How to Get Column Index from Column Name in Python Pandas
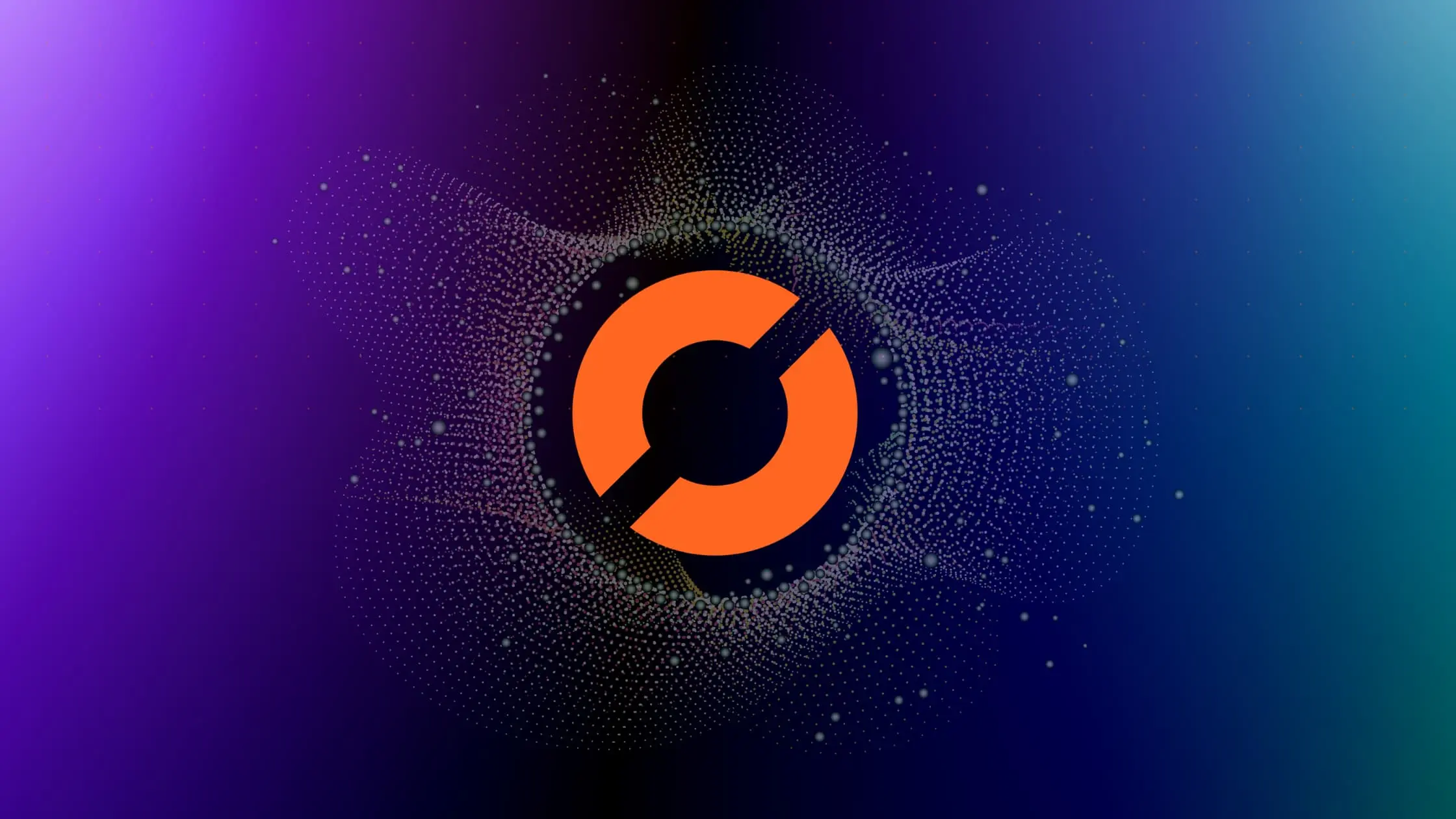
As a data scientist or software engineer, working with data is a crucial part of our daily routine. One of the most popular data analysis libraries in Python is Pandas, which allows us to manipulate and analyze data effectively. In this tutorial, we will explain how to get the column index from a column name in Python Pandas.
What is a Column Index in Pandas?
Before diving into the solution, let’s first understand what a column index is in Pandas. A column index is a numerical representation of the position of a column in a pandas DataFrame. Each column in a DataFrame has a unique index, starting from 0 and incrementing by 1 for each subsequent column.
For example, let’s consider a DataFrame with three columns Name
, Age
, and Gender
. The column index for Name
would be 0, Age
would be 1, and Gender
would be 2.
Name | Age | Gender |
---|---|---|
Alice | 25 | F |
Bob | 30 | M |
John | 20 | M |
Mary | 35 | F |
How to Get Column Index from Column Name
Now that we understand what a column index is, let’s see how we can get the column index from a column name in Python Pandas.
Method 1: Using the .get_loc() method
The easiest way to get the column index from a column name in Pandas is to use the .get_loc()
method. This method returns the integer location of a column in a DataFrame or Series based on its label.
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({
'Name': ['Alice', 'Bob', 'John', 'Mary'],
'Age': [25, 30, 20, 35],
'Gender': ['F', 'M', 'M', 'F']
})
# Get the column index of `Age`
age_index = df.columns.get_loc('Age')
print(age_index) # Output: 1
In the above code, we first create a sample DataFrame with three columns Name
, Age
, and Gender
. We then use the .columns.get_loc()
method to get the index of the Age
column, which is 1. We store this value in the age_index
variable and print it to the console.
Method 2: Using the .index() method
Another way to get the column index from a column name in Pandas is to use the .index()
method. This method returns the index of the first occurrence of a value in a list, which in this case is the index of the column name in the list of column names.
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({
'Name': ['Alice', 'Bob', 'John', 'Mary'],
'Age': [25, 30, 20, 35],
'Gender': ['F', 'M', 'M', 'F']
})
# Get the column index of `Age`
age_index = df.columns.tolist().index('Age')
print(age_index) # Output: 1
In the above code, we first create a sample DataFrame with three columns Name
, Age
, and Gender
. We then use the .columns.tolist().index()
method to get the index of the Age
column, which is 1. We store this value in the age_index
variable and print it to the console.
Method 3: Using a Dictionary
If you have a large DataFrame with many columns and you need to get the index of multiple columns, it may be more efficient to create a dictionary that maps column names to their corresponding indices.
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({
'Name': ['Alice', 'Bob', 'John', 'Mary'],
'Age': [25, 30, 20, 35],
'Gender': ['F', 'M', 'M', 'F']
})
# Create a dictionary to map column names to indices
col_index_map = {col_name: i for i, col_name in enumerate(df.columns)}
# Get the column index of `Age`
age_index = col_index_map['Age']
print(age_index) # Output: 1
In the above code, we first create a sample DataFrame with three columns Name
, Age
, and Gender
. We then create a dictionary col_index_map
that maps each column name to its corresponding index using a dictionary comprehension. Finally, we use the dictionary to get the index of the Age
column, which is 1. We store this value in the age_index
variable and print it to the console.
Conclusion
In this tutorial, we have explained how to get the column index from a column name in Python Pandas. We have shown three different methods to achieve this, each with its own advantages and disadvantages. By using these methods, you can easily get the index of a column in a Pandas DataFrame, which can be useful for various data analysis tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.