How to Fix MemoryError Issues When Using Pandas in Python
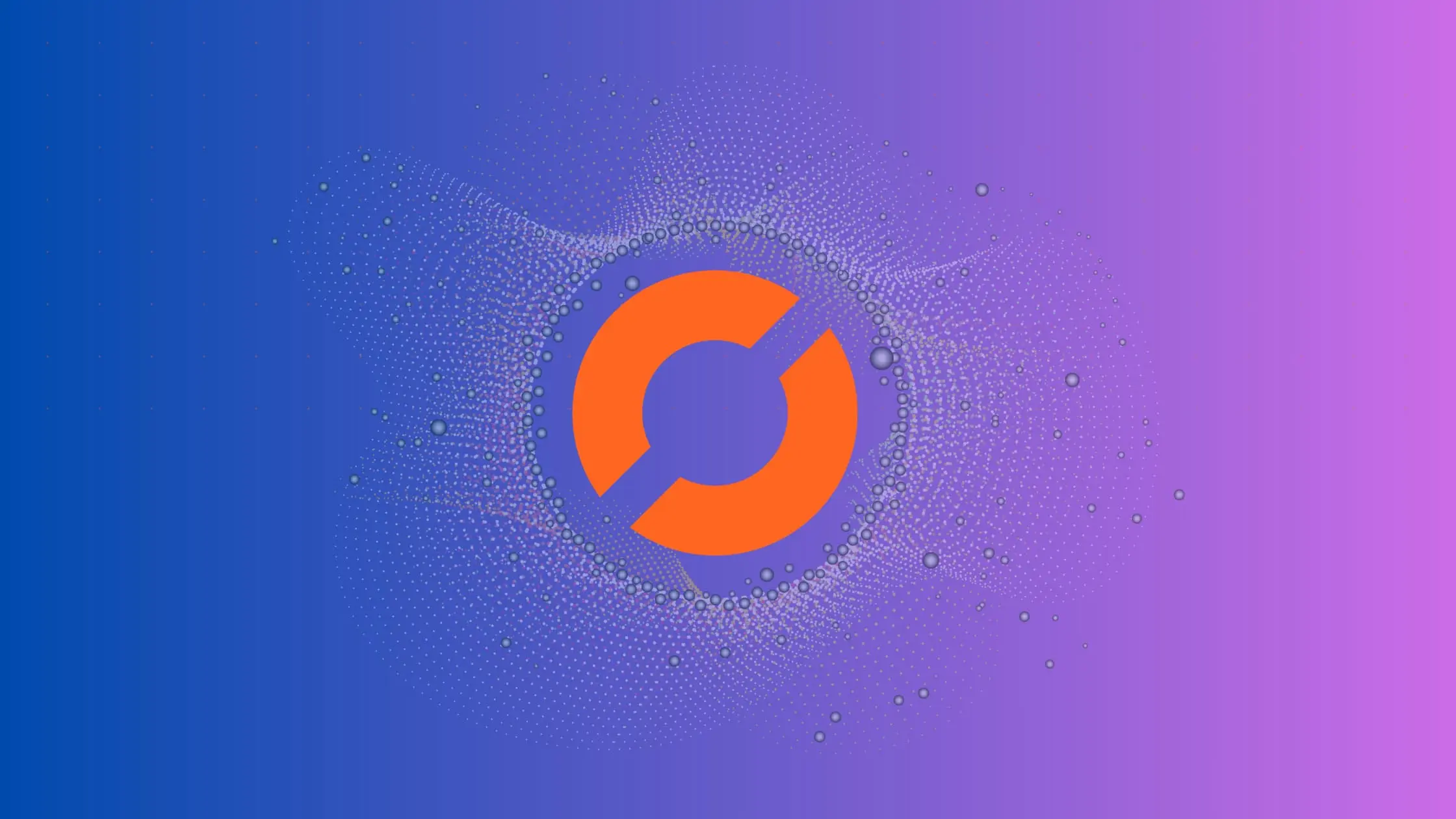
If you are a data scientist or software engineer who works with large datasets in Python, you may have encountered a MemoryError when using the popular data analysis library, Pandas. This error occurs when your system runs out of memory while trying to process a large dataset. In this article, we will explore the reasons behind this error and provide some solutions to help you fix it.
Table of Contents
- What is a MemoryError?
- Why Does this Error Occur in Pandas?
- How to Fix a MemoryError in Pandas
- Approaches to Overcome MemoryError
- Conclusion
What is a MemoryError?
A MemoryError is a common error in Python that occurs when the system runs out of memory. This error can occur when you are working with large datasets in Pandas, as Pandas loads the entire dataset into memory before performing any operations. This means that if your dataset is too large to fit into memory, you will encounter a MemoryError.
Why Does this Error Occur in Pandas?
Pandas is a powerful data analysis library that provides a lot of functionality for data manipulation and analysis. However, it is not optimized for working with very large datasets. When you load a dataset into a Pandas DataFrame, the entire dataset is loaded into memory. If your dataset is too large to fit into memory, you will run into a MemoryError.
Approaches to Overcome MemoryError
Method 1: Chunked Processing
Dividing the dataset into manageable chunks for individual processing prevents MemoryError. This approach is beneficial for operations that do not necessitate the entire dataset simultaneously.
import pandas as pd
chunk_size = 1000
reader = pd.read_csv('large_dataset.csv', chunksize=chunk_size)
for chunk in reader:
# Perform operations on each chunk
process_chunk(chunk)
Method 2: Optimize Data Types
Memory usage can be significantly reduced by optimizing data types. Convert columns to more memory-efficient types, such as using int
instead of float
where applicable.
df['column_name'] = df['column_name'].astype('int32')
Method 3: Increase System Memory
Upgrading system memory by adding more RAM or using cloud services with higher memory configurations is a straightforward solution.
Method 4: Leveraging Dask
Dask is a parallel computing library that seamlessly integrates with Pandas, allowing for distributed processing. This can be particularly advantageous for handling massive datasets without overwhelming system memory.
import dask.dataframe as dd
ddf = dd.read_csv('large_dataset.csv')
# Perform operations on dask dataframe
result = ddf.groupby('column_name').mean().compute()
Pros and Cons Comparison
Method | Pros | Cons |
---|---|---|
Chunked Processing | - Reduced memory footprint | - Increased complexity |
Optimize Data Types | - Improved memory efficiency | - Potential loss of precision |
Increase System Memory | - Immediate relief for memory constraints | - Hardware-dependent, may not be feasible |
Dask | - Scalable, distributed computing | - Learning curve, may not suit all scenarios |
Conclusion
In summary, a MemoryError in Pandas can occur when your dataset is too large to fit into memory. To fix this error, you can use chunking, use Dask, change the data type, or use a larger machine with more memory. By following these solutions, you can ensure that your data analysis projects run smoothly and efficiently, even with very large datasets.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.