How to Format Date in Spark SQL: A Guide for Data Scientists
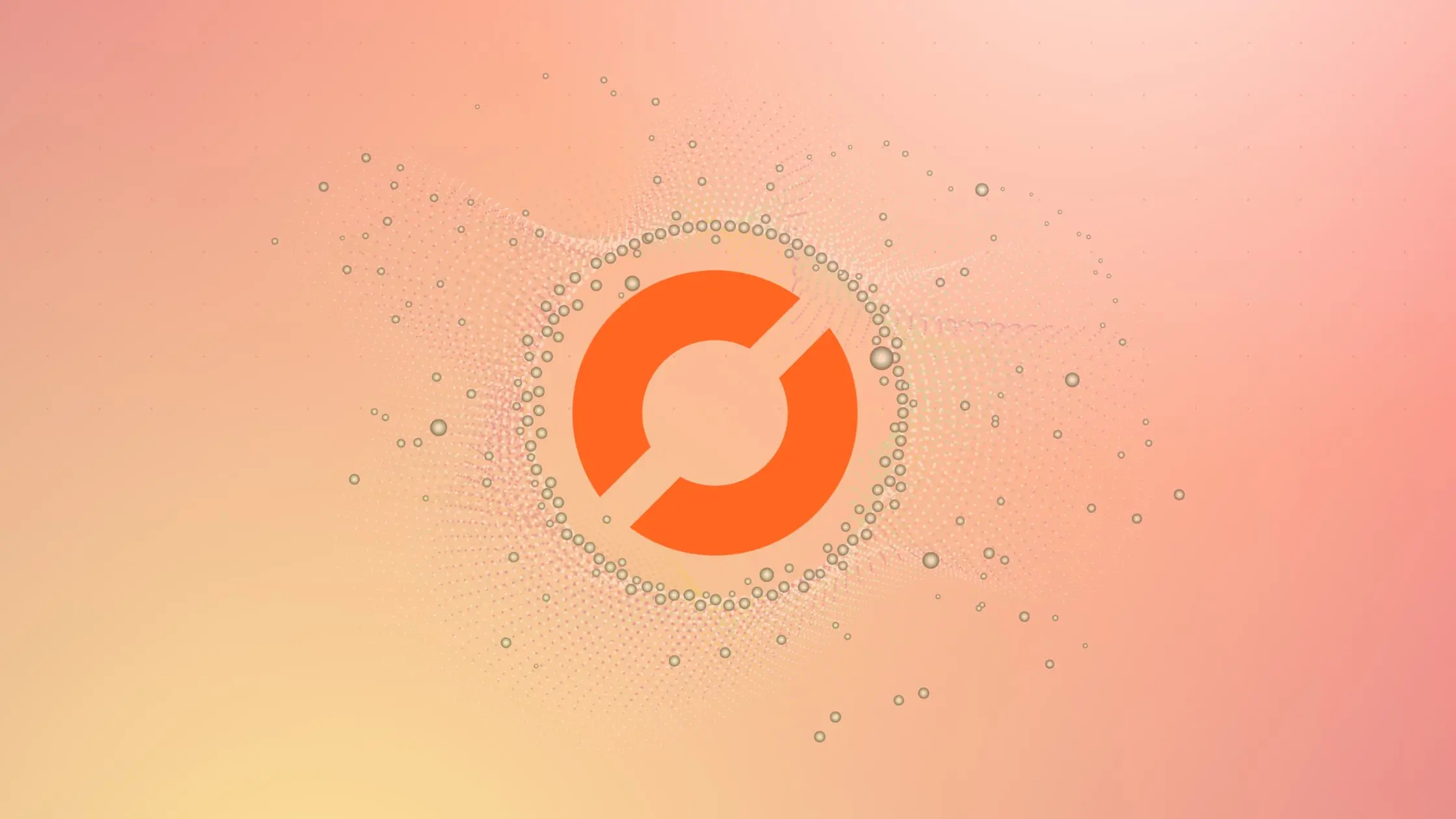
Spark SQL is a powerful tool for processing structured and semi-structured data. It provides a programming interface for data manipulation, including the ability to format dates. This guide will walk you through the process of formatting dates in Spark SQL, a crucial skill for data scientists.
Table of Contents
- Introduction to Spark SQL
- Understanding Date Formats in Spark SQL
- Formatting Dates in Spark SQL
- Converting Strings to Dates
- Dealing with Timestamps
- Basic Date Formatting
- Advanced Date Formatting
- Common Errors and Solutions
- Conclusion
Introduction to Spark SQL
Spark SQL is a module in Apache Spark that integrates relational processing with Spark’s functional programming API. It offers support for various data sources and makes it possible to weave SQL queries with code transformations, resulting in a powerful tool for big data analytics.
Understanding Date Formats in Spark SQL
Before we delve into formatting dates, it’s essential to understand the different date formats that Spark SQL supports. The most common ones include:
yyyy-MM-dd
oryyyy/MM/dd
: This is the standard date format, representing year, month, and day.dd-MM-yyyy
ordd/MM/yyyy
: This format represents day, month, and year.MM-dd-yyyy
orMM/dd/yyyy
: This format represents month, day, and year.
Formatting Dates in Spark SQL
Now, let’s dive into how to format dates in Spark SQL. We’ll use the date_format
function, which takes two arguments: the date column and the format to which you want to convert.
Here’s a simple example:
SELECT date_format('2023-06-30', 'MM/dd/yyyy') as formatted_date;
This will output 06/30/2023
.
Converting Strings to Dates
Often, you’ll have dates as strings that you need to convert to a date format. You can use the to_date
function for this. Here’s an example:
SELECT to_date('30-06-2023', 'dd-MM-yyyy') as date;
This will output 2023-06-30
.
Dealing with Timestamps
Timestamps are another common data type you’ll deal with. To convert a timestamp to a date, you can use the to_date
function without a format string:
SELECT to_date(cast(1625063346 as timestamp)) as date;
This will output 2023-06-30
.
Basic Date Formatting
Using to_date
Function
The to_date
function in Spark SQL is used to convert a string to a date. It takes two arguments - the column containing the date strings and the format of the date.
from pyspark.sql.functions import to_date
formatted_df = df.select(to_date("date", "yyyy-MM-dd").alias("formatted_date"))
formatted_df.show()
Output:
+--------------+
|formatted_date|
+--------------+
| 2023-06-30|
| 2023-07-01|
+--------------+
Using date_format
Function
The date_format
function allows you to format a date according to a specified pattern. It takes two arguments - the column with the date and the desired format.
from pyspark.sql.functions import date_format
formatted_df = df.select(date_format("date", "dd-MM-yyyy").alias("formatted_date"))
formatted_df.show()
Output:
+--------------+
|formatted_date|
+--------------+
| 30-06-2023|
| 01-07-2023|
+--------------+
Advanced Date Formatting
Changing Date Formats
To change the format of the date, you can use a combination of to_date
and date_format
functions.
from pyspark.sql.functions import to_date, date_format
formatted_df = df.select(date_format(to_date("date", "yyyy-MM-dd"), "MM/dd/yyyy").alias("formatted_date"))
formatted_df.show()
Output:
+--------------+
|formatted_date|
+--------------+
| 06/30/2023|
| 07/01/2023|
+--------------+
Extracting Date Components
Spark SQL provides functions like year
, month
, and day
to extract specific components from a date.
from pyspark.sql.functions import year, month, day
extracted_df = df.select(year("date").alias("year"), month("date").alias("month"), day("date").alias("day"))
extracted_df.show()
Output:
+----+-----+---+
|year|month|day|
+----+-----+---+
|2023| 6| 30|
|2023| 7| 1|
+----+-----+---+
Common Errors and Solutions
1. Error: “AnalysisException: cannot resolve…”
Description: This error occurs when the column specified in the function is not found in the DataFrame.
Example:
from pyspark.sql import SparkSession
from pyspark.sql.functions import to_date
# Create Spark session
spark = SparkSession.builder.appName("date_formatting").getOrCreate()
# Sample data
data = [("2023-06-30",), ("2023-07-01",)]
df = spark.createDataFrame(data, ["date"])
# Incorrect column name in the to_date function
formatted_df = df.select(to_date("incorrect_column", "yyyy-MM-dd").alias("formatted_date"))
Solution: Double-check the column name used in the function (to_date
in this case) to ensure it matches the actual column name in your DataFrame.
2. Error: “Py4JJavaError: An error occurred while calling…”
Description: This error indicates a Java exception during the execution of the Spark job.
Example:
from pyspark.sql import SparkSession
from pyspark.sql.functions import to_date
# Create Spark session
spark = SparkSession.builder.appName("date_formatting").getOrCreate()
# Sample data
data = [("2023-06-30",), ("2023-07-01",)]
df = spark.createDataFrame(data, ["date"])
# Incorrect date format in the to_date function
formatted_df = df.select(to_date("date", "yyyy/MM/dd").alias("formatted_date"))
formatted_df.show()
Solution: Check the Spark logs for more details on the Java exception. In this case, ensure that the date format specified in the to_date
function matches the actual format of the date strings in your DataFrame.
Conclusion
Formatting dates in Spark SQL is a crucial skill for data scientists working with big data. With the date_format
and to_date
functions, you can easily convert and format dates to suit your needs. Whether you’re working with SQL queries or DataFrames, Spark SQL provides the tools you need to handle dates effectively.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.