How to Filter Pandas Dataframe by Time
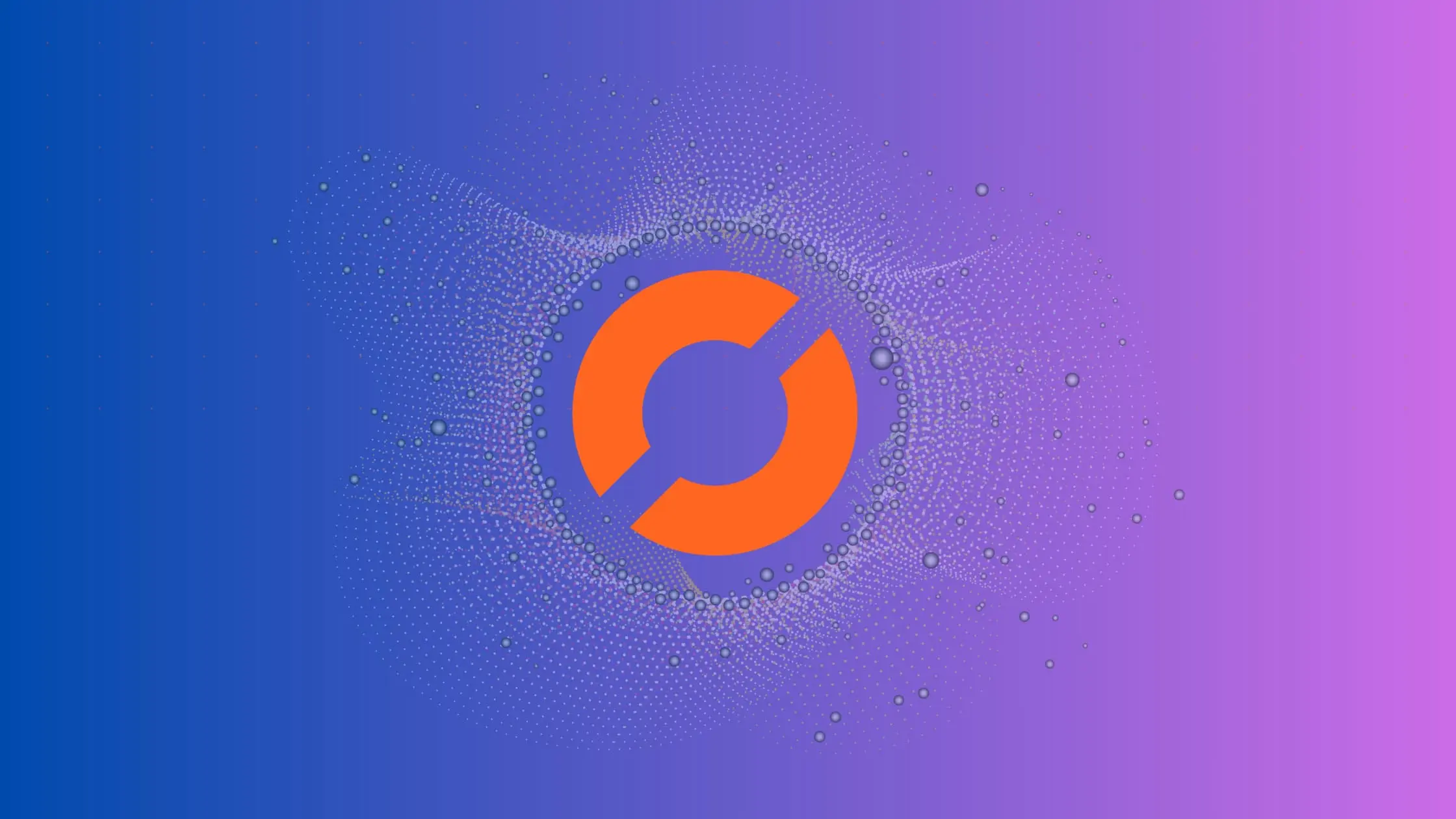
As a data scientist, it is often necessary to filter data using time-based criteria. Pandas is a popular data analysis library in Python that offers robust and flexible tools for working with time series data. In this article, we will explore how to filter a Pandas dataframe by time.
Table of Contents
- What is a Pandas Dataframe?
- Filtering Pandas Dataframe by Time
- Common Errors and Solutions
- Best Practices
- Conclusion
What is a Pandas Dataframe?
Before we dive into filtering by time in Pandas, let’s first define what a Pandas dataframe is. A Pandas dataframe is a two-dimensional, size-mutable, and tabular data structure that consists of rows and columns. Each column can have a different data type (e.g., integer, float, string), and each row represents a unique observation or record in the dataset.
Filtering Pandas Dataframe by Time
Filtering a Pandas dataframe by time involves selecting a subset of rows that meet a specific time-based criteria. There are several ways to filter a Pandas dataframe by time, depending on the format of the time data. In this article, we will focus on two main formats: datetime and timestamp.
Filtering by Datetime
Datetime is a Python module that provides classes for working with dates and times. In Pandas, datetime data can be stored in a column as a datetime object, which can then be used to filter the dataframe.
Suppose we have a Pandas dataframe that contains stock prices for a specific company over a period of time.
date price
0 2022-01-01 100
1 2022-01-02 110
2 2022-01-03 105
3 2022-01-04 115
4 2022-01-05 120
We can filter the dataframe to only include data from a certain date and time using the following code:
import pandas as pd
# create dataframe
df = pd.DataFrame({
'date': ['2022-01-01', '2022-01-02', '2022-01-03', '2022-01-04', '2022-01-05'],
'price': [100, 110, 105, 115, 120]
})
# convert date column to datetime object
df['date'] = pd.to_datetime(df['date'])
# filter dataframe by date
start_date = '2022-01-03'
end_date = '2022-01-05'
mask = (df['date'] >= start_date) & (df['date'] <= end_date)
filtered_df = df.loc[mask]
print(filtered_df)
In the above code, we first create a dataframe that contains the date and price columns. We then convert the date column to a datetime object using the pd.to_datetime()
method. Finally, we filter the dataframe to only include data between January 3, 2022, and January 5, 2022, using the >=
and <=
operators and the &
operator to create a boolean mask. The resulting filtered dataframe only contains the rows that meet the time-based criteria.
Output:
date price
2 2022-01-03 105
3 2022-01-04 115
4 2022-01-05 120
Filtering by Timestamp
Timestamp is a data type that represents a point in time. In Pandas, timestamp data can be stored in a column as a timestamp object, which can then be used to filter the dataframe.
Suppose we have a Pandas dataframe that contains sales data for a specific product over a period of time.
timestamp sales
0 2022-01-01 08:00:00 100
1 2022-01-01 12:00:00 150
2 2022-01-02 08:00:00 120
3 2022-01-02 12:00:00 170
4 2022-01-03 08:00:00 130
We can filter the dataframe to only include data from a certain time of day using the following code:
import pandas as pd
# create dataframe
df = pd.DataFrame({
'timestamp': ['2022-01-01 08:00:00', '2022-01-01 12:00:00', '2022-01-02 08:00:00', '2022-01-02 12:00:00', '2022-01-03 08:00:00'],
'sales': [100, 150, 120, 170, 130]
})
# convert timestamp column to timestamp object
df['timestamp'] = pd.to_datetime(df['timestamp'])
# filter dataframe by time
start_time = '08:00:00'
end_time = '12:00:00'
mask = (df['timestamp'].dt.time >= pd.Timestamp(start_time).time()) & (df['timestamp'].dt.time <= pd.Timestamp(end_time).time())
filtered_df = df.loc[mask]
print(filtered_df)
In the above code, we first create a dataframe that contains the timestamp and sales columns. We then convert the timestamp column to a timestamp object using the pd.to_datetime()
method. Finally, we filter the dataframe to only include data between 8:00 AM and 12:00 PM using the dt.time
attribute and the pd.Timestamp()
method to create a timestamp object. The resulting filtered dataframe only contains the rows that meet the time-based criteria.
Output:
timestamp sales
0 2022-01-01 08:00:00 100
2 2022-01-02 08:00:00 120
4 2022-01-03 08:00:00 130
Common Errors and Solutions:
Incorrect Date/Time Format:
Error: One common mistake is providing date/time data in an incorrect format.
# Incorrect date format df = pd.DataFrame({ 'date': ['01-01-2022', '01-02-2022', '01-03-2022', '01-04-2022', '01-05-2022'], 'price': [100, 110, 105, 115, 120] })
Solution: Ensure that the date column is in the correct format using the
pd.to_datetime
method.df['date'] = pd.to_datetime(df['date'], format='%m-%d-%Y')
Empty DataFrame After Filtering:
Error: If the time range specified for filtering does not match any rows, the resulting dataframe might be empty.
start_date = '2022-01-06' end_date = '2022-01-10' mask = (df['date'] >= start_date) & (df['date'] <= end_date) filtered_df = df.loc[mask]
Solution: Double-check the time range or ensure that the data within the specified range exists in the dataframe.
Mismatched Data Types:
Error: Incorrect data types in the date/time columns can lead to filtering errors.
# Incorrect data type for date df['date'] = df['date'].astype(str) start_date = '2022-01-03' end_date = '2022-01-05' mask = (df['date'] >= start_date) & (df['date'] <= end_date) filtered_df = df.loc[mask]
Solution: Ensure that the date column is of type datetime before applying filters.
df['date'] = pd.to_datetime(df['date'])
Best Practices:
Consistent Date/Time Formatting:
- Always maintain a consistent date/time format throughout your dataset. This ensures accurate filtering and prevents errors.
Use Pandas Native Functions:
- Leverage Pandas native functions, such as
pd.to_datetime
, for converting date/time columns. This ensures accurate type conversion.
- Leverage Pandas native functions, such as
Avoid Hardcoding Dates/Times:
- Avoid hardcoding specific dates or times in your filtering conditions. Instead, use variables to make the code more flexible.
Handle Timezone Differences:
- If your dataset involves different timezones, consider normalizing them to a common timezone to avoid complications during filtering.
Validate Filtered Results:
- Always validate the filtered results, especially when dealing with large datasets, to ensure that the filtering criteria are applied correctly.
Conclusion
Filtering a Pandas dataframe by time is a common task in data analysis and can be accomplished using a variety of methods depending on the format of the time data. In this article, we explored how to filter a Pandas dataframe by datetime and timestamp, using real-world examples of stock prices and sales data. By following these examples and understanding the underlying principles, you can easily apply time-based filtering to your own datasets and gain valuable insights from your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.