How to Get the First Row of Each Group in a Pandas DataFrame
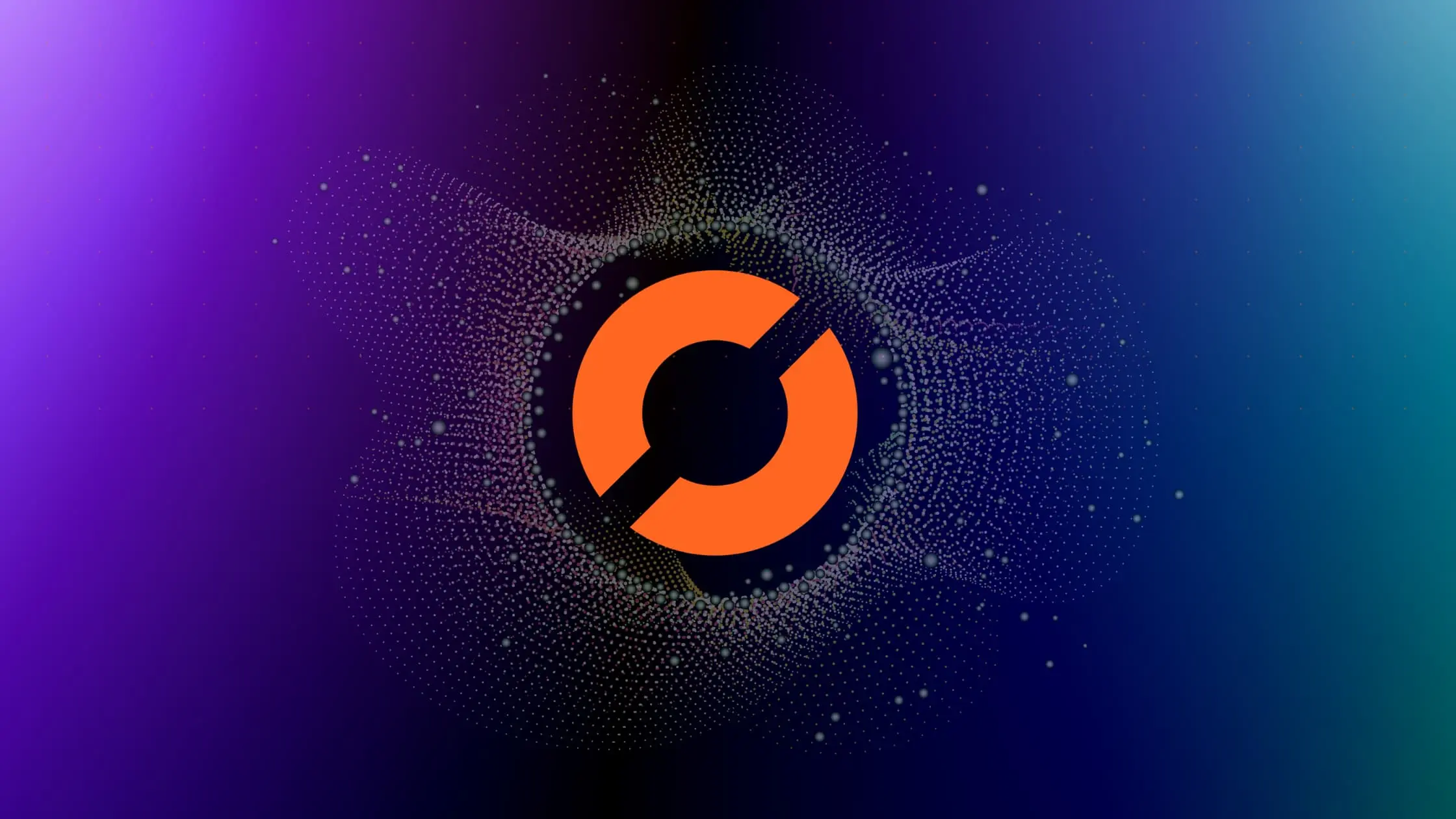
As a data scientist or software engineer, you may often find yourself working with large datasets consisting of multiple groups. In such cases, it can be useful to extract the first row of each group to get a better understanding of the data. In this article, we will explore how to get the first row of each group in a Pandas DataFrame using Python programming language.
Table of Contents
- What is Pandas?
- What is a Groupby Operation?
- How to Get the First Row of Each Group in a Pandas DataFrame?
- Common Errors and How to Handle Them
- Conclusion
What is Pandas?
Pandas is a popular data manipulation library in Python which is widely used in data analysis and data science projects. It provides powerful data structures like DataFrame and Series which allow you to manipulate and analyze data in an easy and intuitive way.
What is a Groupby Operation?
In Pandas, a groupby operation involves grouping data based on a specific column or set of columns and then performing some aggregate operation on each group. For example, you may want to group a dataset based on the ‘category’ column and then perform a mean calculation on the ‘price’ column for each group.
How to Get the First Row of Each Group in a Pandas DataFrame?
Method 1: Using groupby
vs first
:
To get the first row of each group in a Pandas DataFrame, we can use the groupby()
method followed by the first()
method. The groupby()
method groups the data based on a specific column or set of columns, and the first()
method returns the first row of each group.
import pandas as pd
# create a sample dataframe
data = {'category': ['A', 'A', 'B', 'B', 'C', 'C'], 'value': [1, 2, 3, 4, 5, 6]}
df = pd.DataFrame(data)
# group the data by 'category' and get the first row of each group
first_rows = df.groupby('category').first()
print(first_rows)
The output of the above code will be:
value
category
A 1
B 3
C 5
As you can see, the groupby()
method has grouped the data based on the ‘category’ column, and the first()
method has returned the first row of each group.
Method 2: groupby().apply(lambda x: x.iloc[0])
:
Here, we use the apply
function with a lambda function to extract the first row of each group using integer-location based indexing (iloc
). This method provides more flexibility as you can customize the extraction logic within the lambda function.
first_rows = df.groupby('category').apply(lambda x: x.iloc[0]).reset_index(drop=True)
print(first_rows)
Output:
category value
0 A 1
1 B 3
2 C 5
Method 3: groupby().head(1)
:
The head
function retrieves the first n rows of each group, and by specifying 1
, we get only the first row. This method is efficient and concise, especially when dealing with large datasets.
first_rows = df.groupby('category').head(1).reset_index(drop=True)
print(first_rows)
Output:
category value
0 A 1
1 B 3
2 C 5
Common Errors and How to Handle Them
Error 1: Grouping Column Not Present:
# Example
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
result = df.groupby('C').first() # 'C' column does not exist
# Solution
# Ensure the grouping column exists in the DataFrame.
Error 2: Applying Functions with Missing Values:
# Example
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
result = df.groupby('A').apply(lambda x: x.iloc[1]) # IndexError for the second group
# Solution
# Check for missing values or adjust the function to handle them gracefully.
Error 3: Non-Numeric Data Types in the Grouping Column:
# Example
df = pd.DataFrame({'A': ['apple', 'banana', 'apple'], 'B': [4, 5, 6]})
result = df.groupby('A').first() # TypeError due to non-numeric grouping column
# Solution
# Ensure the grouping column has a numeric data type or use a suitable conversion.
Conclusion
In this guide, we explored different methods to extract the first row of each group in a Pandas DataFrame, comparing their pros and cons. We also addressed common errors that may occur during implementation, providing examples and solutions. Choose the method that best suits your needs and be mindful of potential pitfalls when working with grouped data in Pandas. This can be useful in various data analysis and data science projects where you need to extract specific information from large datasets.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.