How to Get the Number of Days Between Two Dates Using Pandas
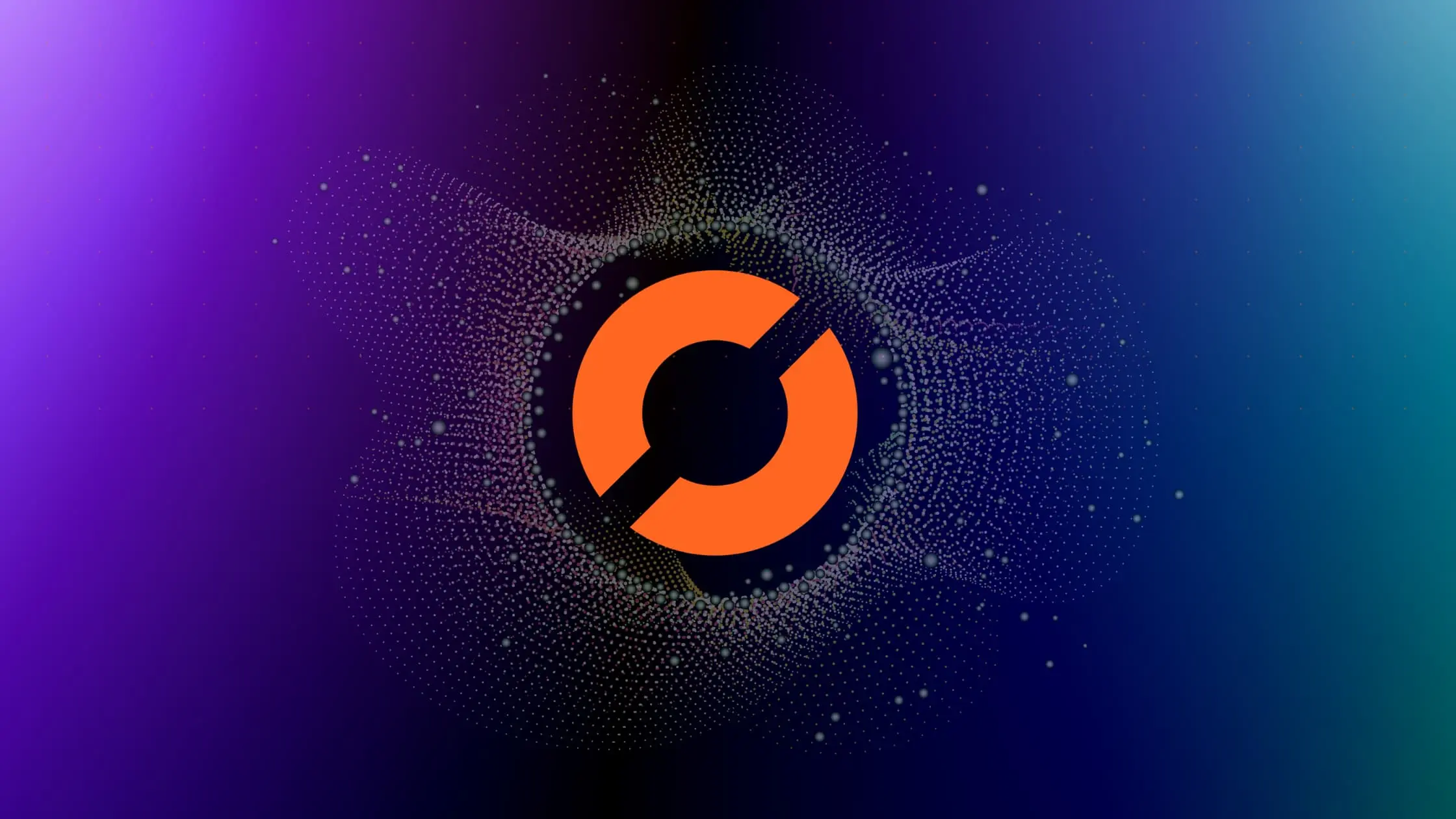
As a data scientist or software engineer, you will often come across situations where you need to work with dates and times. One common task is to calculate the number of days between two dates. In this blog post, we will explore how to get the number of days between two dates using the popular Python library, Pandas.
What is Pandas?
Pandas is a Python library that provides high-performance data manipulation and analysis tools. It is built on top of the NumPy library and provides data structures for efficiently storing and manipulating large datasets. Pandas is widely used in data science and machine learning for data cleaning, exploration, and analysis.
Getting Started
To get started, you will need to have Pandas installed on your system. You can install it using the following command:
pip install pandas
Once you have installed Pandas, you can import it into your Python script using the following command:
import pandas as pd
Calculating the Number of Days Between Two Dates
To calculate the number of days between two dates using Pandas, we can use the datetime
module to create two datetime
objects representing the two dates. We can then subtract one from the other to get a timedelta
object representing the difference between the two dates. Finally, we can extract the number of days from the timedelta
object.
Here’s an example:
import pandas as pd
from datetime import datetime
# Create two datetime objects
date1 = datetime(2022, 1, 1)
date2 = datetime(2022, 1, 15)
# Calculate the number of days between the two dates
delta = date2 - date1
num_days = delta.days
print(num_days) # Output: 14
Output:
14
In the example above, we create two datetime
objects representing January 1, 2022, and January 15, 2022. We then subtract date1
from date2
to get a timedelta
object representing the difference between the two dates. Finally, we extract the number of days from the timedelta
object using the days
attribute and store it in the num_days
variable.
Using Pandas to Calculate the Number of Days Between Two Dates
While the above approach works fine, it can be cumbersome if you have to work with a large number of dates. Fortunately, Pandas provides a more concise and efficient way to calculate the number of days between two dates.
To use Pandas, we first need to create a DataFrame with the two dates as columns. We can then subtract one column from the other to get a new column with the time delta between the two dates. Finally, we can extract the number of days from the time delta column.
Here’s an example:
import pandas as pd
# Create a DataFrame with two dates
df = pd.DataFrame({
'date1': pd.to_datetime(['2022-01-01', '2022-01-15']),
'date2': pd.to_datetime(['2022-01-15', '2022-01-30'])
})
# Calculate the number of days between the two dates
df['num_days'] = (df['date2'] - df['date1']).dt.days
print(df)
Output:
date1 date2 num_days
0 2022-01-01 2022-01-15 14
1 2022-01-15 2022-01-30 15
In the example above, we create a DataFrame with two columns, date1
and date2
, representing the two dates. We then calculate the time delta between the two dates by subtracting date1
from date2
and storing the result in a new column called num_days
. Finally, we print the DataFrame to the console.
Conclusion
Calculating the number of days between two dates is a common task in data science and machine learning. In this blog post, we have explored two approaches for doing this using Pandas. While the first approach using the datetime
module is straightforward, the second approach using Pandas is more concise and efficient, especially when working with large datasets.
Whether you are a data scientist or software engineer, Pandas is an essential library to have in your toolkit. Its powerful data manipulation and analysis tools make it a must-have for anyone working with data. We hope this blog post has been helpful in showing you how to use Pandas to calculate the number of days between two dates.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.