How to Handle Pandas KeyError Value Not in Index
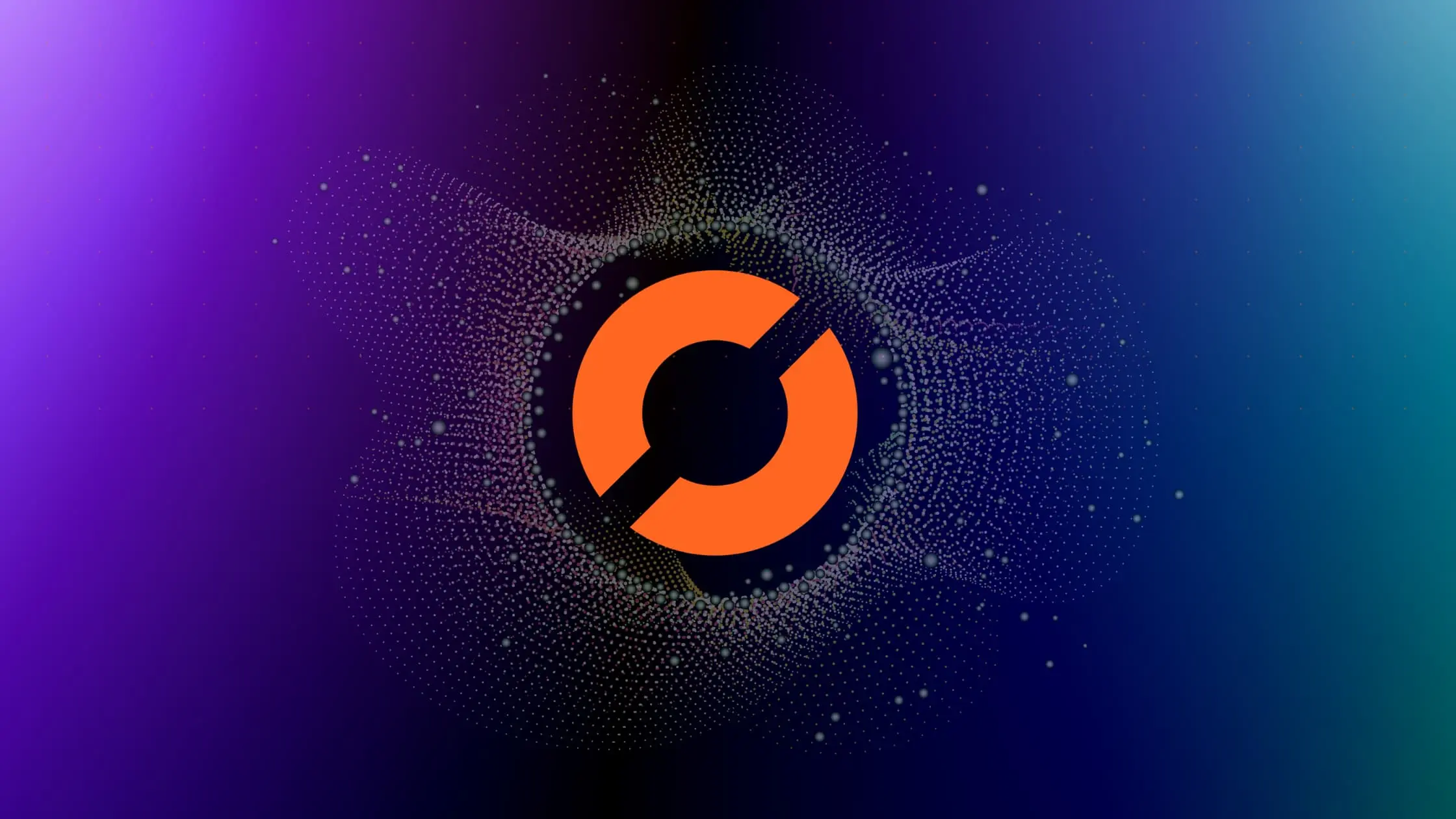
Understanding the Pandas KeyError
The Pandas KeyError occurs when a key (e.g., a column or index label) is not found in a DataFrame or Series. This error can occur for several reasons, such as:
- The key does not exist in the DataFrame or Series.
- The key is misspelled or capitalized differently from the actual key.
- The key has a different data type than expected.
Let’s take a look at an example. Suppose we have a DataFrame called df
with columns ‘A’, ‘B’, and ‘C’:
import pandas as pd
data = {'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]}
df = pd.DataFrame(data)
If we try to access the value in the ‘D’ column, we will get a Pandas KeyError:
df['D']
Output:
KeyError: 'D'
Handling Pandas KeyError
Now that we understand the causes of the Pandas KeyError, let’s explore some solutions to handle it.
1. Check the Spelling and Capitalization
One common reason for the Pandas KeyError is misspelling or capitalization. Make sure that the key is spelled correctly and capitalized in the same way as in the DataFrame or Series. You can check the keys of a DataFrame or Series using the keys()
method:
df.keys()
Output:
Index(['A', 'B', 'C'], dtype='object')
2. Check the Data Type
Another reason for the Pandas KeyError is a different data type of the key than expected. For example, if the index of a DataFrame is numeric, but you try to access it with a string, you will get a KeyError. You can check the data type of the index using the dtype
attribute:
df.index.dtype
Output:
dtype('int64')
3. Use the loc and iloc Accessors
If you want to access a specific value in a DataFrame or Series, you can use the loc
and iloc
accessors. The loc
accessor is used to access values by label, while the iloc
accessor is used to access values by integer position. Let’s see an example:
# Access the value in row 0, column 'A'
df.loc[0, 'A']
# Access the value in row 1, column 2
df.iloc[1, 2]
4. Use the in Operator
If you want to check if a key is present in a DataFrame or Series, you can use the in
operator. For example:
# Check if 'D' is in the columns of the DataFrame
'D' in df.columns
# Check if 2 is in the index of the DataFrame
2 in df.index
5. Use the reindex Method
If you want to add a new key to a DataFrame or Series, you can use the reindex
method. This method creates a new DataFrame or Series with the specified index and fills the missing values with NaN:
# Add a new column 'D' to the DataFrame
df = df.reindex(columns=['A', 'B', 'C', 'D'])
# Add a new row with index 3 to the DataFrame
df = df.reindex(index=[0, 1, 2, 3])
Conclusion
The Pandas KeyError can be frustrating, but it is also a valuable error message that can help you debug your code. By understanding the causes of the error and using the solutions we have provided, you can handle the Pandas KeyError and make your code more robust.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.