How to Get a List from a Pandas Dataframe Column
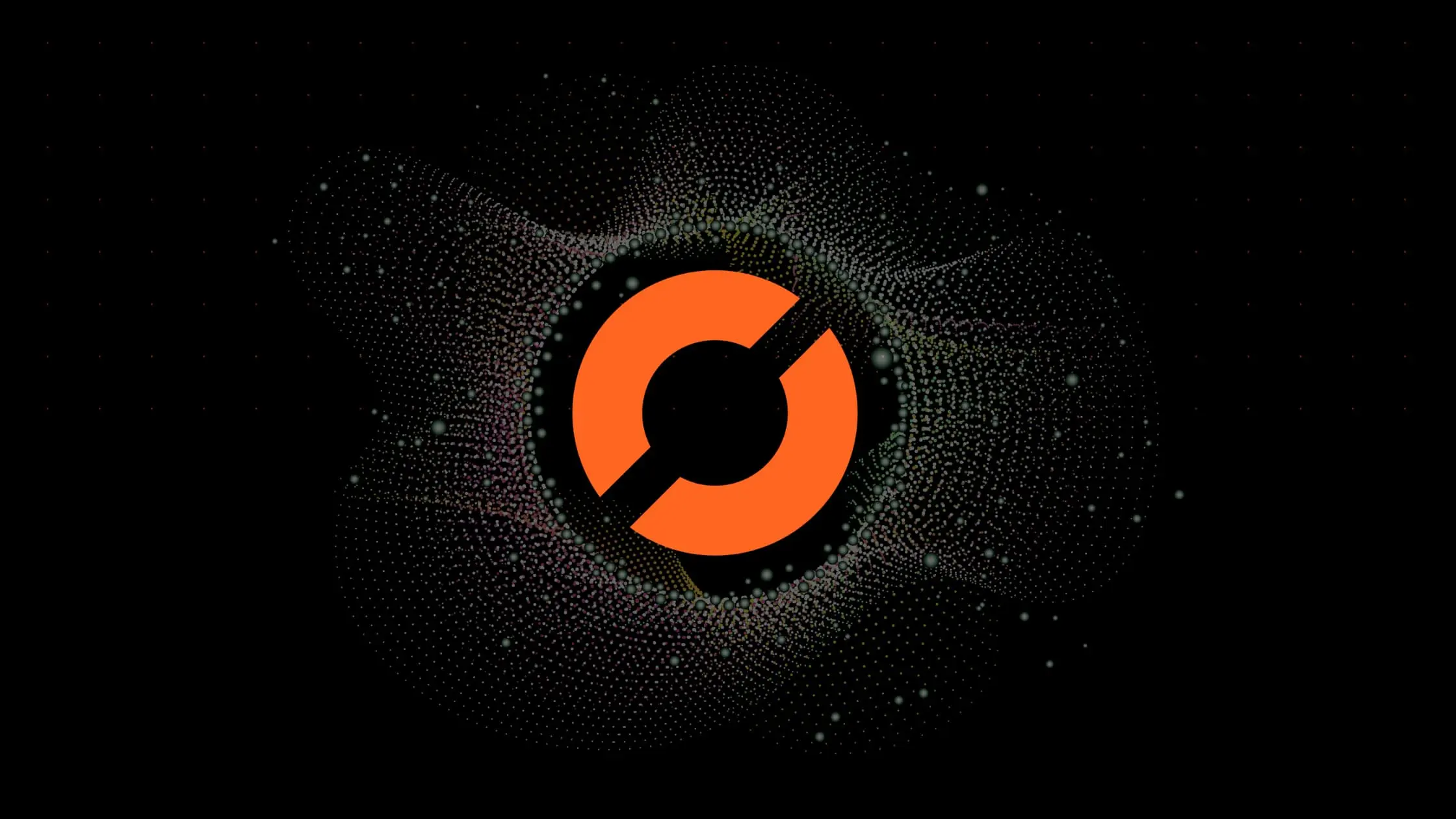
How to Get a List from a Pandas Dataframe Column
As a data scientist or software engineer, you may often need to extract a list of values from a specific column of a Pandas dataframe. This is a common task in data analysis and machine learning, and can be easily accomplished using a few lines of code in Python.
In this article, we will walk through the steps to extract a list from a Pandas dataframe column, including some examples and best practices. We will assume that you have a basic understanding of Python and Pandas.
What is Pandas?
Pandas is a popular open-source data analysis library for Python. It provides easy-to-use data structures and data analysis tools for manipulating and analyzing data in a flexible and efficient way. Pandas is widely used in data science, machine learning, and finance, among other fields.
How to Get a List from a Pandas Dataframe Column
To get a list of values from a specific column of a Pandas dataframe, you can use the tolist()
method of the Pandas Series object, which represents a column of the dataframe. Here is the general syntax:
df['column_name'].tolist()
where df
is the Pandas dataframe and column_name
is the name of the column from which you want to extract the list of values.
Let’s illustrate this with an example. Suppose we have a Pandas dataframe df
with two columns: ‘Name’ and ‘Age’, and we want to extract a list of ages. Here is the code to do this:
import pandas as pd
# create a sample dataframe
data = {'Name': ['Alice', 'Bob', 'Charlie', 'Dave'], 'Age': [25, 30, 35, 40]}
df = pd.DataFrame(data)
# extract a list of ages
ages = df['Age'].tolist()
print(ages)
Output:
[25, 30, 35, 40]
As you can see, the tolist()
method returns a list of values from the ‘Age’ column of the dataframe.
Best Practices
Use the tolist() Method
As we have seen, the tolist()
method is the simplest and most efficient way to extract a list from a Pandas dataframe column. It takes advantage of the optimized internal data structures of Pandas and NumPy to quickly convert a column of data into a list.
Check for Missing Values
Before extracting a list from a Pandas dataframe column, you should check for missing or NaN values in the column. These values can cause errors or unexpected behavior in your code, and may need to be handled separately depending on your analysis.
To check for missing values, you can use the isnull()
method of the Pandas Series object, which returns a Boolean mask indicating whether each value is missing or not. Here is an example:
# check for missing values in the 'Age' column
missing = df['Age'].isnull().any()
print(missing)
Output:
False
This code checks whether there are any missing values in the ‘Age’ column of the dataframe. In this case, there are no missing values, so the output is False
.
Handle Data Types
When extracting a list from a Pandas dataframe column, you should also consider the data type of the column. Depending on the data type, you may need to perform additional processing to convert the data into a list.
For example, if the column contains strings, you may need to remove whitespace or perform other cleaning operations before converting the data into a list. Similarly, if the column contains dates or times, you may need to convert the data into a different format before extracting a list.
Filter Data in Pandas
To filter data effectively, consider using Pandas' inherent data filtering capabilities. By filtering the data within Pandas and subsequently generating the list, you ensure that your code operates within the optimized data structures of Pandas. This results in more streamlined and concise code while achieving the desired outcome.
Here is an example filtering ages greater than 30 from the ‘Age’ column of the dataframe and coverting it to a list:
# extract a list of ages greater than 30
ages_gt_30 = df[df['Age'] > 30]['Age'].tolist()
print(ages_gt_30)
Output:
[35, 40]
Conclusion
In this article, we have seen how to extract a list from a Pandas dataframe column using the tolist()
method of the Pandas Series object. We have also discussed some best practices for handling missing values, data types, and list operations. By following these best practices, you can ensure that your code is efficient, robust, and easy to maintain.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.