How to Find Which Columns Contain Any NaN Value in Pandas DataFrame
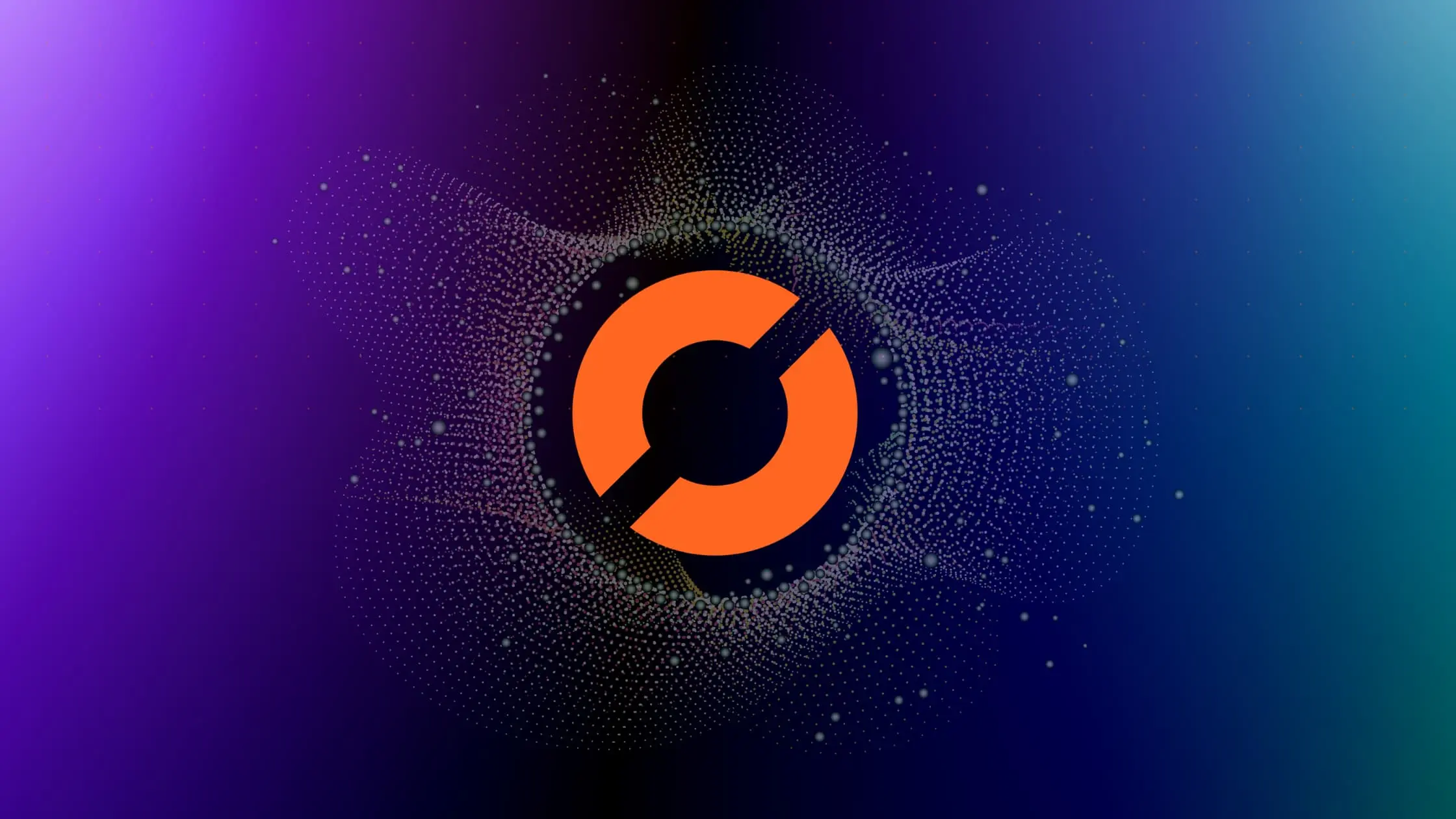
As a data scientist or software engineer, you often deal with large datasets that may contain missing or NaN values. These missing values can significantly impact the accuracy of your analysis or machine learning models. In this article, we will discuss how to find which columns contain any NaN value in a Pandas DataFrame.
What is Pandas?
Pandas is an open-source data analysis and manipulation library for Python. It provides data structures for efficiently storing and manipulating large datasets and tools for data cleaning, transformation, and analysis.
What are NaN Values?
NaN (Not a Number) is a special value used in Pandas to represent missing or undefined data. NaN values can occur due to various reasons such as data entry errors, incomplete data, or data conversion issues.
How to Check for NaN Values in Pandas DataFrame?
To check for NaN values in a Pandas DataFrame, you can use the isnull()
method. This method returns a DataFrame of the same shape as the input DataFrame, where each element is a boolean value indicating whether the corresponding element in the input DataFrame is NaN or not.
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({'A': [1, 2, 3, 4, 5], 'B': [6, 7, 8, None, 10], 'C': [11, 12, None, 14, 15]})
# check for NaN values
print(df.isnull())
Output:
A B C
0 False False False
1 False False False
2 False False True
3 False True False
4 False False False
In the above example, we created a sample DataFrame with three columns ‘A’, ‘B’, and ‘C’. Column ‘B’ and ‘C’ contain NaN values. Using the isnull()
method, we checked for NaN values and got a boolean DataFrame indicating the location of NaN values in each column.
How to Find Which Columns Contain Any NaN Value in Pandas DataFrame?
To find which columns contain any NaN value in a Pandas DataFrame, we can use the any()
method along with the isnull()
method. The any()
method returns a boolean value indicating whether any element in the input DataFrame is True or not.
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({'A': [1, 2, 3, 4, 5], 'B': [6, 7, 8, None, 10], 'C': [11, 12, None, 14, 15]})
# check for NaN values in each column
print(df.isnull().any())
Output:
A False
B True
C True
dtype: bool
In the above example, we used the isnull()
method to check for NaN values in each column and then applied the any()
method to each column. The output shows the boolean value indicating whether the corresponding column contains any NaN value or not.
How to Count NaN Values in Pandas DataFrame?
To count the number of NaN values in each column of a Pandas DataFrame, we can use the sum()
method along with the isnull()
method. The sum()
method returns the sum of all elements in the input DataFrame.
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({'A': [1, 2, 3, 4, 5], 'B': [6, 7, 8, None, 10], 'C': [11, 12, None, 14, 15]})
# count NaN values in each column
print(df.isnull().sum())
Output:
A 0
B 1
C 1
dtype: int64
In the above example, we used the isnull()
method to check for NaN values in each column and then applied the sum()
method to each column. The output shows the number of NaN values in each column.
Conclusion
In this article, we discussed how to find which columns contain any NaN value in a Pandas DataFrame. We learned how to check for NaN values using the isnull()
method, find which columns contain any NaN value using the any()
method, and count the number of NaN values in each column using the sum()
method. By using these methods, we can efficiently handle missing data in our data analysis or machine learning projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.