How to Extract Column Values Based on Another Column in Pandas
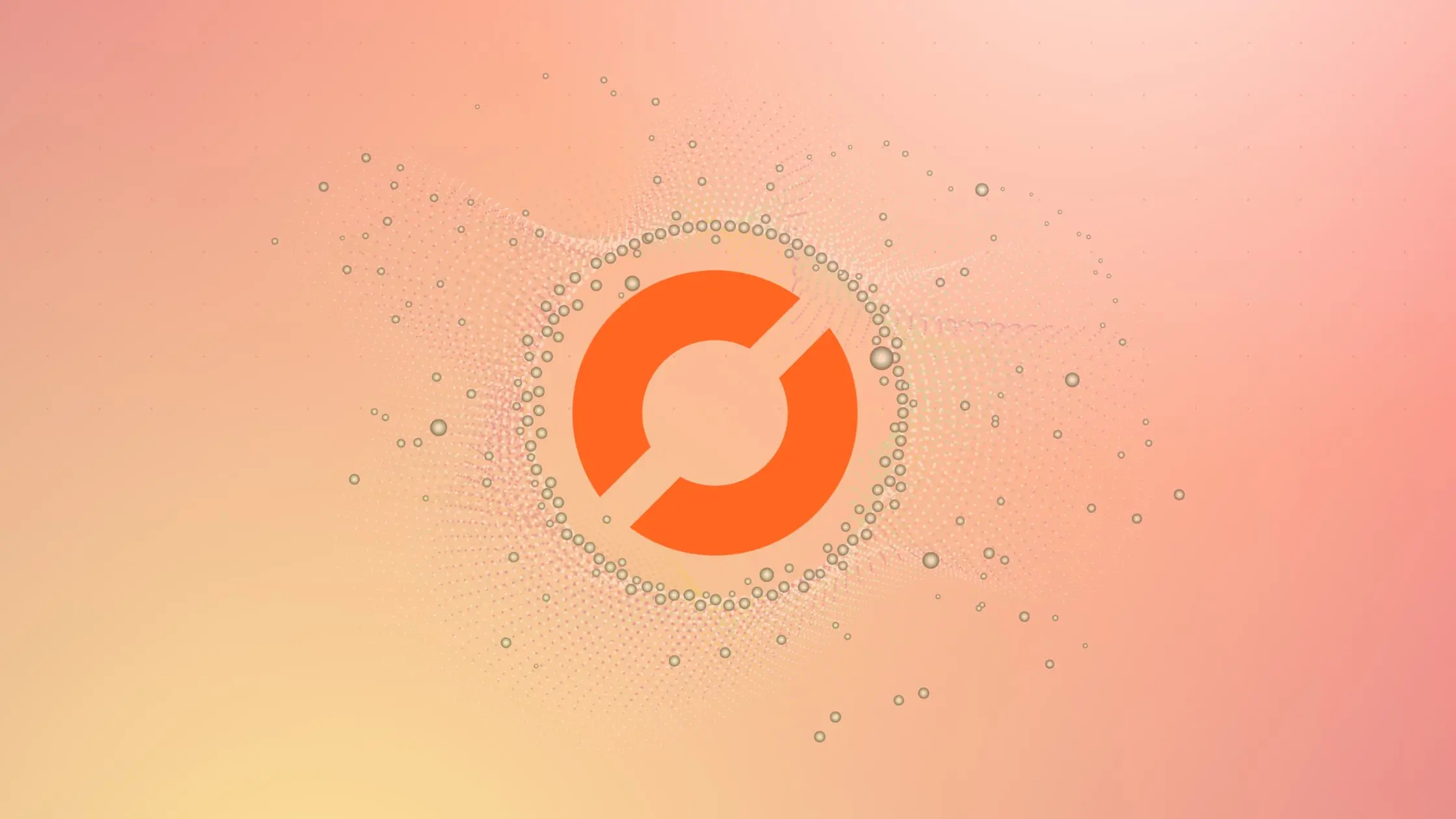
As a data scientist or software engineer, you’re likely familiar with the Pandas library in Python. Pandas is a powerful tool for data manipulation and analysis, and it’s commonly used in data science projects. In this blog post, we’ll explore how to extract column values based on another column in Pandas.
Table of Contents
- Introduction
- The Problem
- The Solution
- Method 1: Using Boolean Indexing
- Method 2: Using the query Method
- Method 3: Using the groupby Method
- Common Errors and Handling
- Syntax Error
- Data Type Error
- Conclusion
The Problem
Suppose you have a dataset with multiple columns, and you want to extract the values of one column based on the values of another column. For example, let’s say you have a dataset of customer orders, and you want to extract the names of customers who have ordered a particular product. How can you do this in Pandas?
The Solution
Pandas provides several ways to extract column values based on another column. Let’s explore some of the most common methods.
Method 1: Using Boolean Indexing
Boolean indexing is a powerful feature of Pandas that allows you to filter a DataFrame based on a condition. To extract column values based on another column, you can use boolean indexing in combination with the .loc
accessor.
Here’s an example:
import pandas as pd
# Create a sample DataFrame
data = {'customer': ['Alice', 'Bob', 'Charlie', 'David'],
'product': ['A', 'B', 'A', 'C']}
df = pd.DataFrame(data)
# Use boolean indexing to extract the names of customers who ordered product A
result = df.loc[df['product'] == 'A', 'customer']
print(result)
Output:
0 Alice
2 Charlie
Name: customer, dtype: object
In this example, we create a DataFrame with two columns: customer
and product
. We then use boolean indexing to extract the names of customers who ordered product A. The condition df['product'] == 'A'
returns a boolean Series that indicates which rows satisfy the condition. We then use the .loc
accessor to select the customer
column for the rows that satisfy the condition.
Method 2: Using the query Method
The query
method is another way to filter a DataFrame based on a condition. It allows you to write a condition as a string, which can be more readable and concise than boolean indexing.
Here’s an example:
import pandas as pd
# Create a sample DataFrame
data = {'customer': ['Alice', 'Bob', 'Charlie', 'David'],
'product': ['A', 'B', 'A', 'C']}
df = pd.DataFrame(data)
# Use the query method to extract the names of customers who ordered product A
result = df.query("product == 'A'")['customer']
print(result)
Output:
0 Alice
2 Charlie
Name: customer, dtype: object
In this example, we use the query
method to extract the names of customers who ordered product A. The string "product == 'A'"
specifies the condition we want to filter on, and we use bracket notation to select the customer
column.
Method 3: Using the groupby Method
The groupby
method is a powerful tool for grouping data based on one or more columns. It can also be used to extract column values based on another column.
Here’s an example:
import pandas as pd
# Create a sample DataFrame
data = {'customer': ['Alice', 'Bob', 'Charlie', 'David'],
'product': ['A', 'B', 'A', 'C']}
df = pd.DataFrame(data)
# Use the groupby method to group the data by product and extract the names of customers who ordered product A
result = df[df['product'] == 'A'].groupby('product')['customer'].apply(list)
print(result)
Output:
product
A [Alice, Charlie]
Name: customer, dtype: object
In this example, the code extracts the names of customers who ordered product ‘A’ from a DataFrame using groupby
and apply(list)
. The output is a Pandas Series that displays this list of names in the form of a table indexed by the product.
Common Errors and Handling
When working with the Pandas library in Python, it’s common to encounter some errors while extracting column values based on another column. Here are some common errors and solutions to resolve them:
1. Syntax Error
If you come across a syntax error using boolean indexing or the query method, ensure that the condition is correctly formulated. Check the syntax and make sure quotes or apostrophes are used appropriately.
# Example of an incorrect condition with missing quotes
result = df.query(product == 'A')['customer'] # Syntax error
- Solution: Surround the condition with single or double quotes, depending on the context.
# Corrected condition with quotes around 'A'
result = df.query("product == 'A'")['customer']
2. Data Type Error
Ensure that the data types of the columns are compatible with the operations you are performing. If an operation cannot be executed due to an incompatible data type, an error will occur.
# Example of a data type error when using groupby
result = df[df['product'] == 'A'].groupby('product')['customer'].apply(list)
# Type error: 'DataFrameGroupBy' object has no attribute 'apply'
- Solution: Ensure that the data types are correct and convert them if necessary.
# Convert the 'customer' column to a list before applying the groupby method
result = df[df['product'] == 'A']['customer'].apply(list)
Conclusion
In this blog post, we’ve explored three different methods for extracting column values based on another column in Pandas. Whether you prefer boolean indexing, the query
method, or the groupby
method, Pandas provides powerful tools for data manipulation and analysis. By using these methods effectively, you can quickly and easily extract the data you need to answer your data science questions.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.