How to Get the Index of a Row in a Pandas DataFrame as an Integer
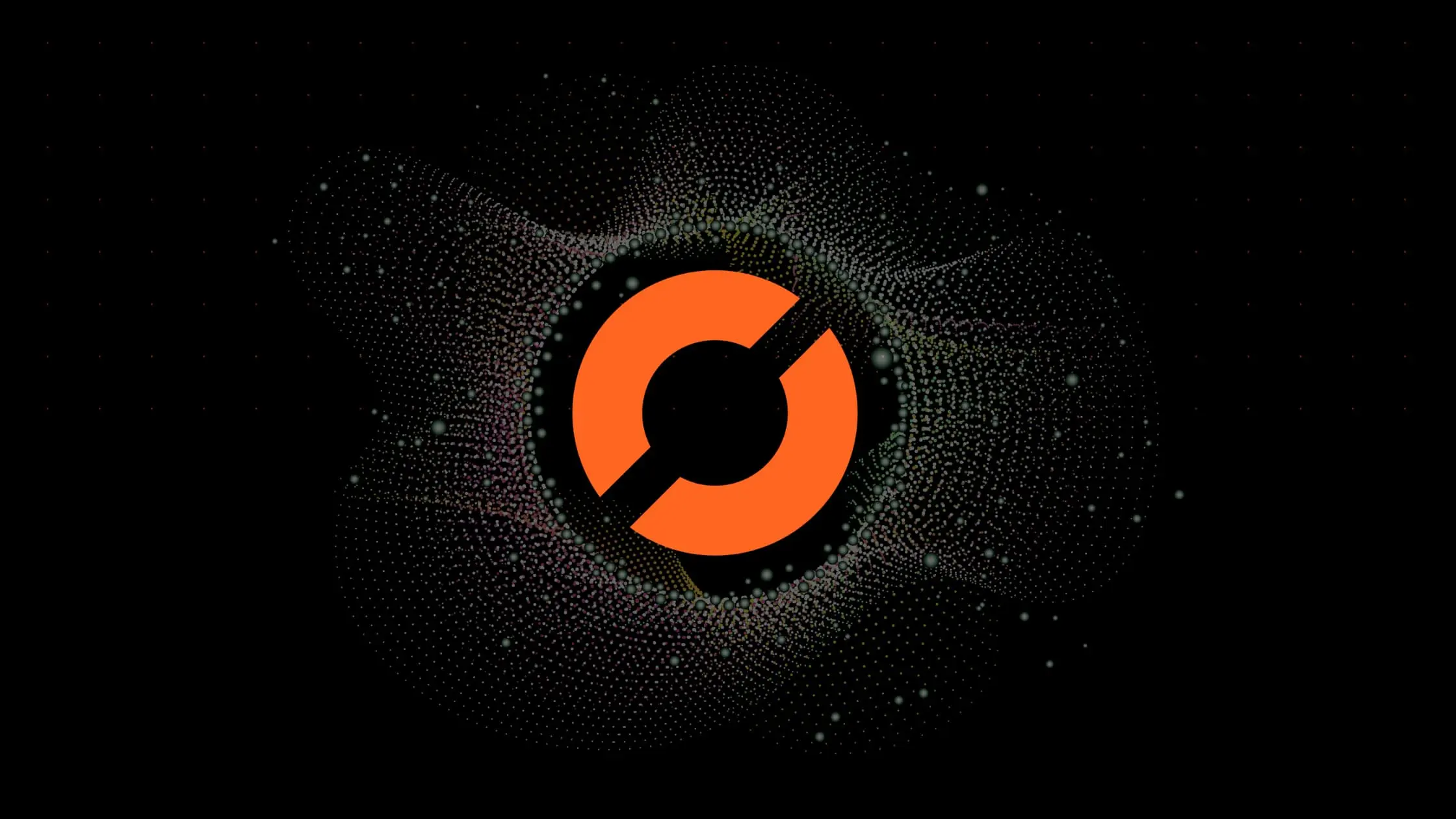
As a data scientist or software engineer, you may often work with large datasets in your projects. One of the most popular tools for data manipulation and analysis is the Pandas library in Python. Pandas provides an easy-to-use interface for handling tabular data, but sometimes you may need to retrieve the index of a specific row in a DataFrame as an integer. In this article, we will explore different methods to obtain the index of a row in a Pandas DataFrame as an integer.
What is a Pandas DataFrame?
A Pandas DataFrame is a two-dimensional labeled data structure with columns of potentially different types. It is similar to a spreadsheet or SQL table, where each row represents an observation and each column represents a variable. Pandas allows you to perform various operations such as filtering, sorting, grouping, and merging on the DataFrame.
How to Get the Index of a Row in a Pandas DataFrame as an Integer?
There are several ways to retrieve the index of a row in a Pandas DataFrame as an integer. Let’s explore some of them.
Using the iloc method
The iloc
method allows you to access rows and columns of a Pandas DataFrame by integer position. You can pass a single integer or a list of integers to the iloc
method to retrieve the corresponding row(s). To get the index of a row as an integer, you can call the index
attribute on the resulting DataFrame slice.
import pandas as pd
# create a sample DataFrame
data = {
'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Eva'],
'Math': [95, 88, 76, 92, 89],
'Science': [78, 90, 82, 96, 88],
'History': [85, 79, 91, 88, 94]
}
df = pd.DataFrame(data)
print(df)
Output:
Name Math Science History
0 Alice 95 78 85
1 Bob 88 90 79
2 Charlie 76 82 91
3 David 92 96 88
4 Eva 89 88 94
For example, if you want to obtain the index of the row containing information about David
, you can do the following:
row_index = df.index[df['Name'] == 'David'].tolist()
print(row)
Output:
3
In the above example:
df['Name'] == 'David'
filters the DataFrame to find the row(s) where the ‘Name’ column matchesDavid
..tolist()
converts the resulting index into a list. In this case, it will be a list containing a single integer value, which is the index of the row containingDavid
.
Using the .index.get_loc()
method:
You can use the .index.get_loc()
method to directly retrieve the integer location of a specific index label. This method allows you to obtain the row index based on the value in the Name
column.
row_index = df.index.get_loc(df[df['Name'] == 'David'].index[0])
print(row_index)
Output:
3
In this code, we first find the index label of the row with the Name
column equal to David
, and then we use .index.get_loc()
to get its integer location.
Using the .loc
method:
The .loc
method can be used to access rows by their index labels, and it can return the integer location of a specific row as well. Here’s how you can do it:
row_index = df.index.get_loc(df.loc[df['Name'] == 'David'].index[0])
Output
3
This approach combines both .loc
and .index.get_loc()
to achieve the same result.
Using the .query()
method:
The .query()
method allows you to filter rows based on specific criteria, making it convenient for obtaining the index as an integer.
row_index = df.query("Name == 'David'").index[0]
Output:
3
This code uses the .query()
method to filter rows where the Name
column is equal to David
and then retrieves the index as an integer.
Conclusion
Retrieving the index of a row in a Pandas DataFrame as an integer is a common task in data analysis and manipulation. In this article, we explored different methods to achieve this, including using the iloc
, get_loc
, loc
, query
methods, and the index
attribute. Each method has its own advantages and disadvantages, depending on the specific use case. By understanding these methods, you can improve your productivity and efficiency when working with large datasets in your projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.