How to Insert a List into a Cell in Python Pandas
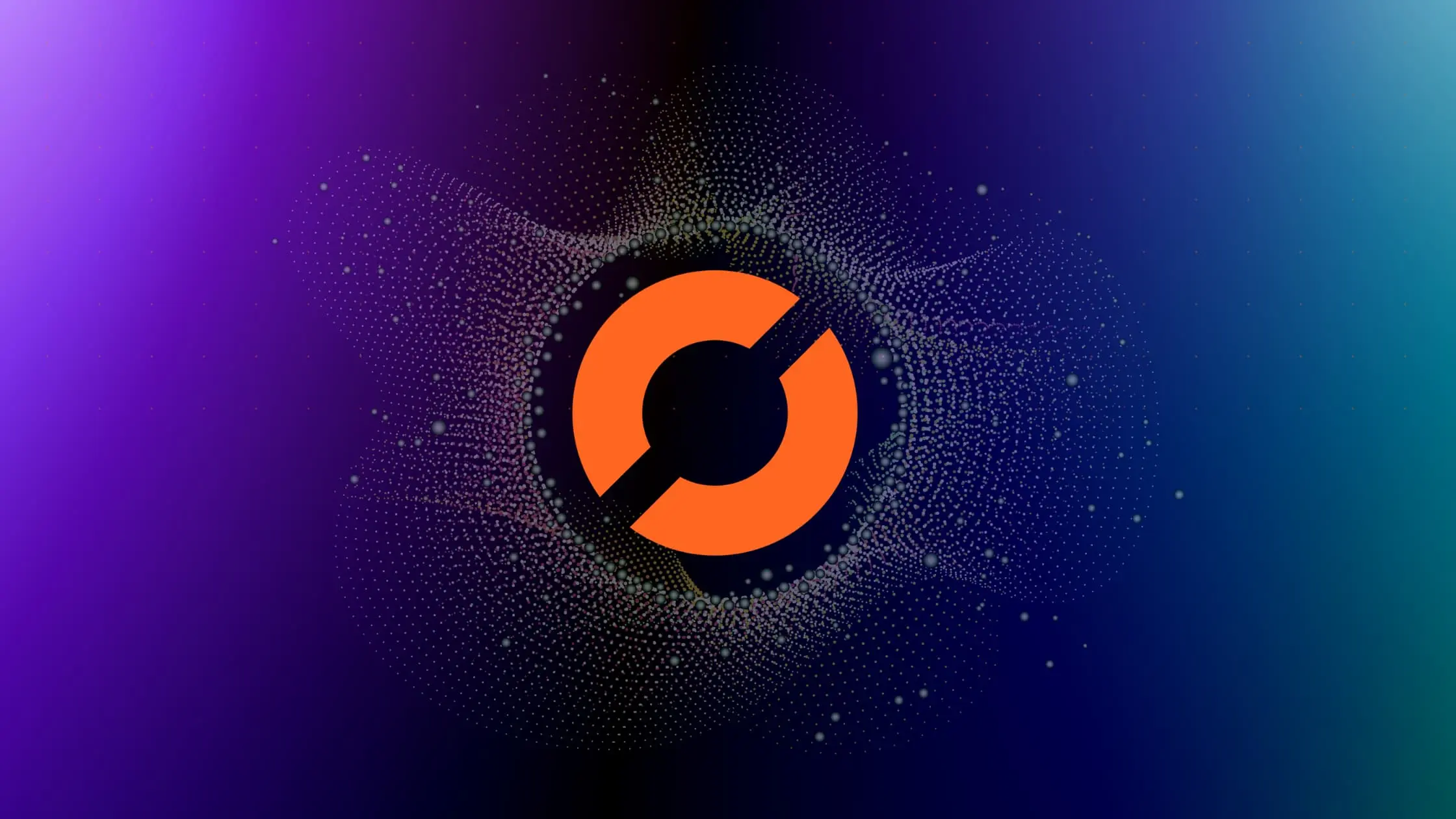
As a data scientist, you’re likely to come across situations where you need to insert a list into a cell of a Pandas DataFrame. Python Pandas is a powerful library for data manipulation, and it provides several ways to insert data into a DataFrame. In this article, we will discuss how to insert a list into a cell of a Pandas DataFrame.
What is Pandas?
Pandas is a popular Python library for data manipulation and analysis. It provides data structures to efficiently store and manipulate large datasets. The two main data structures in Pandas are Series and DataFrame. A Series is a one-dimensional labeled array that can hold any data type. A DataFrame is a two-dimensional labeled data structure with columns of potentially different types.
How to Insert a List into a Cell in Pandas DataFrame
To insert a list into a cell of a Pandas DataFrame, we can use the iloc
or loc
method. The iloc
method is used to access rows and columns of a DataFrame by integer position, while the loc
method is used to access rows and columns by label.
Using iloc
Let’s create a simple Pandas DataFrame to demonstrate how to insert a list into a cell using the iloc
method.
import pandas as pd
# Sample DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [20, 22, 21],
'Grade': [None, None, None]} # Initialize Grade column with None
df = pd.DataFrame(data)
# List of grades to be inserted
grades_to_insert = [95, 88, 92]
# Display df
print(df)
Output:
Name Age Grade
0 Alice 20 None
1 Bob 22 None
2 Charlie 21 None
In this example, the DataFrame has three columns: Name
, Age
, and Grade
. The Grade
column is initialized with None, and we want to insert the values from the grades_to_insert list into the ‘Grade’ column for each respective student.
# Inserting the list of grades into the 'Grade' column using iloc
df.iloc[:, 2] = grades_to_insert
# Display df
print(df)
Here, df.iloc[:, 2]
selects all rows in the Grade
column. The right-hand side of the assignment (grades_to_insert) assigns the list of grades to the selected cells. The resulting DataFrame will look like this:
Name Age Grade
0 Alice 20 95
1 Bob 22 88
2 Charlie 21 92
Using loc
We can also use the loc
method to insert a list into a cell of a Pandas DataFrame. Let’s modify the previous example to use the loc
method.
# Assign the list to Grade column
df.loc[:, 'Grade'] = grades_to_insert
print(df)
Here, we are using the loc
method to access all rows in the Grade
column and assigning a list grades_to_insert
to it. The resulting DataFrame will be the same as the previous example.
Output:
Name Age Grade
0 Alice 20 95
1 Bob 22 88
2 Charlie 21 92
Conclusion
In this article, we explored how to use the iloc
and loc
methods in Pandas to insert a list of values into a specific cell in a DataFrame. While loc
excels in label-based indexing, offering an intuitive approach for selecting and modifying data based on labels or boolean arrays, iloc
is valuable for its proficiency in index-based indexing. Understanding the loc method enhances your ability to manipulate and update data in a Pandas DataFrame with greater flexibility.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.