How to Get the First Row (occurence) of a Pandas DataFrame in Python Using a Specific Column Value
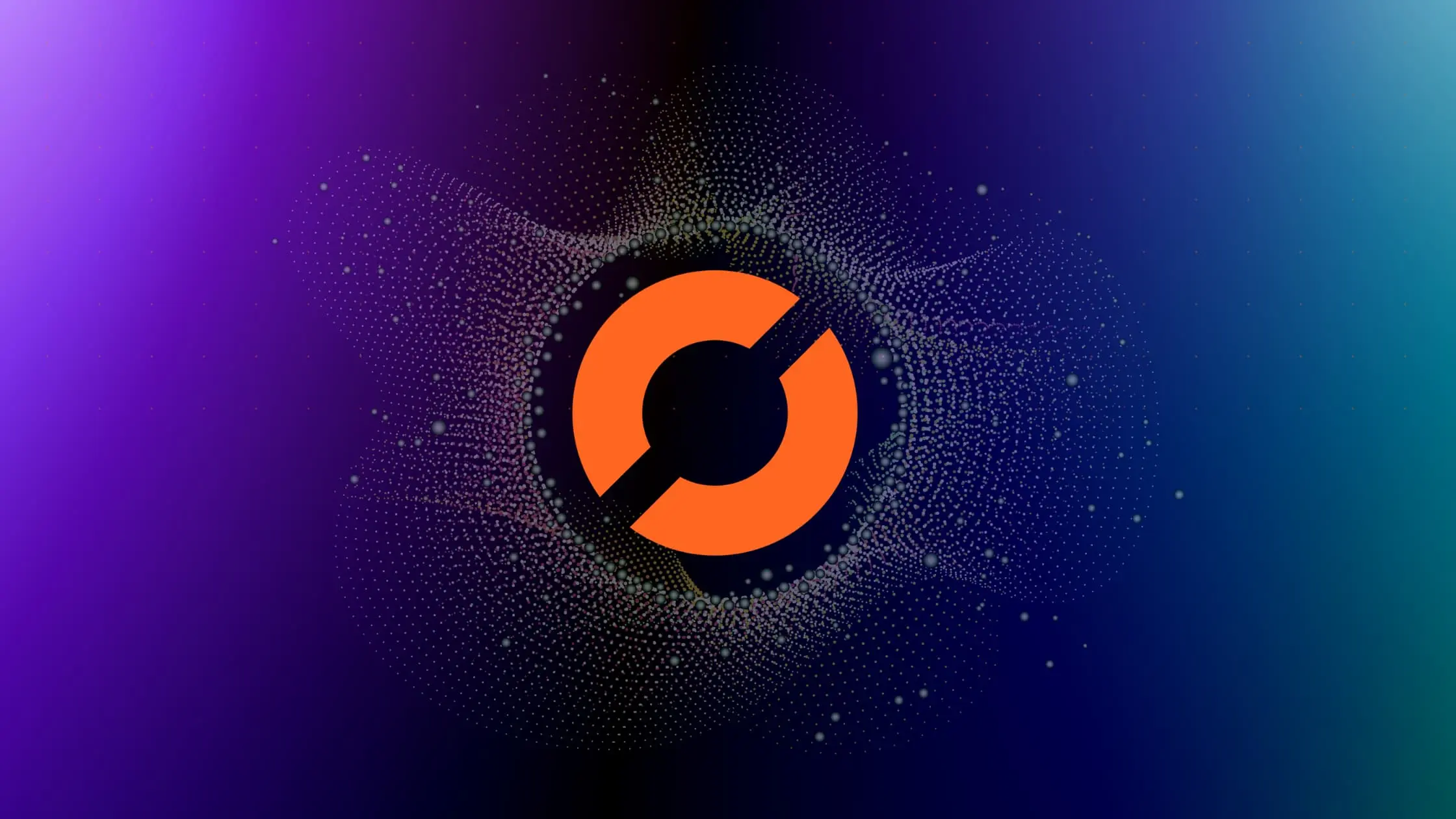
Table of Contents
- Method 1: Using the .loc Function
- Method 2: Using the .query Function
- Method 3: Using the .head Function
- Pros and Cons
- Conclusion
In this article, we will explore some efficient and effective ways to get the first row of a Pandas dataframe based on criteria, without iterating over the entire dataframe.
Method 1: Using the .loc Function
One of the simplest and most straightforward ways to extract the first row of a dataframe based on a specific condition is to use the .loc
function in Pandas. The .loc
function allows you to select rows and columns of a dataframe based on labels or conditions.
To use the .loc
function to extract the first row of a dataframe based on a condition, you can use the following syntax:
df.loc[df['column_name'] == 'desired_value'].iloc[0]
Here, df
is the name of your dataframe, column_name
is the name of the column you want to filter on, and desired_value
is the value you want to filter for. The .loc
function returns a subset of the dataframe that satisfies the condition, and the .iloc
function is used to select the first row of the resulting subset.
Let’s take a look at an example to see how this works in practice:
import pandas as pd
# create a sample dataframe
data = {'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'city': ['New York', 'Los Angeles', 'Chicago', 'Houston']}
df = pd.DataFrame(data)
# extract the first row where the age is greater than 30
first_row = df.loc[df['age'] > 30].iloc[0]
print(first_row)
Output:
name Charlie
age 35
city Chicago
Name: 2, dtype: object
In this example, we created a sample dataframe with three columns: name
, age
, and city
. We then used the .loc
function to extract the first row where the age
was greater than 30, and the .iloc
function to select the first row of the resulting subset. The output shows that the first row where the age
is greater than 30 is Charlie
, who is 35 years old and lives in Chicago.
Method 2: Using the .query Function
Another way to extract the first row of a dataframe based on a condition is to use the .query
function in Pandas. The .query
function allows you to filter a dataframe based on a string expression.
To use the .query
function to extract the first row of a dataframe based on a condition, you can use the following syntax:
df.query('column_name == desired_value').iloc[0]
Here, df
is the name of your dataframe, column_name
is the name of the column you want to filter on, and desired_value
is the value you want to filter for. The .query
function returns a subset of the dataframe that satisfies the condition, and the .iloc
function is used to select the first row of the resulting subset.
Let’s take a look at an example to see how this works in practice:
import pandas as pd
# create a sample dataframe
data = {'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'city': ['New York', 'Los Angeles', 'Chicago', 'Houston']}
df = pd.DataFrame(data)
# extract the first row where the age is greater than 30
first_row = df.query('age > 30').iloc[0]
print(first_row)
Output:
name Charlie
age 35
city Chicago
Name: 2, dtype: object
In this example, we created a sample dataframe with three columns: name
, age
, and city
. We then used the .query
function to extract the first row where the age
was greater than 30, and the .iloc
function to select the first row of the resulting subset. The output shows that the first row where the age
is greater than 30 is Charlie
, who is 35 years old and lives in Chicago.
Method 3: Using the .head Function
If you know that the first row of a dataframe that meets a certain condition is located at the beginning of the dataframe, you can use the .head
function in Pandas to extract the first few rows of the dataframe that meet the condition.
To use the .head
function to extract the first row of a dataframe based on a condition, you can use the following syntax:
df[df['column_name'] == 'desired_value'].head(1)
Here, df
is the name of your dataframe, column_name
is the name of the column you want to filter on, and desired_value
is the value you want to filter for. The .head
function returns the first n
rows of the resulting subset, where n
is the number specified in the function call.
Let’s take a look at an example to see how this works in practice:
import pandas as pd
# create a sample dataframe
data = {'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'city': ['New York', 'Los Angeles', 'Chicago', 'Houston']}
df = pd.DataFrame(data)
# extract the first row where the age is greater than 30
first_row = df[df['age'] > 30].head(1)
print(first_row)
Output:
name age city
2 Charlie 35 Chicago
In this example, we created a sample dataframe with three columns: name
, age
, and city
. We then used the .head
function to extract the first row where the age
was greater than 30, and the output shows that the first row where the age
is greater than 30 is Charlie
, who is 35 years old and lives in Chicago.
Pros and Cons
Method 1 (.loc):
- Pros: readable, supports complex filtering conditions, performant (small-medium data).
- Cons: Less concise, extra indexing layer.
Method 2 (.query):
- Pros: Concise, flexible for simple comparisons.
- Cons: Less intuitive, potentially slower (large data).
Method 3 (.head):
- Pros: Most concise, efficient (small data).
- Cons: Limited, inflexible, potentially redundant.
You can choose based on: Data size, code clarity, and complexity of filtering.
Conclusion
In this article, we have explored some efficient and effective ways to get the first row of a Pandas dataframe based on criteria, without iterating over the entire dataframe. By using the .loc
function, the .query
function, and the .head
function, you can quickly and easily extract the first row of a dataframe that meets a certain condition, even when working with large datasets.
Remember that the method you choose will depend on the specifics of your use case, and it is always important to carefully test and validate your code to ensure that it is working as expected. With these techniques in your toolbox, you can streamline your Pandas workflows and become a more efficient and effective data scientist or software engineer.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.