How to Extract Substring from an Entire Column in Pandas Dataframe
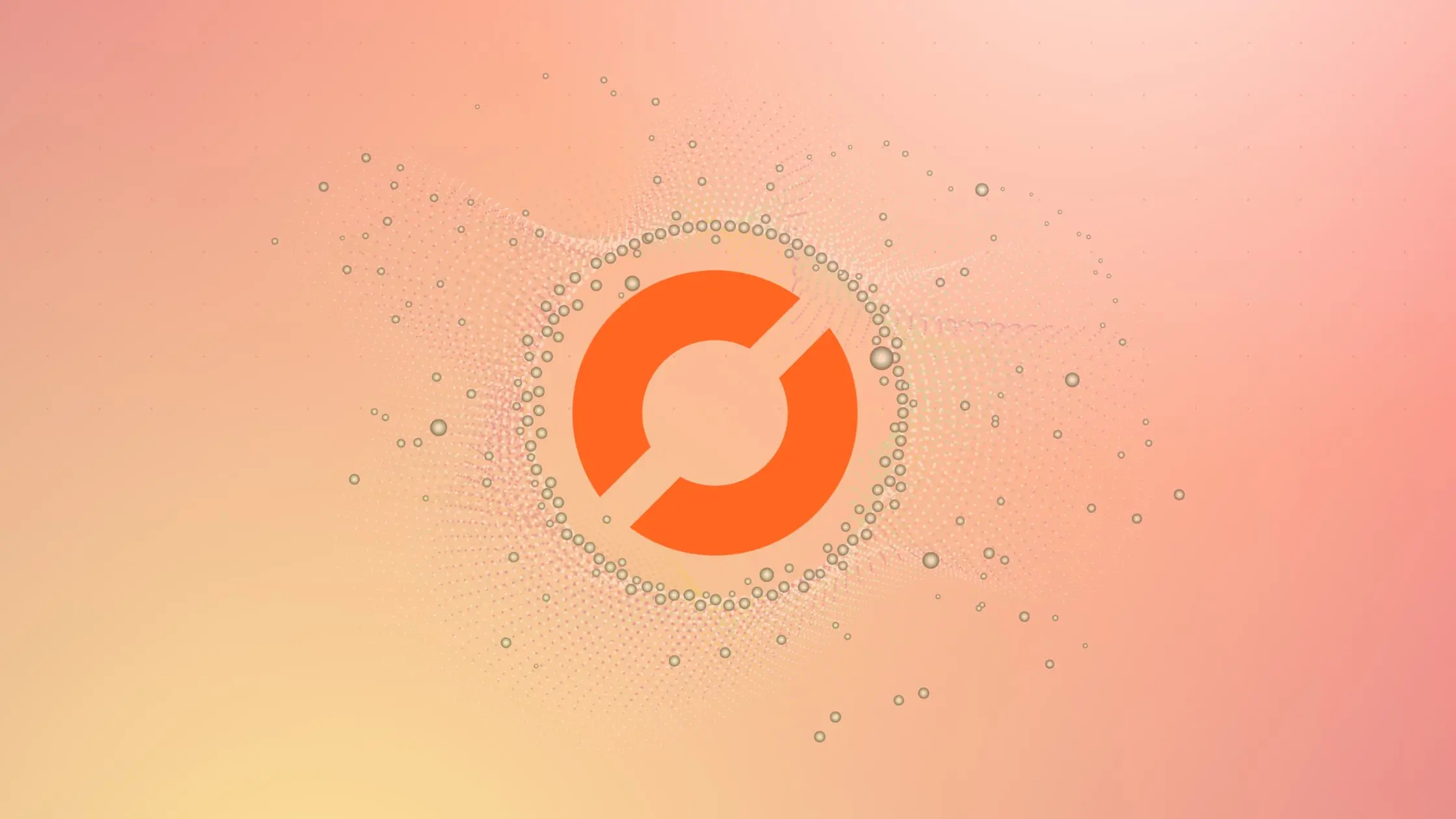
As a data scientist, you may have come across a situation where you need to extract a specific part of a string from a column in a pandas dataframe. For example, you may want to extract the date or time from a timestamp column, or extract a specific part of a string column. In this article, we will explore different techniques to extract substrings from an entire column in a pandas dataframe.
Table of Contents
- What is a Pandas Dataframe?
- Understanding Pandas String Methods
- Extracting Substrings using str.extract()
- Common Errors and Solutions
- Conclusion
What is a Pandas Dataframe?
A pandas dataframe is a two-dimensional table-like data structure that consists of rows and columns. It is a popular data manipulation tool used in data science and machine learning applications. A dataframe can be created from a variety of sources, such as CSV files, Excel spreadsheets, SQL databases, or Python lists.
Understanding Pandas String Methods
Before we dive into the techniques for extracting substrings from a pandas dataframe, it is important to understand pandas string methods. Pandas provides a set of string methods that can be applied to a pandas series or column. These methods include:
str.contains()
: returns a boolean series indicating whether each string contains a specified substring.str.replace()
: replaces a specified substring with another substring.str.split()
: splits each string into a list of substrings based on a specified delimiter.str.extract()
: extracts a substring from each string based on a specified regular expression.str.len()
: returns the length of each string.
In this article, we will focus on the str.extract()
method for extracting substrings from a pandas dataframe.
Extracting Substrings using str.extract()
Let’s take a look at the following examples
import pandas as pd
data = {'Column1': ['abc123', 'def456', 'ghi789']}
df = pd.DataFrame(data)
Method 1: Using the str.extract()
method
The str.extract()
method in Pandas is a powerful tool designed specifically for extracting substrings from DataFrame columns based on regular expressions. Its pros include a clean and efficient syntax tailored for substring extraction, allowing for straightforward implementation of complex patterns. However, it may be less versatile than the apply
function for intricate operations, making it more suitable for tasks where regular expressions suffice.
df['New_Column'] = df['Column1'].str.extract(r'([a-z]+)')
Method 2: Using the apply
function
Utilizing the apply
function offers versatility in substring manipulation, allowing users to define custom functions for more complex operations. This method is advantageous for scenarios where straightforward slicing or basic manipulations are insufficient. However, it may be less efficient for large datasets compared to specialized methods like str.extract()
, and it requires additional coding for custom functions.
def extract_substring(value):
return value[0:3]
df['New_Column'] = df['Column1'].apply(extract_substring)
Method 3: Utilizing regular expressions directly
Directly applying regular expressions using the apply
function provides a robust approach for intricate substring patterns. This method is powerful and flexible, making it suitable for advanced string manipulation tasks. Nonetheless, the syntax may be challenging for beginners, and the use of regular expressions can impact performance on large datasets, making it essential to balance power with efficiency.
import re
df['New_Column'] = df['Column1'].apply(lambda x: re.search(r'([a-z]+)', x).group() if re.search(r'([a-z]+)', x) else None)
Output:
Column1 New_Column
0 abc123 abc
1 def456 def
2 ghi789 ghi
Common Errors and Solutions
Error 1: IndexError
If you encounter an IndexError
, ensure the specified range is within the length of the string when using str.extract()
.
# Before
df['New_Column'] = df['Column1'].str.extract(r'([a-z]+)') # Potential IndexError
# After
df['New_Column'] = df['Column1'].str.extract(r'([a-z]+)') # Ensure the pattern matches the desired substring
Error 2: AttributeError
To avoid an AttributeError
, ensure the column contains only string values when using str.extract()
.
# Before
df['Column1'] = df['Column1'].apply(lambda x: x.upper()) # Potential AttributeError
# After
df['Column1'] = df['Column1'].astype(str).apply(lambda x: x.upper())
Error 3: ValueError
If you encounter a ValueError
, double-check and adjust the regular expression pattern accordingly.
# Before
df['New_Column'] = df['Column1'].apply(lambda x: re.search(r'([a-z]+)', x).group()) # Potential ValueError
# After
df['New_Column'] = df['Column1'].apply(lambda x: re.search(r'([a-zA-Z]+)', x).group() if re.search(r'([a-zA-Z]+)', x) else None)
Conclusion
In this article, we explored different techniques for extracting substrings from an entire column in a pandas dataframe. We focused on using the str.extract()
method to extract substrings based on a specified regular expression. The str.extract()
method is a powerful tool for data manipulation and can be used to extract dates, times, words, or any other substring from a pandas dataframe. By understanding pandas string methods, you can become more proficient in data manipulation and analysis, and be better equipped to solve real-world data science problems.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.