How to Initialize Numpy Array of List Objects: A Guide
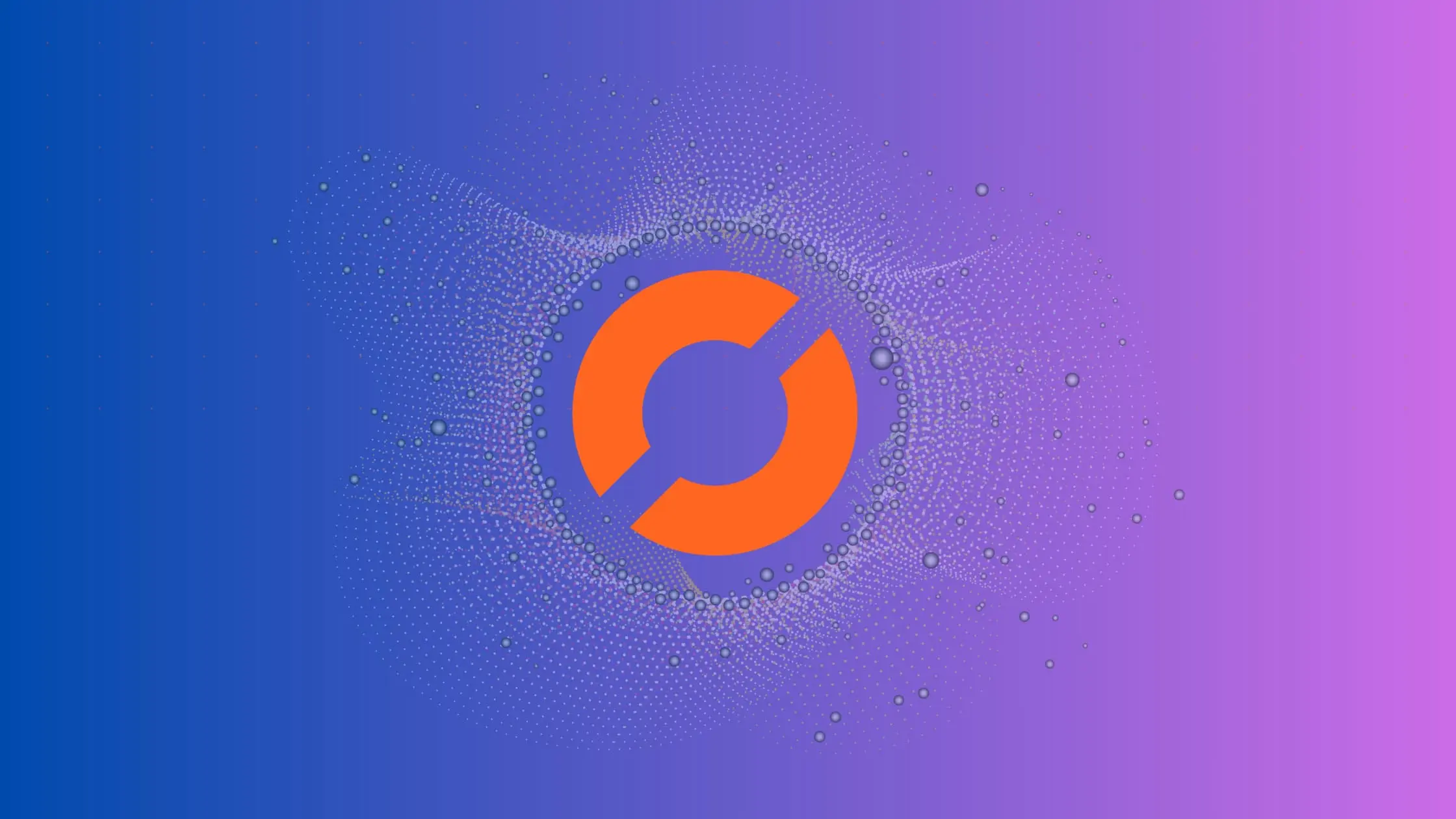
In the world of data science, Numpy is a fundamental library that provides a high-performance multidimensional array object. It’s a go-to tool for numerical operations. Today, we’ll delve into a specific aspect of Numpy: initializing an array of list objects.
Table of Contents
What is Numpy?
Numpy, or Numerical Python, is a library for the Python programming language, adding support for large, multi-dimensional arrays and matrices, along with a large collection of high-level mathematical functions to operate on these arrays.
Why Use Numpy Arrays?
Numpy arrays are memory-efficient and provide fast numerical operations. They are a great alternative to Python Lists. Here are some reasons why you should consider using Numpy arrays:
- Performance: Numpy data structures perform better in:
- Size - Numpy data structures take up less space
- Speed - they have a need for speed and are faster than lists
- Functionality: SciPy and NumPy have optimized functions such as linear algebra operations built in.
- Versatility: You can create vectors, matrices, and tensors with Numpy arrays.
Initializing Numpy Array of List Objects
Let’s get to the main topic: how to initialize a Numpy array of list objects. Here’s a step-by-step guide:
Step 1: Import Numpy
First, you need to import the Numpy library. If you haven’t installed it yet, use the pip install command:
pip install numpy
Then, import Numpy in your Python script:
import numpy as np
Step 2: Create a List
Next, create a list or lists that you want to convert into a Numpy array:
list1 = [1, 2, 3, 4]
list2 = [5, 6, 7, 8]
Step 3: Convert List to Numpy Array
Now, use the np.array()
function to convert lists into a Numpy array:
array = np.array([list1, list2])
This will create a 2D Numpy array.
Step 4: Verify the Type
To ensure that your list has been converted to a Numpy array, use the type()
function:
print(type(array))
If the output is <class 'numpy.ndarray'>
, congratulations! You’ve successfully created a Numpy array from a list.
Convert List to Numpy Array using np.asarray()
Instead of using the np.array()
function, you can also use the np.asarray()
method to convert lists into a Numpy array:
array_asarray = np.asarray([list1, list2])
This will achieve the same result as using `np.array()``.
Now, let’s compare the two approaches:
import numpy as np
# Step 2: Create a List
list1 = [1, 2, 3, 4]
list2 = [5, 6, 7, 8]
# Step 3: Convert List to Numpy Array using np.array()
array_nparray = np.array([list1, list2])
# Step 3 (Alternative): Convert List to Numpy Array using np.asarray()
array_asarray = np.asarray([list1, list2])
# Step 4: Verify the Type (using np.array() approach)
print(type(array_nparray))
# Output: <class 'numpy.ndarray'>
# Step 4: Verify the Type (using np.asarray() approach)
print(type(array_asarray))
# Output: <class 'numpy.ndarray'>
Both np.array()
and np.asarray()
methods are commonly used to convert lists into Numpy arrays. The choice between them often comes down to personal preference or specific use cases. np.asarray()
is a bit more flexible and can handle existing Numpy arrays, Python lists, or other iterable objects. The key idea is that both methods facilitate the conversion of lists to Numpy arrays, making it easy to work with numerical data in a more efficient manner.
Conclusion
Numpy is a powerful library that allows you to work with complex data structures more efficiently. Initializing a Numpy array of list objects is a simple process that can greatly optimize your data science projects.
Remember, the key steps are to import the Numpy library, create your list or lists, convert the list to a Numpy array using np.array()
, and verify the type of your new array.
We hope this guide has been helpful in your data science journey. Stay tuned for more tips and tricks on leveraging Python’s powerful libraries!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.