How to Get Column Name Which Contains a Specific Value at Any Rows in Python Pandas
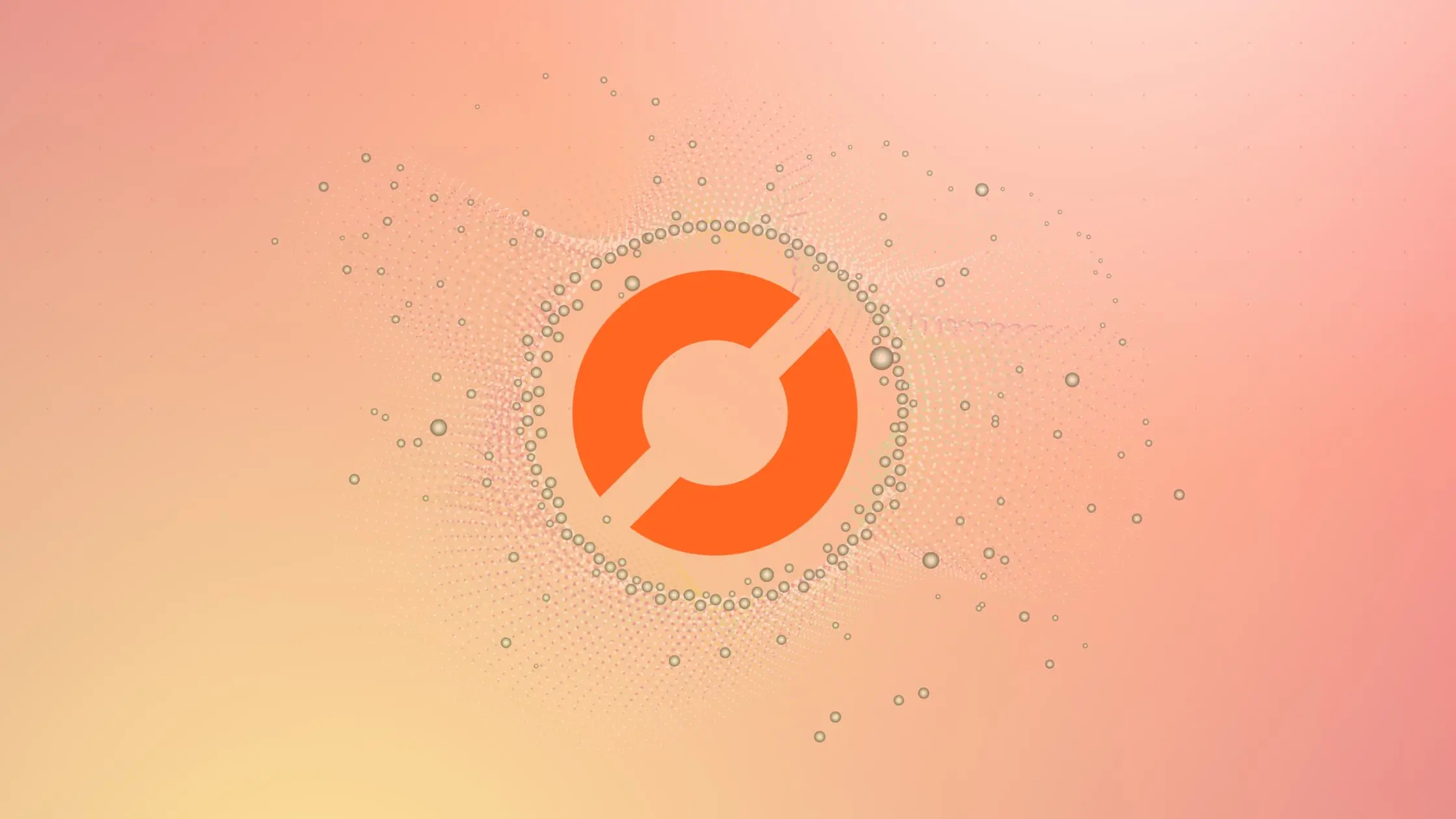
As a data scientist or software engineer working with data, you may find yourself needing to identify the column name that contains a specific value at any row in a Pandas DataFrame. This can be a common task when performing data cleaning, data wrangling, or data analysis. In this article, we will explore different ways to achieve this task using Python Pandas.
Table of Contents
Understanding the Problem
Before diving into the solution, it is important to understand the problem we are trying to solve. Let’s consider the following example DataFrame:
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
})
Suppose we want to find the column name that contains the value 5
at any row in the DataFrame. In this case, the expected output is 'B'
.
Solution
Using df.isin() Function
This method involves the use of the isin()
function combined with boolean indexing.
df.isin([5])
: Creates a boolean DataFrame where each cell isTrue
if it matches the specified value (5 in this case) andFalse
otherwise..any()
: This is applied to the resulting boolean DataFrame, aggregating each column to a single boolean value (True
if any cell in the column matches the value,False
otherwise).df.columns[...]
: The boolean Series obtained from the previous step is then used to index the DataFrame’s columns, extracting the names of columns that contain the target value.
# Target value
value = 5
# Extract Column Names
column_names = df.columns[df.isin([value]).any()].tolist()
print(column_names)
Output:
['B']
Pros
Efficiency: This method is highly efficient, especially for large datasets, as it leverages vectorized operations.
Simplicity: The code is concise and easy to read.
Directness: This approach directly targets the columns, reducing intermediate steps.
Cons
- Understanding Vectorization: Requires a basic understanding of Pandas operations and boolean indexing.
Using a Boolean Mask with Pandas
Create a Boolean Mask: First, create a Boolean DataFrame where each cell is True if it matches the target value and
False
otherwise.Aggregate Across Rows: Then, use the
any()
function to aggregate this information across rows. This will give you a Series where each element isTrue
if the corresponding column contains the target value at least once.Extract Column Names: Finally, extract the column names where the value is
True
.
# Target value
value = 5
# Step 1: Create a Boolean Mask
mask = df == value
# Step 2: Aggregate across rows
columns_with_value = mask.any()
# Step 3: Extract Column Names
column_names = columns_with_value[columns_with_value].index.tolist()
print(column_names)
Output:
['B']
Pros
Efficiency: Leveraging vectorized operations makes this method suitable for large DataFrames.
Comprehensiveness: Retrieves all columns containing the specified value.
Readability: The code is clear and easy to follow.
Cons
- Understanding Vectorization: Requires understanding of vectorized operations in Pandas, which might be slightly advanced for beginners.
Using numpy
The numpy
library is known for its efficient numerical operations. By combining numpy
with Pandas, we can achieve a more optimized solution for identifying the column name containing the target value.
- Create a NumPy Array: Convert the Pandas DataFrame into a NumPy array for efficient element-wise operations.
import numpy as np
value = 5
df_array = df.to_numpy()
- Find Indices of Target Value: Use numpy functions to find the indices where the target value occurs in the array.
row_indices, col_indices = np.where(df_array == value)
- Extract Unique Column Names: Extract unique column names corresponding to the identified column indices.
column_names = df.columns[col_indices].unique().tolist()
print(column_names)
Output:
['B']
Pros
Takes advantage of the efficiency of numpy for array operations.
Suitable for large datasets due to optimized underlying operations.
Cons
Requires conversion of the DataFrame to a NumPy array, which may not be desirable in some cases.
Conclusion
This article provided three efficient methods to find all column names in a Pandas DataFrame that contain a specific value. These techniques are essential for data analysts and scientists to effectively manage and analyze their data. Remember to test your code thoroughly and adapt it to fit your specific data scenarios.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.