 Alice 25
1 Bob 30
2 Charlie 35
3 David 40
As we can see in the example above, we first create a DataFrame with three rows and two columns. Then, we create a dictionary with the data for the new row, which includes the name and age of the person we want to add. We then append the dictionary to the DataFrame using the append
method, and set the ignore_index
parameter to True
to generate a new index for the DataFrame. Finally, we reset the index using the reset_index
method to ensure that the index is sequential and starts from 0.
Using loc method
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]
})
# Create a dictionary with the data for the new row
new_row = {'Name': 'David', 'Age': 40}
# Inserting the new row
df.loc[len(df)] = new_row
# Reset the index
df = df.reset_index(drop=True)
print(df)
Output:
Name Age
0 Alice 25
1 Bob 30
2 Charlie 35
3 David 40
In the code above, the new_row
dictionary contains the information for the person we want to add. Using df.loc[len(df)]
, we insert the new row at the end of the DataFrame. The length of the DataFrame (len(df))
serves as the index for the new row.
Conclusion
In this article, we have learned how to insert a row to a pandas DataFrame. Pandas is a powerful library for data manipulation and analysis in Python, and being able to insert rows to a DataFrame is a fundamental skill for any data scientist or software engineer working with tabular data. By following the simple steps outlined in this article, you can easily add new rows to your DataFrame and continue your data analysis with confidence.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.