How to Flatten a List of Lists in Python
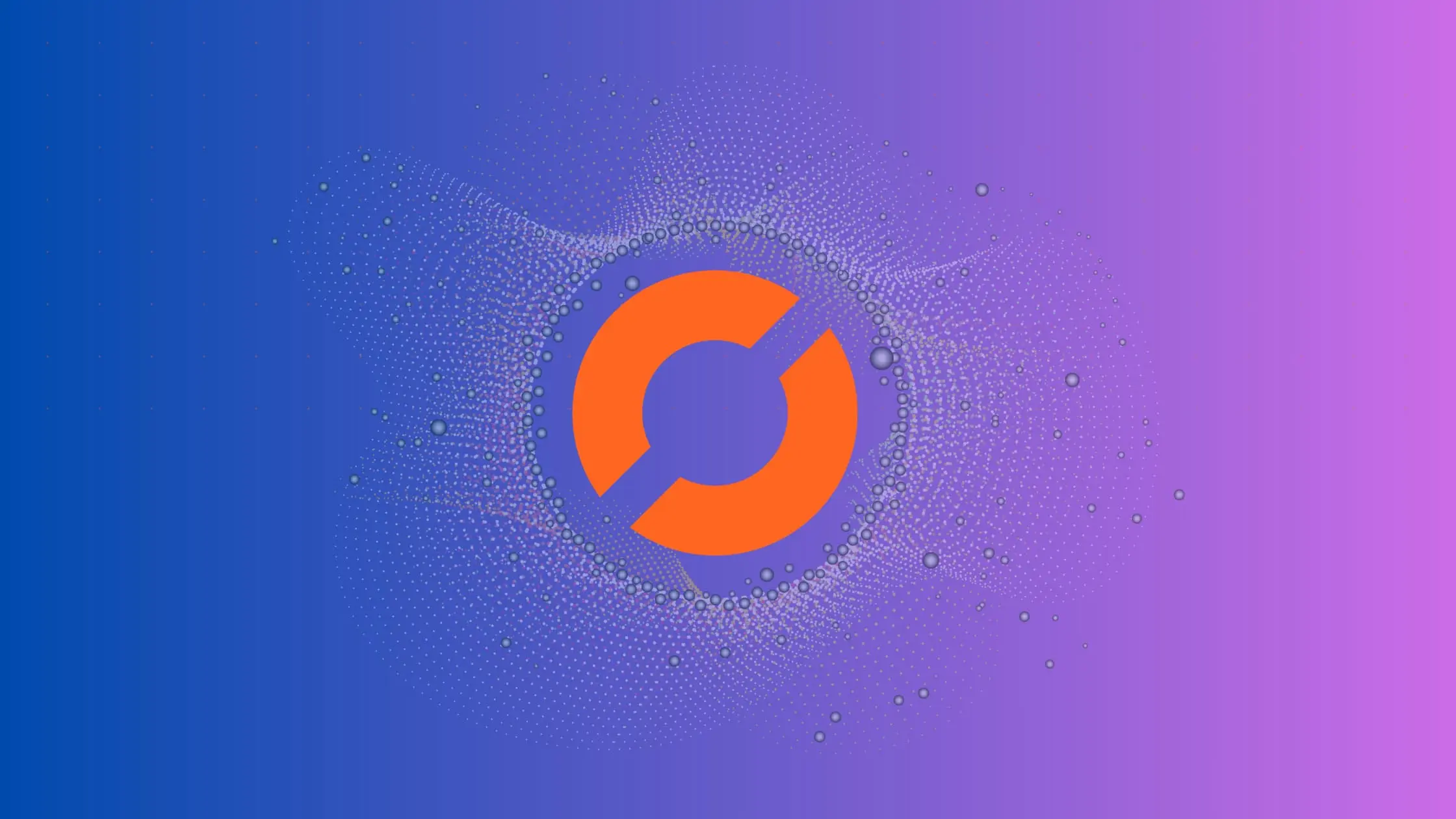
Flattening a list of lists can be useful when you want to perform operations on all the elements of the nested lists without having to iterate through each nested list separately. In this post, we’ll explore some techniques to flatten a list of lists in Python.
Method 1: Using Nested Loops
One way to flatten a list of lists is to use nested loops. This method is straightforward and easy to understand, but it may not be the most efficient for large lists.
def flatten_list(nested_list):
flat_list = []
for sublist in nested_list:
for item in sublist:
flat_list.append(item)
return flat_list
In this code, we define a function flatten_list
that takes a list of lists as an argument. We then create an empty list called flat_list
to store the flattened list. Next, we iterate over each sublist in the nested list using a for
loop. For each sublist, we iterate over each item in the sublist using another for
loop. We then append each item to the flat_list
. Finally, we return the flattened list.
Let’s test the function with an example:
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flat_list = flatten_list(nested_list)
print(flat_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
Generally, using nested loops is a simple and easy-to-understand method, but it may not be the most efficient for large lists.
Method 2: Using List Comprehension
Another way to flatten a list of lists is to use list comprehension. This method is more concise and often faster than using nested loops.
def flatten_list(nested_list):
return [item for sublist in nested_list for item in sublist]
In this code, we define a function flatten_list
that takes a list of lists as an argument. We then use list comprehension to iterate over each sublist in the nested list and each item in the sublist. We then return the flattened list.
Let’s test the function with the same example as before:
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flat_list = flatten_list(nested_list)
print(flat_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
List comprehension is a more concise and often faster method.
Method 3: Using itertools.chain()
The itertools
module in Python provides a chain()
function that can be used to flatten a list of lists.
import itertools
def flatten_list(nested_list):
return list(itertools.chain(*nested_list))
In this code, we first import the itertools
module. We then define a function flatten_list
that takes a list of lists as an argument. We use the chain()
function from the itertools
module to flatten the nested list. The *
operator is used to unpack the nested list into individual lists, which are then passed as arguments to the chain()
function. Finally, we convert the result to a list and return it.
Let’s test the function with the same example as before:
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flat_list = flatten_list(nested_list)
print(flat_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
Using the itertools.chain() function is always more effecient than the nested loops and the list comprehension methods
Method 4: Using Nested Functions
Another way to flatten a list of lists is to use nested functions. This method is more advanced and requires a good understanding of Python functions and recursion.
def flatten_list(nested_list):
def flatten(lst):
for item in lst:
if isinstance(item, list):
flatten(item)
else:
flat_list.append(item)
flat_list = []
flatten(nested_list)
return flat_list
In this code, we define a function flatten_list
that takes a list of lists as an argument. We then define a nested function flatten
that takes a list as an argument. We use recursion to flatten the nested list. For each item in the list, we check if it is another list using the isinstance()
function. If it is another list, we call the flatten()
function recursively. If it is not another list, we append the item to flat_list
. Finally, we call the flatten()
function with the nested list and return the flattened list.
Let’s test the function with the same example as before:
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flat_list = flatten_list(nested_list)
print(flat_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
Using nested functions is a more advanced and effecient method that requires a good understanding of Python functions and recursion.
Conclusion
In this post, we explored several techniques to flatten a list of lists in Python. Each method has its advantages and disadvantages, depending on the size of the nested list and the performance requirements of your application.
Using nested loops is a simple and easy-to-understand method, but it may not be the most efficient for large lists. List comprehension is a more concise and often faster method. Using the itertools.chain()
function is also a good option. Finally, using nested functions is a more advanced method that requires a good understanding of Python functions and recursion.
Regardless of which method you choose, flattening a list of lists can help simplify your code and make it more efficient.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.