How to Extract Dictionary Values from a Pandas Dataframe
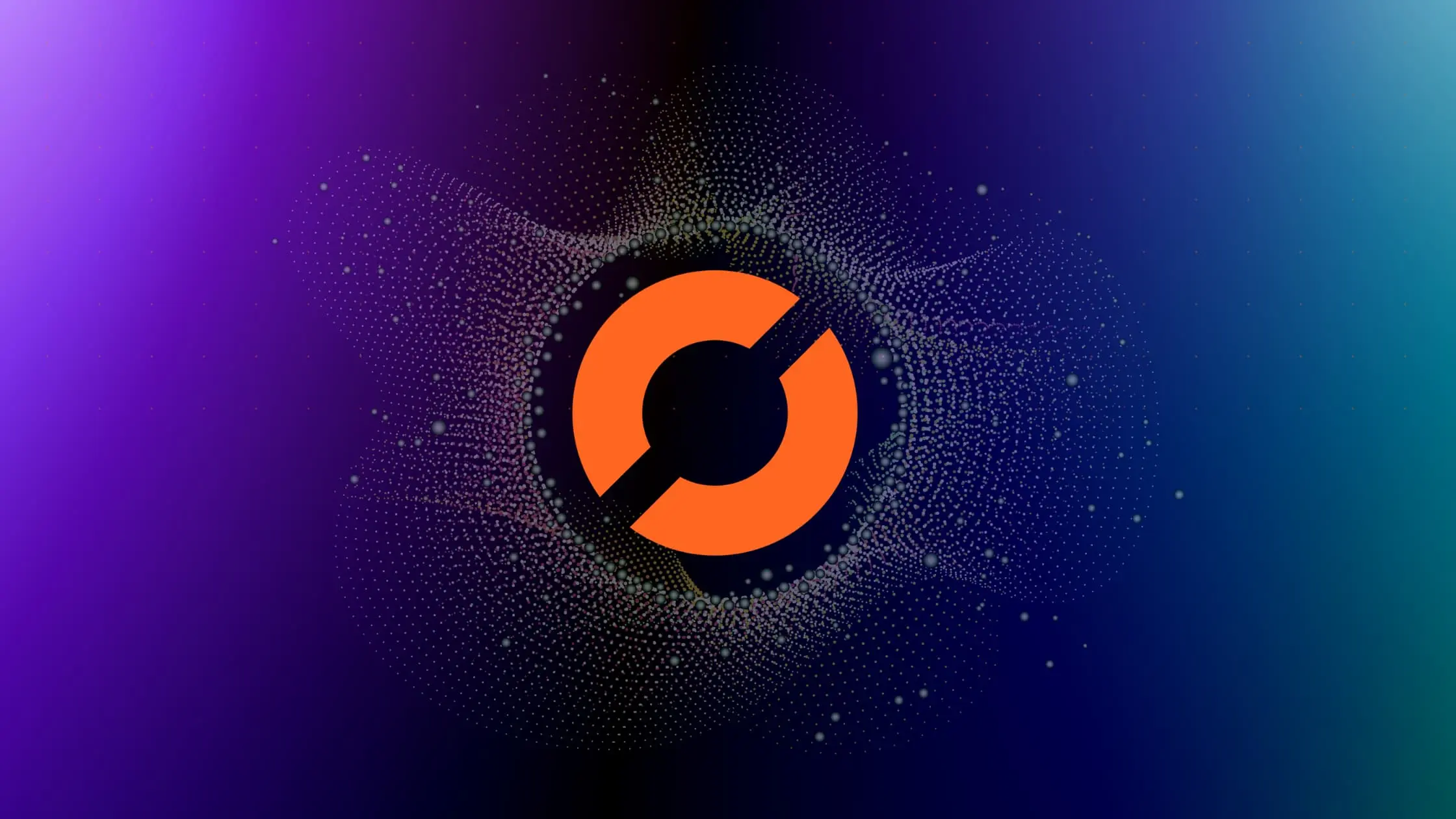
As a data scientist or software engineer, you may have come across a situation where you needed to extract dictionary values from a pandas dataframe. Pandas is one of the most popular data manipulation libraries in Python, and it provides a wide range of functionalities for data analysis. In this article, we will explore how to extract dictionary values from a pandas dataframe in Python, and provide some useful tips to optimize your code.
Table of Contents
Understanding Pandas Dataframe
Before we dive into the extraction of dictionary values, let’s first understand what a pandas dataframe is. A pandas dataframe is a two-dimensional table-like data structure with rows and columns. It is similar to a spreadsheet or SQL table, where each row represents a single observation, and each column represents a variable or feature. In pandas, a dataframe can hold different data types, such as integers, floats, strings, and even dictionaries.
Extracting Dictionary Values
Suppose you have a pandas dataframe with a column containing dictionary values. You may want to extract specific values from the dictionary and store them in a new column or variable. Let’s take a look at an example.
import pandas as pd
data = {'id': [1, 2, 3],
'name': ['Alice', 'Bob', 'Charlie'],
'info': [{'age': 25, 'gender': 'female'},
{'age': 30, 'gender': 'male', 'location': 'New York'},
{'age': 35, 'gender': 'male', 'location': 'San Francisco'}]}
df = pd.DataFrame(data)
print(df)
Output:
id name info
0 1 Alice {'age': 25, 'gender': 'female'}
1 2 Bob {'age': 30, 'gender': 'male', 'location': 'New...
2 3 Charlie {'age': 35, 'gender': 'male', 'location': 'San...
In this example, we have a pandas dataframe with three columns: id
, name
, and info
. The info
column contains dictionaries with different keys and values.
Accessing Dictionary Values
To extract specific values from the dictionary, you can use the .apply()
method in pandas. This method applies a function to each element in a column or row and returns a new column or row with the results.
Let’s say you want to extract the age and gender from the info
column and store them in new columns called age
and gender
. You can define a function that takes a dictionary as an input and returns the age and gender values.
def extract_values(dictionary):
age = dictionary['age']
gender = dictionary['gender']
return age, gender
df[['age', 'gender']] = df['info'].apply(lambda x: pd.Series(extract_values(x)))
print(df)
Output:
id name info age gender
0 1 Alice {'age': 25, 'gender': 'female'} 25 female
1 2 Bob {'age': 30, 'gender': 'male', 'location': 'New... 30 male
2 3 Charlie {'age': 35, 'gender': 'male', 'location': 'San... 35 male
In this example, we define a function called extract_values
that takes a dictionary as an input and returns the age and gender values. We then use the .apply()
method to apply this function to each element in the info
column and return a new dataframe with the results.
Another method is to convert each dictionary into Pandas Serires using apply(pd.Series)
, as shown below:
# Extracting dictionary values from the 'Details' column and creating new columns
df_details = df['info'].apply(lambda x: {} if pd.isna(x) else x).apply(pd.Series)[['age', 'gender']]
# Concatenating the new columns to the original DataFrame
df = pd.concat([df, df_details], axis=1)
print(df)
Output:
id name info age gender
0 1 Alice {'age': 25, 'gender': 'female'} 25 female
1 2 Bob {'age': 30, 'gender': 'male', 'location': 'New... 30 male
2 3 Charlie {'age': 35, 'gender': 'male', 'location': 'San... 35 male
Handling Missing Values
In some cases, the dictionary may not contain a specific key, or it may contain a null value. In such cases, you may want to handle missing values to avoid errors or incorrect results.
Let’s say you want to extract the location
value from the info
column, which may or may not exist in the dictionary. You can modify the extract_values
function to handle missing values using the .get()
method in Python.
def extract_values(dictionary):
age = dictionary['age']
gender = dictionary['gender']
location = dictionary.get('location', None)
return age, gender, location
df[['age', 'gender', 'location']] = df['info'].apply(lambda x: pd.Series(extract_values(x)))
print(df)
Output:
id name info age gender location
0 1 Alice {'age': 25, 'gender': 'female'} 25 female None
1 2 Bob {'age': 30, 'gender': 'male', 'location': 'New... 30 male New York
2 3 Charlie {'age': 35, 'gender': 'male', 'location': 'San... 35 male San Francisco
In this example, we modify the extract_values
function to include a location
variable that uses the .get()
method to return the value of the location
key if it exists, or None
if it does not exist. We then use the .apply()
method to apply this function to each element in the info
column and return a new dataframe with the results.
Error Handling
Nested Dictionaries: If the dictionaries within the dataframe column are nested, additional handling may be required. A more complex extraction function might be necessary to navigate and extract values from nested dictionaries.
Unexpected Data Structures: There might be scenarios where the data is not structured as expected. Adding checks or validation steps to ensure the data conforms to expectations would enhance error handling.
Performance Concerns: As datasets grow, performance becomes critical. Consider profiling the code and optimizing it further for larger datasets if needed.
AttributeError: Occurs if the column doesn’t contain dictionaries, this error may occur if you try to perform dictionary-related operations on a column that doesn’t actually contain dictionaries. For example, if the ‘Details’ column has non-dictionary objects like strings or integers, attempting to apply operations like
apply(lambda x: {} if pd.isna(x) else x)
will result in an AttributeError.
To handle this, it’s crucial to ensure that the ‘Details’ column indeed contains dictionaries before applying any dictionary-specific operations. You can use conditional checks, such as if isinstance(x, dict)
, to verify the type of each element in the column.
- ValueError: Raised when the column has
NaN
values, and proper handling is not applied, when working with dictionaries in Pandas,NaN
(Not a Number) values can pose challenges. If the ‘Details’ column containsNaN
values, applying operations directly on them may result in aValueError
. For instance, attempting to convertNaN
to a dictionary usingapply(lambda x: {} if pd.isna(x) else x)
might trigger this error.
To address this issue, it’s crucial to handle NaN values explicitly. In the provided example, pd.isna(x)
is used within the apply function to replace NaN values with an empty dictionary. This ensures that the subsequent operations on the column are performed on valid dictionary objects.
Conclusion
In this article, we have explored how to extract dictionary values from a pandas dataframe in Python. We have shown how to access specific values from a dictionary using the .apply()
method in pandas, and how to handle missing values using the .get()
method in Python. We hope this article has provided some useful tips to optimize your code and improve your data analysis workflows.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.