How to Filter Pandas DataFrames on Dates
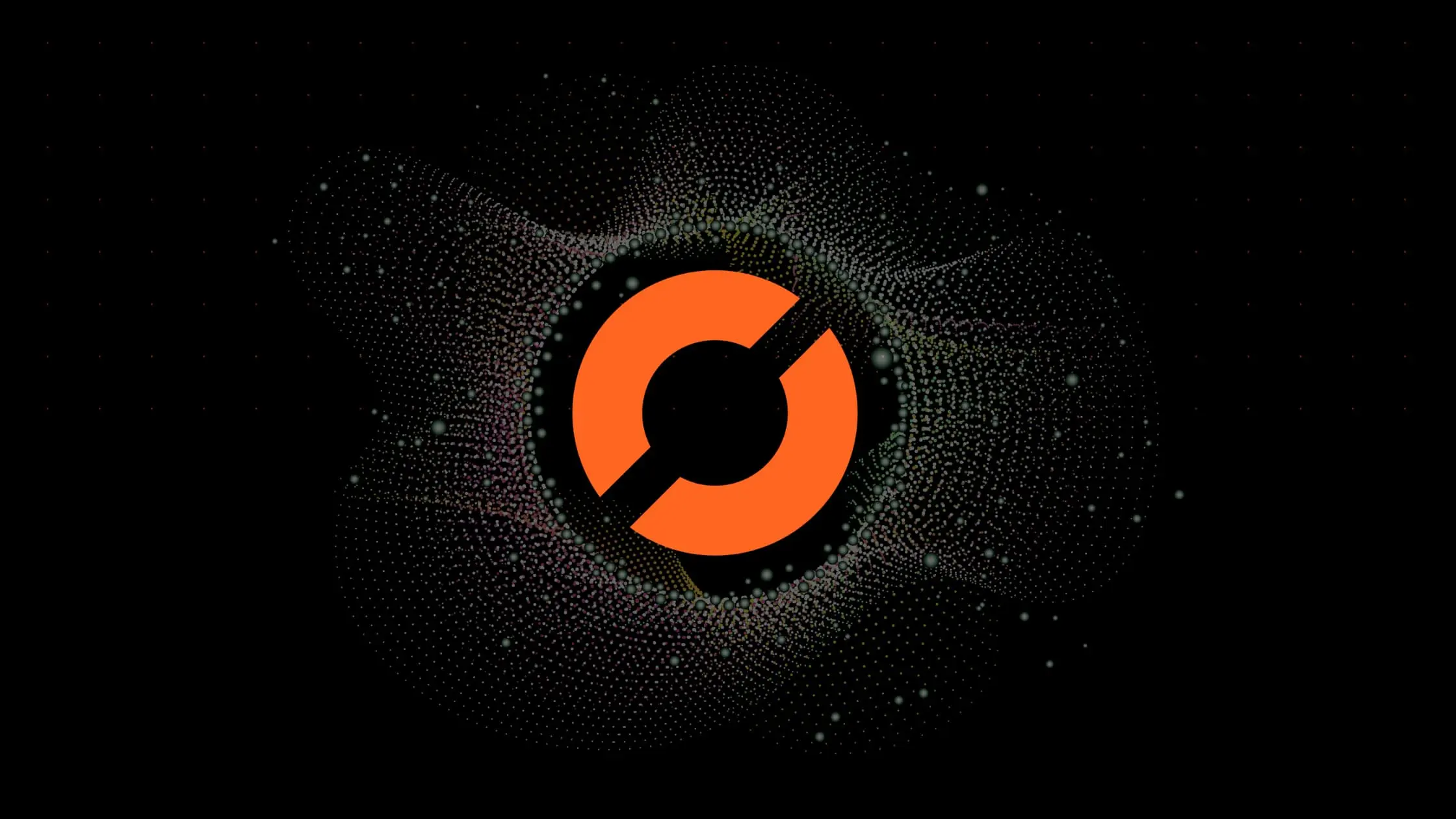
As a data scientist or software engineer, you know that working with dates in pandas can be a bit tricky. Fortunately, pandas provides powerful tools for filtering data based on dates. In this article, we’ll explore how to filter pandas DataFrames on dates, including a few examples of common use cases.
What Are Pandas DataFrames?
Before we dive into filtering pandas DataFrames on dates, let’s first define what a DataFrame is. A DataFrame is a two-dimensional labeled data structure with columns of potentially different types. You can think of it like a spreadsheet or SQL table. Each column in a DataFrame can have a different data type, such as integers, floats, or strings.
Pandas is a popular data analysis library in Python that provides data structures and functions for working with structured data. The DataFrame is one of the key data structures in pandas, and it provides a powerful and flexible way to work with tabular data.
Filtering Pandas DataFrames on Dates
Filtering pandas DataFrames on dates can be done using the loc
method. The loc
method is used to access a group of rows and columns by label(s) or a boolean array. Here’s an example:
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({
'date': pd.date_range(start='2020-01-01', end='2020-01-10'),
'value': [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
})
# filter rows where date is greater than 2020-01-05
filtered_df = df.loc[df['date'] > '2020-01-05']
print(filtered_df)
This code creates a simple DataFrame with two columns: date
and value
. The date
column contains a range of dates from January 1, 2020, to January 10, 2020. The value
column contains a corresponding set of values from 1 to 10.
The loc
method is then used to filter the DataFrame. The df['date'] > '2020-01-05'
expression creates a boolean array that is True
for rows where the date
column is greater than January 5, 2020. The loc
method is then used to access the rows where this condition is True
.
The resulting DataFrame contains rows where the date
column is greater than January 5, 2020, as shown below:
date value
5 2020-01-06 6
6 2020-01-07 7
7 2020-01-08 8
8 2020-01-09 9
9 2020-01-10 10
Filtering Pandas DataFrames on Date Ranges
In addition to filtering pandas DataFrames on specific dates, you can also filter on date ranges. Here’s an example:
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({
'date': pd.date_range(start='2020-01-01', end='2020-01-10'),
'value': [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
})
# filter rows where date is between 2020-01-05 and 2020-01-08
filtered_df = df.loc[(df['date'] >= '2020-01-05') & (df['date'] <= '2020-01-08')]
print(filtered_df)
This code creates the same DataFrame as before, but it filters on a date range instead of a specific date. The df['date'] >= '2020-01-05'
expression creates a boolean array that is True
for rows where the date
column is greater than or equal to January 5, 2020. The df['date'] <= '2020-01-08'
expression creates a boolean array that is True
for rows where the date
column is less than or equal to January 8, 2020. The &
operator is used to combine these boolean arrays using element-wise logical AND.
The resulting DataFrame contains rows where the date
column is between January 5, 2020, and January 8, 2020, as shown below:
date value
4 2020-01-05 5
5 2020-01-06 6
6 2020-01-07 7
7 2020-01-08 8
Filtering Pandas DataFrames on Date Ranges with Time Zones
If you’re working with dates that have time zone information, you may need to perform date filtering while taking time zones into account. Here’s an example:
import pandas as pd
from pytz import timezone
# create a sample DataFrame with time zone information
df = pd.DataFrame({
'date': pd.date_range(start='2020-01-01', end='2020-01-10', tz=timezone('US/Eastern')),
'value': [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
})
# filter rows where date is between 2020-01-05 and 2020-01-08 in the US/Pacific time zone
filtered_df = df.loc[(df['date'].dt.tz_convert(timezone('US/Pacific')) >= '2020-01-05') & (df['date'].dt.tz_convert(timezone('US/Pacific')) <= '2020-01-08')]
print(filtered_df)
This code creates the same DataFrame as before, but with time zone information. The pytz
library is used to create a timezone
object for the US/Eastern time zone. The tz
parameter is then used to specify this time zone when creating the date
column.
To filter on a date range in a different time zone (US/Pacific in this example), the dt.tz_convert
method is used to convert the date
column to the desired time zone. The resulting DataFrame contains rows where the date
column is between January 5, 2020, and January 8, 2020, in the US/Pacific time zone, as shown below:
date value
5 2020-01-06 00:00:00-05:00 6
6 2020-01-07 00:00:00-05:00 7
7 2020-01-08 00:00:00-05:00 8
Conclusion
Filtering pandas DataFrames on dates is a common task for data scientists and software engineers. Fortunately, pandas provides powerful tools for filtering data based on dates. In this article, we covered how to filter pandas DataFrames on dates, including examples of common use cases. By using the loc
method and boolean arrays, you can easily filter DataFrames on specific dates or date ranges. By taking time zones into account, you can perform date filtering across different time zones. With these tools, you can effectively work with dates in pandas and perform powerful data analysis.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.