How to Handle the pandas ValueError could not convert string to float
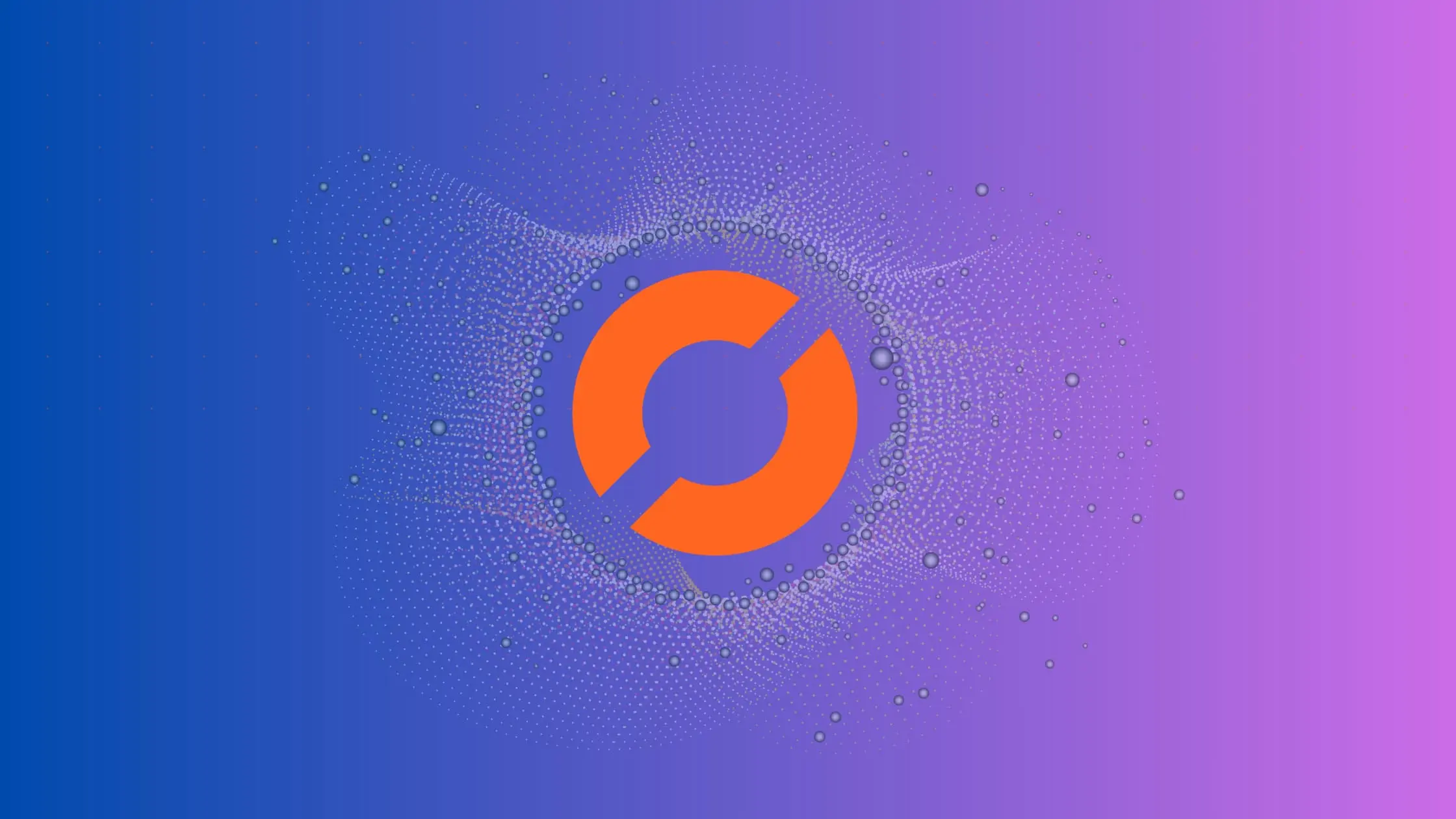
In this article, we will discuss what causes the pandas ValueError: could not convert string to float error and how to handle it.
What Causes the pandas ValueError: could not convert string to float Error?
The pandas ValueError occurs when you use the float()
function to convert a string to a float, but the string contains characters that cannot be interpreted as a float. For example, if the string includes commas or special characters, it can’t be directly converted to a float.
How to Handle the pandas ValueError: Handling the pandas ValueError: Could Not Convert String to Float Error
Dealing with this error is a common task in data preprocessing. Below, we’ll discuss a few approaches to handle it using relevant examples
1. Using the replace() function
One way to address the pandas ValueError is by removing problematic characters from the string. Let’s consider an example where we have a DataFrame with strings that contain commas:
import pandas as pd
# Create a DataFrame with strings containing commas
df = pd.DataFrame({'values': ['1,234', '56,78', '9,100', '3.14']})
# Use the `replace()` function to remove commas
df['values'] = df['values'].replace(',', '', regex=True)
# Convert the column to floats
df['values'] = df['values'].astype(float)
# Print the DataFrame
print(df)
In this example, we create a DataFrame with string values containing commas. We utilize the replace() function with a regular expression to remove the commas, making the strings convertible to floats. Finally, we convert the column to floats using the astype()
function.
2. Using the to_numeric() function
Another approach is to employ the to_numeric() function, which can handle a variety of non-numeric characters, including special symbols. Let’s see it in action:
import pandas as pd
# Create a DataFrame with strings containing special characters
df = pd.DataFrame({'values': ['42@', '$78', '12%', '3.14']})
# Use the `to_numeric()` function to convert the column to floats
df['values'] = pd.to_numeric(df['values'], errors='coerce')
# Print the DataFrame
print(df)
In this example, we have a DataFrame with string values that include special characters. By using the to_numeric() function with the errors=‘coerce’ parameter, we convert the column to floats, and non-numeric values are replaced with NaN.
3. Using the apply() function
A more flexible approach is to utilize the apply() function. This allows you to define custom conversion logic. Suppose you have a DataFrame with strings containing a mix of numbers and special characters:
import pandas as pd
# Create a DataFrame with strings containing a mix of numbers and special characters
df = pd.DataFrame({'values': ['123', '@456', '78$', '3.14']})
# Use the `apply()` function to convert the column to floats
df['values'] = df['values'].apply(lambda x: float(''.join(filter(str.isdigit, x))) if not x.isnumeric() else float(x))
# Print the DataFrame
print(df)
In this example, we’ve employed the apply() function along with a custom lambda function. It checks each value, extracts the numeric parts, and converts them to floats, handling various special characters.
Conclusion
Handling the pandas ValueError: could not convert string to float error is a common challenge in data processing. By using the replace(), to_numeric(), or apply() functions, you can effectively manage strings with non-numeric characters. Hopefully, this article has helped you to better understand how to handle this error and make your data science work a little bit easier.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.