How to Filter for a List of Values in Python Pandas Using Loc
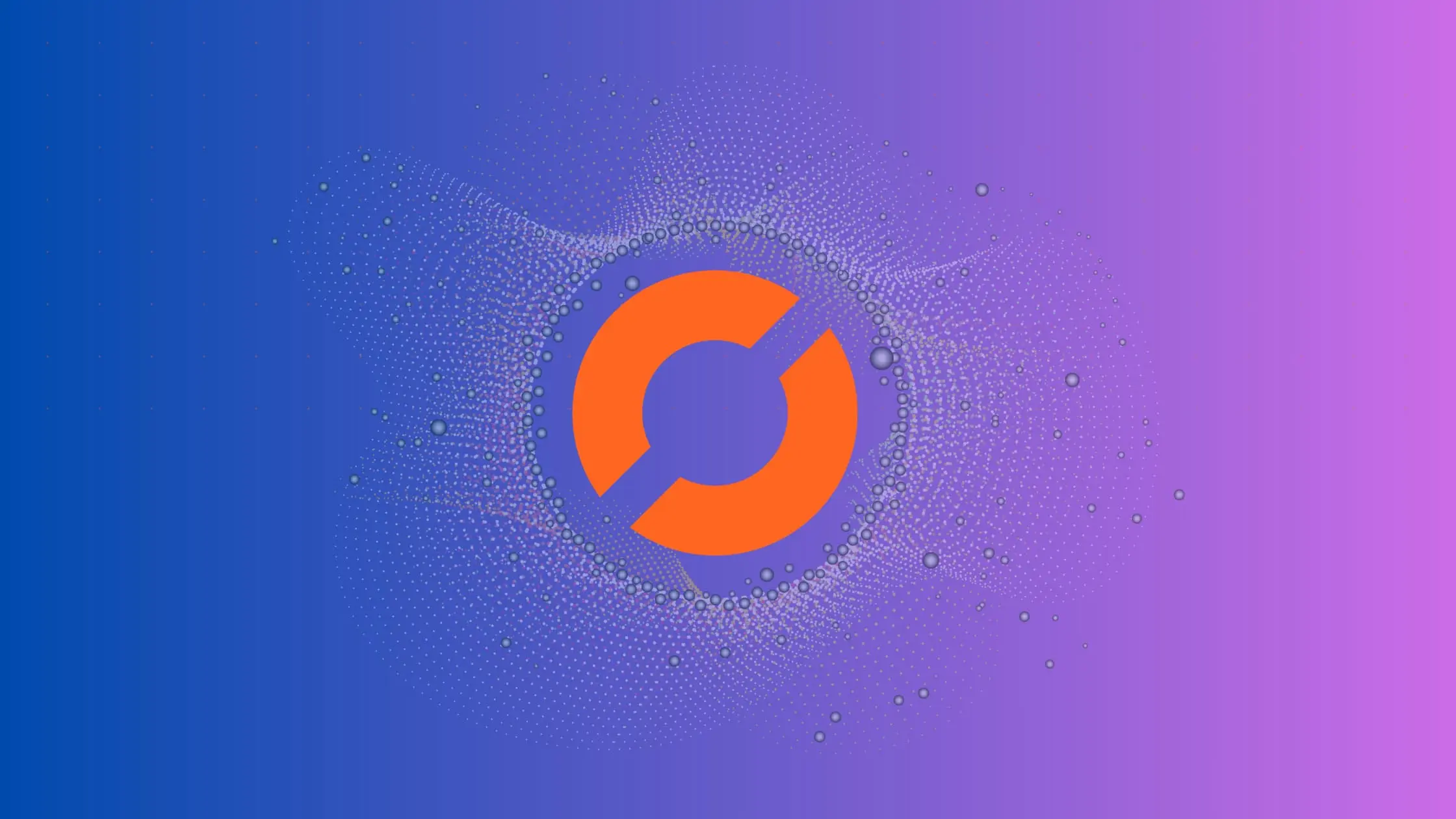
As a data scientist or software engineer, filtering data is a common task when working with datasets. One of the most powerful tools for filtering data in Python is the pandas library, which provides a wide range of functions to help you extract and manipulate data.
In this article, we will explore how to filter for a list of values in a pandas dataframe using the loc function. Specifically, we will explain how to use the loc function to filter a dataframe based on a list of values in one or more columns.
Table of Contents
- What Is the Loc Function in Python Pandas?
- How to Filter for a List of Values Using the Loc Function
- Common Errors and How to Handle Them
- Conclusion
What Is the Loc Function in Python Pandas?
The loc function is a powerful tool in the pandas library for selecting data from a dataframe based on a set of labels. It is commonly used to select subsets of data based on row and column labels. The loc function takes two arguments, the row label and the column label, and returns a subset of the dataframe that matches the specified labels.
Here is a simple example of how to use the loc function to select a subset of data from a pandas dataframe:
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'city': ['New York', 'San Francisco', 'Chicago', 'Los Angeles']})
# use loc to select rows where the age is greater than or equal to 35
result = df.loc[df['age'] >= 35]
# print the result
print(result)
Output:
name age city
2 Charlie 35 Chicago
3 David 40 Los Angeles
In this example, we used the loc function to select all rows where the ‘age’ column is greater than or equal to 35. The resulting dataframe contains only the rows that match this condition.
How to Filter for a List of Values Using the Loc Function
Now that we have a basic understanding of the loc function, let’s explore how to use it to filter a dataframe based on a list of values in one or more columns.
Suppose we have a dataframe with the following columns:
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'city': ['New York', 'San Francisco', 'Chicago', 'Los Angeles']})
If we want to filter this dataframe based on a list of values in the ‘city’ column, we can use the isin method, which returns a boolean series indicating whether each element of the dataframe is contained in the specified list of values.
# create a list of values to filter for
cities = ['New York', 'Chicago']
# filter the dataframe using loc and isin
result = df.loc[df['city'].isin(cities)]
# print the result
print(result)
Output:
name age city
0 Alice 25 New York
2 Charlie 35 Chicago
In this example, we used the loc function to filter the dataframe based on the list of values in the city
column. The resulting dataframe contains only the rows where the city
column matches one of the values in the list.
We can also filter for a list of values in multiple columns by using the & operator to combine multiple conditions. For example, suppose we want to filter the dataframe based on a list of values in both the ‘city’ and ‘age’ columns:
# create a list of values to filter for
cities = ['New York', 'Chicago']
ages = [25, 35]
# filter the dataframe using loc and isin
result = df.loc[(df['city'].isin(cities)) & (df['age'].isin(ages))]
# print the result
print(result)
Output:
name age city
0 Alice 25 New York
2 Charlie 35 Chicago
In this example, we used the loc function to filter the dataframe based on a list of values in both the ‘city’ and ‘age’ columns. The resulting dataframe contains only the rows where the ‘city’ column matches one of the values in the list and the ‘age’ column matches one of the values in the second list.
Common Errors and How to Handle Them
Error 1: Mismatched Data Types
One common error is dealing with mismatched data types between the DataFrame and the list of values. To address this, we can use the astype
method to ensure compatibility.
Error 2: Missing Values in the List
Handling missing values in the list is crucial. We’ll explore how to identify and handle situations where the list contains NaN or null values.
Error 3: Incorrect Syntax
Incorrect syntax can lead to unexpected results. We’ll walk through examples of common syntax errors and how to correct them.
Conclusion
Filtering data is a common task in data science and software engineering, and the pandas library provides a wide range of tools to help you extract and manipulate data. In this article, we explored how to filter for a list of values in a pandas dataframe using the loc function. We demonstrated how to use the isin method to filter based on a list of values in one column and how to combine multiple conditions using the & operator to filter based on a list of values in multiple columns. With these tools, you can easily extract and manipulate subsets of data from large datasets, making it easier to analyze and visualize your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.