How to Import Custom Modules in Google Colab
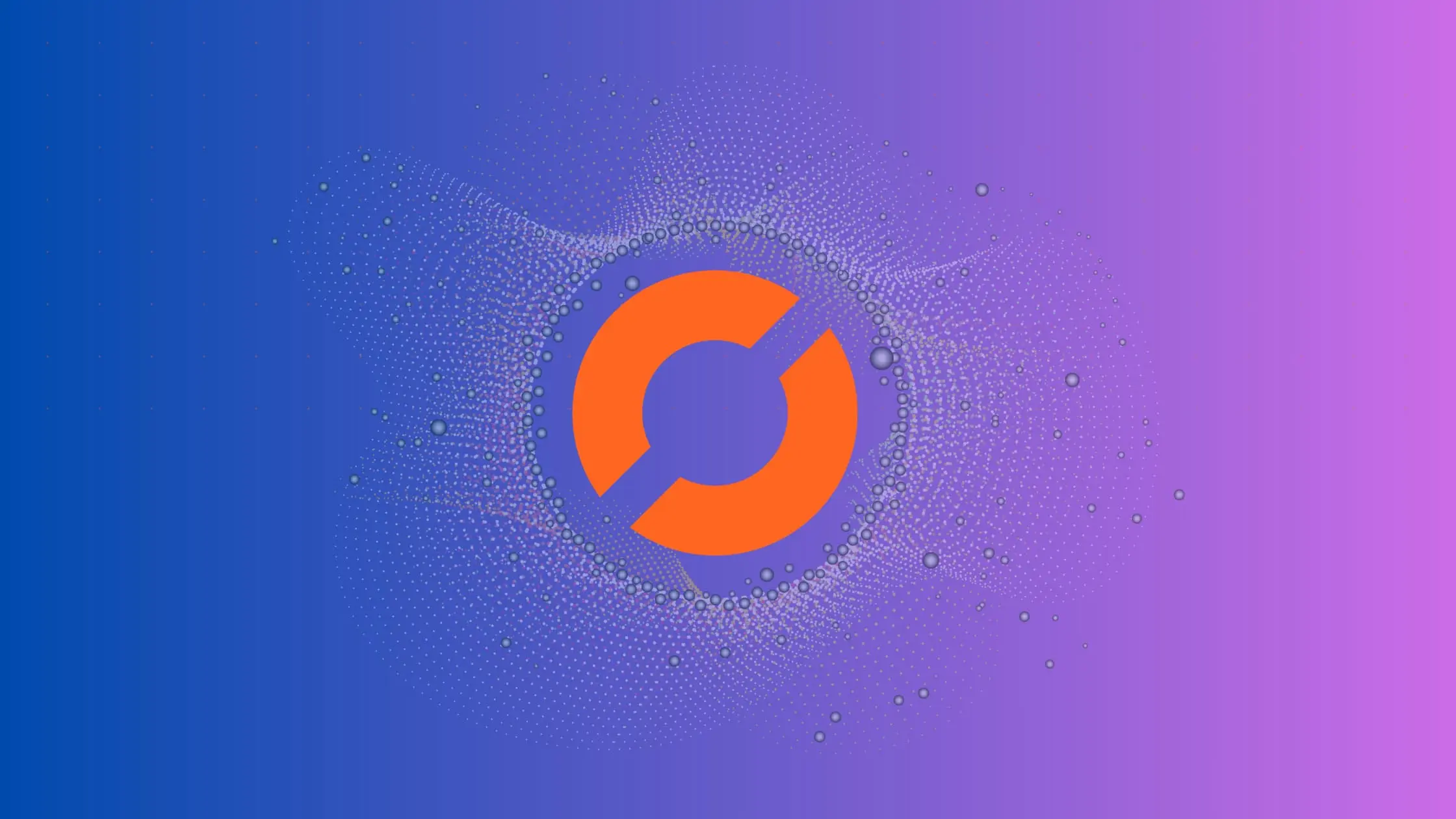
Google Colab is a popular online platform for running data science and machine learning experiments. It provides a powerful and flexible environment for running Python code, including support for popular libraries like TensorFlow, Keras, and PyTorch. However, one common problem that many users face when working with Colab is importing custom modules. In this post, we will explore some techniques for importing custom modules in Google Colab.
Table of Contents
- Introduction
- Why Importing Custom Modules Can Be Tricky in Colab
- Techniques for Importing Custom Modules in Colab
- Conclusion
Why Importing Custom Modules Can Be Tricky in Colab
The reason why importing custom modules can be difficult in Colab is because of the way that Colab is set up. When you launch a new Colab notebook, you are given access to a virtual machine (VM) that has a fresh installation of Python. This means that any Python packages or custom modules that you have installed on your local machine will not be available in Colab by default.
Another issue is that Colab notebooks are ephemeral. This means that the VM that your notebook is running on can be destroyed at any time, which means that any changes that you make to the VM (such as installing custom modules) will be lost when the VM is destroyed. This can make it difficult to maintain a consistent environment across multiple Colab sessions.
Techniques for Importing Custom Modules in Colab
Module example:
# my_module.py
def my_function():
print("Hello from my_function in my_module!")
Technique 1: Uploading the Module to Colab
The easiest way to import a custom module in Colab is to simply upload the module file to Colab. You can do this by clicking on the “Files” button on the left-hand side of the Colab interface, and then clicking on the “Upload” button. Once you have uploaded the module file, you can import it in your Colab notebook using a standard Python import statement.
from my_module import my_function
This technique works well for small modules that do not have many dependencies. However, it can become cumbersome if you have many modules or if your modules have many dependencies.
try:
from my_module import my_function
except ImportError:
print("Module not found. Make sure you uploaded the correct file.")
Technique 2: Cloning a GitHub Repository
Another technique for importing custom modules in Colab is to clone a GitHub repository that contains your module. This has the advantage of making it easy to maintain multiple modules across multiple Colab sessions. To clone a GitHub repository in Colab, you can use the following command:
!git clone https://github.com/username/repo.git
Once you have cloned the repository, you can import your module in your Colab notebook using a standard Python import statement.
from repo.my_module import my_function
This technique works well for larger projects that have many modules and dependencies. However, it does require some setup time to get your module into a GitHub repository.
import os
try:
!git clone https://github.com/username/repo.git
from repo.my_module import my_function
except Exception as e:
print(f"Error: {e}. Check if the repository exists and the module is in the correct location.")
Technique 3: Using Google Drive
Another technique for importing custom modules in Colab is to use Google Drive. Google Drive provides a way to store and share files across multiple devices and platforms, including Colab. To use Google Drive to import your custom module, you can follow these steps:
- Upload your module file to Google Drive.
- Mount Google Drive in your Colab notebook using the following command:
from google.colab import drive
drive.mount('/content/drive')
- Import your module using a standard Python import statement, but with the path to your module file on Google Drive.
from my_module import my_function
This technique works well for modules that are too large to upload to Colab directly, and for modules that you want to share across multiple Colab notebooks.
Leveraging Google Drive requires additional steps. Handle errors related to mounting the drive and importing the module.
from google.colab import drive
try:
drive.mount('/content/drive')
from my_module import my_function
except ImportError:
print("Module not found. Ensure the correct path to the module file on Google Drive.")
except Exception as e:
print(f"Error: {e}. Check if Google Drive is properly mounted.")
Conclusion
Importing custom modules in Google Colab can be tricky, but there are several techniques that you can use to make the process easier. By uploading your module to Colab, cloning a GitHub repository, or using Google Drive, you can import your custom modules in Colab and take advantage of the powerful data science and machine learning capabilities that Colab provides.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.