How to Improve TensorFlow Model Accuracy
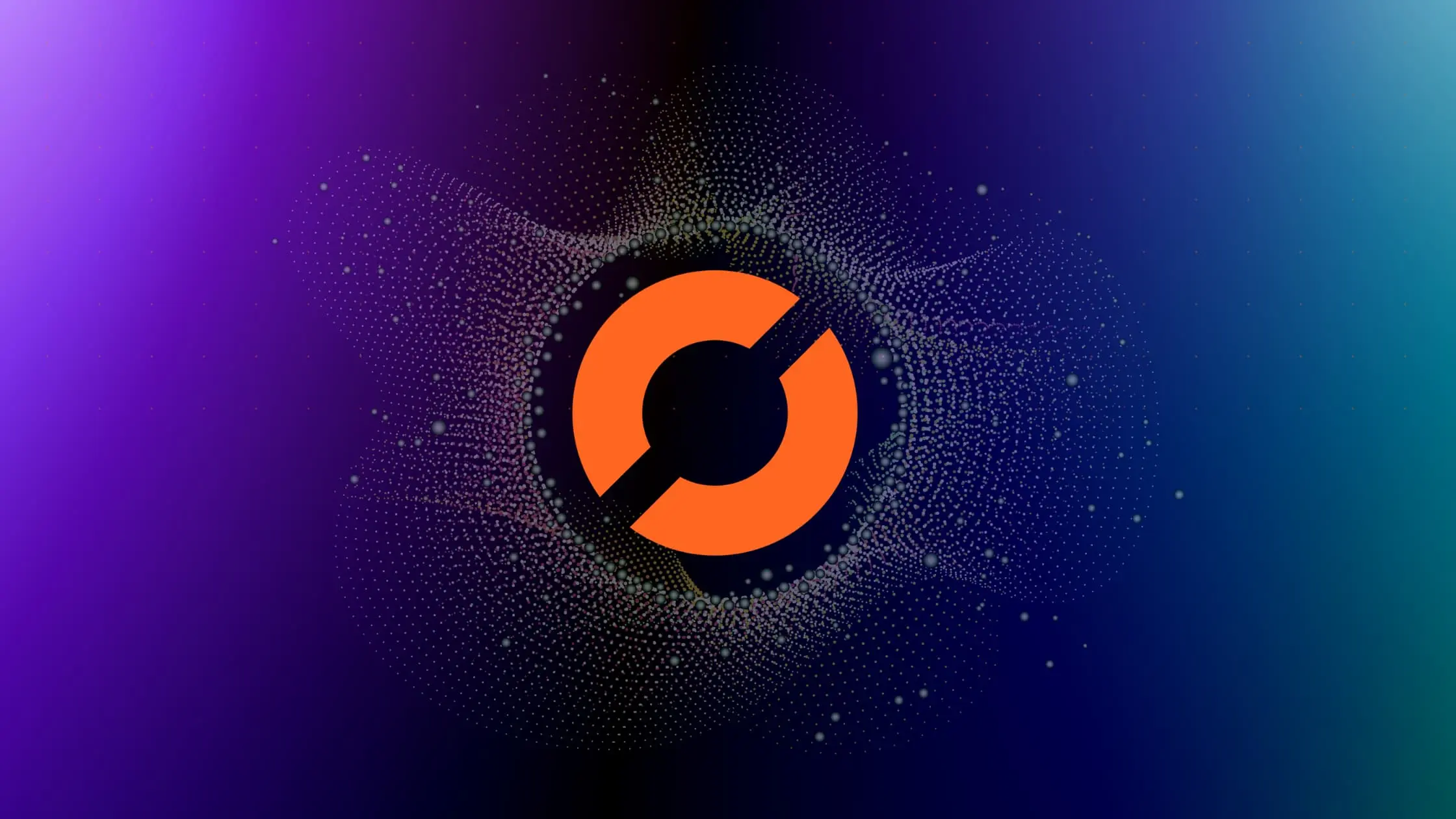
As a data scientist, one of the most important tasks is building machine learning models that can predict outcomes with high accuracy. One popular tool for building these models is TensorFlow, an open-source machine learning framework developed by Google. However, sometimes, even with the best practices in place, your TensorFlow model’s accuracy may not increase. In this post, we’ll explore some common reasons for this issue and provide some tips on how to improve TensorFlow model accuracy.
Table of Contents
- What is TensorFlow?
- Reasons for Low Model Accuracy
- Tips to Improve Model Accuracy
- Pros and Cons of Each Technique
- Common Errors and How to Handle
- Conclusion
What is TensorFlow?
Before diving into the topic, let’s take a quick look at what TensorFlow is and how it works. TensorFlow is a powerful machine learning framework that allows developers to build and train machine learning models. It is based on data flow graphs, where nodes represent mathematical operations, and edges represent the data flowing between them. This architecture makes it easy to parallelize and distribute computations across multiple CPUs or GPUs, making it an ideal tool for building large-scale machine learning models.
Reasons for Low Model Accuracy
Now, let’s explore some common reasons why your TensorFlow model’s accuracy may not be increasing:
Insufficient Data
One of the most common reasons for low model accuracy is insufficient data. Machine learning models require a large amount of data to learn patterns and make accurate predictions. If the training data is too small or doesn’t represent the problem domain well, the model may not be able to learn the underlying patterns and generalize to new data.
To overcome this issue, you can try to collect more data or use data augmentation techniques to increase the size of your training dataset. You can also try to balance the dataset if it’s imbalanced, using techniques like oversampling or undersampling.
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# Create an image data generator with augmentation parameters
datagen = ImageDataGenerator(
rotation_range=20,
width_shift_range=0.2,
height_shift_range=0.2,
shear_range=0.2,
zoom_range=0.2,
horizontal_flip=True,
fill_mode='nearest'
)
# Generate augmented images
augmented_images = datagen.flow(X_train, y_train)
Poor Data Quality
Another reason for low model accuracy is poor data quality. If the data contains errors, missing values, or outliers, the model may not be able to learn the underlying patterns and make accurate predictions. It’s essential to clean and preprocess the data before training the model to ensure that it’s of high quality.
To overcome this issue, you can use techniques like data imputation, outlier detection, or feature scaling to preprocess the data. You can also use data visualization techniques to identify any patterns or outliers in the data.
from sklearn.preprocessing import StandardScaler, MinMaxScaler
# Standardization
scaler = StandardScaler()
X_train_std = scaler.fit_transform(X_train)
X_test_std = scaler.transform(X_test)
# Normalization
minmax_scaler = MinMaxScaler()
X_train_norm = minmax_scaler.fit_transform(X_train)
X_test_norm = minmax_scaler.transform(X_test)
Inappropriate Model Architecture
The model architecture is another critical factor that affects the accuracy of the machine learning model. If the model architecture is too simple, it may not be able to capture the underlying patterns in the data. On the other hand, if the model architecture is too complex, it may overfit the training data and not generalize well to new data.
To overcome this issue, you can try to experiment with different model architectures, such as adding more layers or increasing the number of neurons in each layer. You can also use regularization techniques like L1 or L2 regularization to prevent overfitting.
Inappropriate Hyperparameters
Hyperparameters are the parameters that define the model’s architecture and training process, such as learning rate, batch size, and optimizer. If the hyperparameters are not appropriately set, the model may not be able to learn the underlying patterns in the data and make accurate predictions.
To overcome this issue, you can try to experiment with different hyperparameters and use techniques like grid search or random search to find the best combination of hyperparameters that improve model accuracy.
Tips to Improve Model Accuracy
Now that we’ve explored some common reasons for low model accuracy let’s look at some tips to improve TensorFlow model accuracy:
Use Transfer Learning
Transfer learning is a technique that allows you to reuse a pre-trained model’s knowledge to train a new model on a different task. This technique can be useful when you have limited data or limited computational resources. You can use a pre-trained model as a feature extractor and train a new model on top of it to improve model accuracy.
from tensorflow.keras.applications import VGG16
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Dense, GlobalAveragePooling2D
# Load pre-trained VGG16 model without top layers
base_model = VGG16(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
# Add custom dense layers for the target task
x = base_model.output
x = GlobalAveragePooling2D()(x)
x = Dense(256, activation='relu')(x)
predictions = Dense(10, activation='softmax')(x)
# Create the final model
model = Model(inputs=base_model.input, outputs=predictions)
Use Regularization Techniques
Regularization techniques like L1 or L2 regularization can be used to prevent overfitting and improve model accuracy. L1 regularization adds a penalty term to the loss function that encourages the model to learn sparse weights, while L2 regularization adds a penalty term that encourages the model to learn small weights.
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
from tensorflow.keras.regularizers import l2
from tensorflow.keras.datasets import mnist
from tensorflow.keras.utils import to_categorical
from sklearn.model_selection import train_test_split
# Load and preprocess the MNIST dataset
(X_train, y_train), (X_test, y_test) = mnist.load_data()
X_train, X_val, y_train, y_val = train_test_split(X_train, y_train, test_size=0.1, random_state=42)
X_train = X_train.reshape(-1, 28 * 28) / 255.0
X_val = X_val.reshape(-1, 28 * 28) / 255.0
X_test = X_test.reshape(-1, 28 * 28) / 255.0
y_train = to_categorical(y_train, num_classes=10)
y_val = to_categorical(y_val, num_classes=10)
y_test = to_categorical(y_test, num_classes=10)
# Build a neural network with L2 regularization
model = Sequential()
model.add(Dense(128, activation='relu', kernel_regularizer=l2(0.001), input_shape=(28 * 28,)))
model.add(Dense(64, activation='relu', kernel_regularizer=l2(0.001)))
model.add(Dense(10, activation='softmax'))
# Compile the model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# Train the model with regularization
history = model.fit(X_train, y_train, epochs=10, batch_size=64, validation_data=(X_val, y_val))
# Evaluate the model on the test set
test_loss, test_acc = model.evaluate(X_test, y_test)
print(f'Test Accuracy: {test_acc * 100:.2f}%')
Use Dropout
Dropout is a regularization technique that randomly drops out some of the neurons in the network during training, which helps to prevent overfitting. This technique can be especially useful when dealing with large and complex datasets.
Use Data Augmentation
Data augmentation techniques like rotation, translation, or scaling can be used to increase the size of the training dataset and improve model accuracy. This technique can be especially useful when dealing with limited data.
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Dropout
model = Sequential()
model.add(Dense(128, activation='relu'))
model.add(Dropout(0.2))
model.add(Dense(64, activation='relu'))
model.add(Dropout(0.3))
model.add(Dense(10, activation='softmax'))
Pros and Cons of Each Technique
Data Preprocessing
Pros:
- Improved model convergence: Standardizing and normalizing input data can help the model converge faster during training.
- Mitigates the impact of varying scales: Standardization and normalization ensure that input features are on a similar scale, preventing some features from dominating others.
Cons:
- Sensitivity to outliers: Standardization and normalization can be sensitive to outliers in the data, which might affect their effectiveness.
Adding Dropout Layers
Pros:
- Reduces overfitting: Dropout layers help prevent overfitting by randomly dropping a fraction of neurons during training, forcing the model to learn more robust features.
- Enhances generalization: By preventing co-adaptation of hidden units, dropout promotes the learning of more general features.
Cons:
- Increased training time: The random dropout during training increases the time required for model convergence.
Data Augmentation
Pros:
- Increased model robustness: Augmenting data with random transformations increases the model’s ability to generalize to different variations of the input.
- Mitigates overfitting: Data augmentation provides additional training examples, reducing the risk of overfitting on a limited dataset.
Cons:
- Increased training time: Generating augmented data on-the-fly during training increases the overall training time.
Transfer Learning
Pros:
- Effective with limited data: Transfer learning is particularly effective when you have limited labeled data for the target task.
- Reduces training time: Leveraging a pretrained model saves training time compared to training a model from scratch.
Cons:
- Domain-specific features might not be captured: The pre-trained model might not capture specific features relevant to the target task, requiring fine-tuning or adjustments.
Regularization Techniques
Pros:
- Prevents overfitting: L2 regularization adds a penalty term to the loss function, preventing the model from fitting the training data too closely.
- Encourages weight sparsity: L2 regularization encourages the model to use smaller weights, leading to a more compact and interpretable model.
Cons:
- Not suitable for all cases: L2 regularization might not be effective in scenarios where some degree of feature selection is desired.
- May not handle correlated features well: L2 regularization may not handle highly correlated features effectively.
These pros and cons provide insights into the considerations and trade-offs associated with each technique. It’s important to carefully select and combine these methods based on the characteristics of your data and the goals of your machine learning task.
Common Errors and How to Handle
Data Preprocessing
- Inconsistent preprocessing: Applying different preprocessing steps or parameters to the training and test datasets can lead to inconsistency and unexpected results. Ensure consistency in preprocessing between training and testing phases.
Adding Dropout Layers
- Excessive use of dropout: Setting dropout rates too high may lead to underfitting, as the model may struggle to learn useful patterns. Fine-tune the dropout rate based on cross-validation results.
Image Data Augmentation
- Incorrect augmentation parameters: Setting overly aggressive augmentation parameters may result in distorted or unrealistic augmented images. Carefully choose augmentation parameters and visually inspect augmented data.
Fine-Tuning a Pretrained Model
- Incompatibility with target task: Choosing a pre-trained model that is not well-suited for the target task may result in suboptimal performance. Ensure the pre-trained model’s architecture aligns with the characteristics of the target task.
L2 Regularization
- Incorrect regularization strength: Choosing an inappropriate regularization strength (lambda) may lead to either insufficient regularization (overfitting) or excessive regularization (underfitting). Experiment with different values to find an optimal balance.
Conclusion
In conclusion, building accurate machine learning models requires a lot of experimentation and patience. If your TensorFlow model’s accuracy is not increasing, you should consider the factors we’ve discussed in this post. By addressing issues like insufficient data, poor data quality, inappropriate model architecture, and hyperparameters, and using techniques like transfer learning, regularization, dropout, and data augmentation, you can improve your TensorFlow model’s accuracy and build better machine learning models.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.