How to Find All Rows with NaN Values in Python Pandas
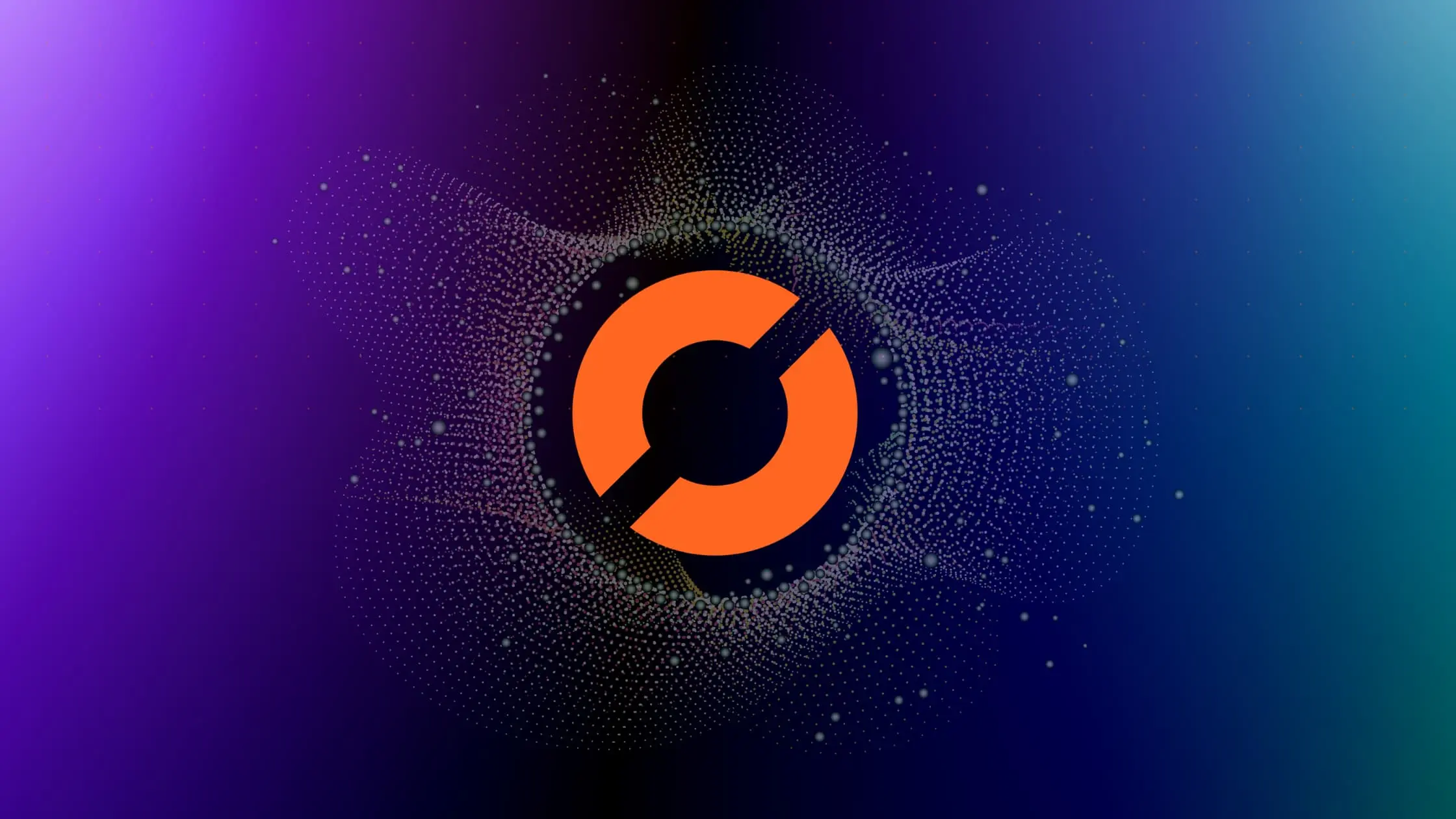
As a data scientist or software engineer, working with large datasets is a common task. In the process of analyzing data, it is not uncommon to encounter missing values. Missing values can be represented in different ways, but in Python Pandas, they are represented as NaN (Not a Number) values.
In this article, we will explore how to find all rows with NaN values in Python Pandas. We will cover different approaches to handle missing values, and how to determine which approach is the best for your data.
What are NaN values in Python Pandas?
NaN values are used to represent missing or undefined values in Python Pandas. They are a special floating-point value and can be created using the numpy.nan
function or by loading data containing missing values.
Consider the following example:
import pandas as pd
import numpy as np
data = {'Name': ['John', 'Mary', 'Luke', 'Peter', 'Jane', 'Alice'],
'Age': [32, np.nan, 25, np.nan, 29, 40],
'Gender': ['M', 'F', 'M', 'M', 'F', 'F'],
'Salary': [50000, 60000, np.nan, 70000, 80000, np.nan]}
df = pd.DataFrame(data)
print(df)
This creates a Pandas DataFrame with four columns: Name, Age, Gender, and Salary. The Age and Salary columns contain NaN values, which represent missing data.
Name Age Gender Salary
0 John 32.0 M 50000.0
1 Mary NaN F 60000.0
2 Luke 25.0 M NaN
3 Peter NaN M 70000.0
4 Jane 29.0 F 80000.0
5 Alice 40.0 F NaN
How to Find Rows with NaN Values in Python Pandas
In Python Pandas, there are different approaches to handle missing data. The approach you choose depends on the nature of your data and the analysis you want to perform.
To find all rows with NaN values in a Pandas DataFrame, you can use the isna()
function. This function returns a DataFrame of the same shape as the input, but with boolean values indicating where NaN values are present.
nan_df = df.isna()
print(nan_df)
This returns the following DataFrame:
Name Age Gender Salary
0 False False False False
1 False True False False
2 False False False True
3 False True False False
4 False False False False
5 False False False True
Each cell in the DataFrame is either True or False, depending on whether NaN is present in that cell.
To find all rows with NaN values, you can use the any()
function, which returns True if any NaN value is present in a row.
nan_rows = df.isna().any(axis=1)
print(nan_rows)
This returns a Series with boolean values indicating which rows contain NaN values.
0 False
1 True
2 True
3 True
4 False
5 True
dtype: bool
In this example, rows 1, 2, 3, and 5 contain NaN values.
Handling NaN Values in Python Pandas
Handling NaN values is an essential part of data analysis. Depending on the nature of your data and the analysis you want to perform, you can choose different approaches to handle missing data.
Drop NaN Values
One approach to handling NaN values is to drop all rows containing NaN values. You can use the dropna()
function to remove all rows containing NaN values.
clean_df = df.dropna()
print(clean_df)
This returns a DataFrame with all rows containing NaN values removed.
Name Age Gender Salary
0 John 32.0 M 50000.0
4 Jane 29.0 F 80000.0
Fill NaN Values
Another approach to handling NaN values is to fill them with a value. You can use the fillna()
function to replace all NaN values with a specified value.
fill_df = df.fillna(0)
print(fill_df)
This returns a DataFrame with all NaN values replaced with 0.
Name Age Gender Salary
0 John 32.0 M 50000.0
1 Mary 0.0 F 60000.0
2 Luke 25.0 M 0.0
3 Peter 0.0 M 70000.0
4 Jane 29.0 F 80000.0
5 Alice 40.0 F 0.0
You can also replace NaN values with the mean, median, or mode of the column.
mean_age = df['Age'].mean()
median_salary = df['Salary'].median()
mean_df = df.fillna({'Age': mean_age, 'Salary': median_salary})
print(mean_df)
This returns a DataFrame with NaN values in the Age and Salary columns replaced with the mean and median of their respective columns.
Name Age Gender Salary
0 John 32.0 M 50000.0
1 Mary 32.0 F 60000.0
2 Luke 25.0 M 65000.0
3 Peter 32.0 M 70000.0
4 Jane 29.0 F 80000.0
5 Alice 40.0 F 65000.0
Interpolate NaN Values
Another approach to handling NaN values is to interpolate them. Interpolation is the process of estimating missing values based on the values of neighboring data points.
You can use the interpolate()
function to interpolate NaN values.
interp_df = df.interpolate()
print(interp_df)
This returns a DataFrame with NaN values interpolated.
Name Age Gender Salary
0 John 32.0 M 50000.0
1 Mary 28.5 F 60000.0
2 Luke 25.0 M 65000.0
3 Peter 27.0 M 70000.0
4 Jane 29.0 F 80000.0
5 Alice 40.0 F 80000.0
In this example, Nan in both Age column and Salary column are filled using interpolation method.
Conclusion
In this article, we explored how to find all rows with NaN values in Python Pandas. We also covered different approaches to handle missing data, including dropping NaN values, filling NaN values, and interpolating NaN values.
Handling missing data is an essential part of data analysis, and choosing the best approach depends on the nature of your data and the analysis you want to perform. By using the techniques outlined in this article, you can effectively handle missing data in your Python Pandas projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.