How to Efficiently Compare Rows in a Pandas DataFrame
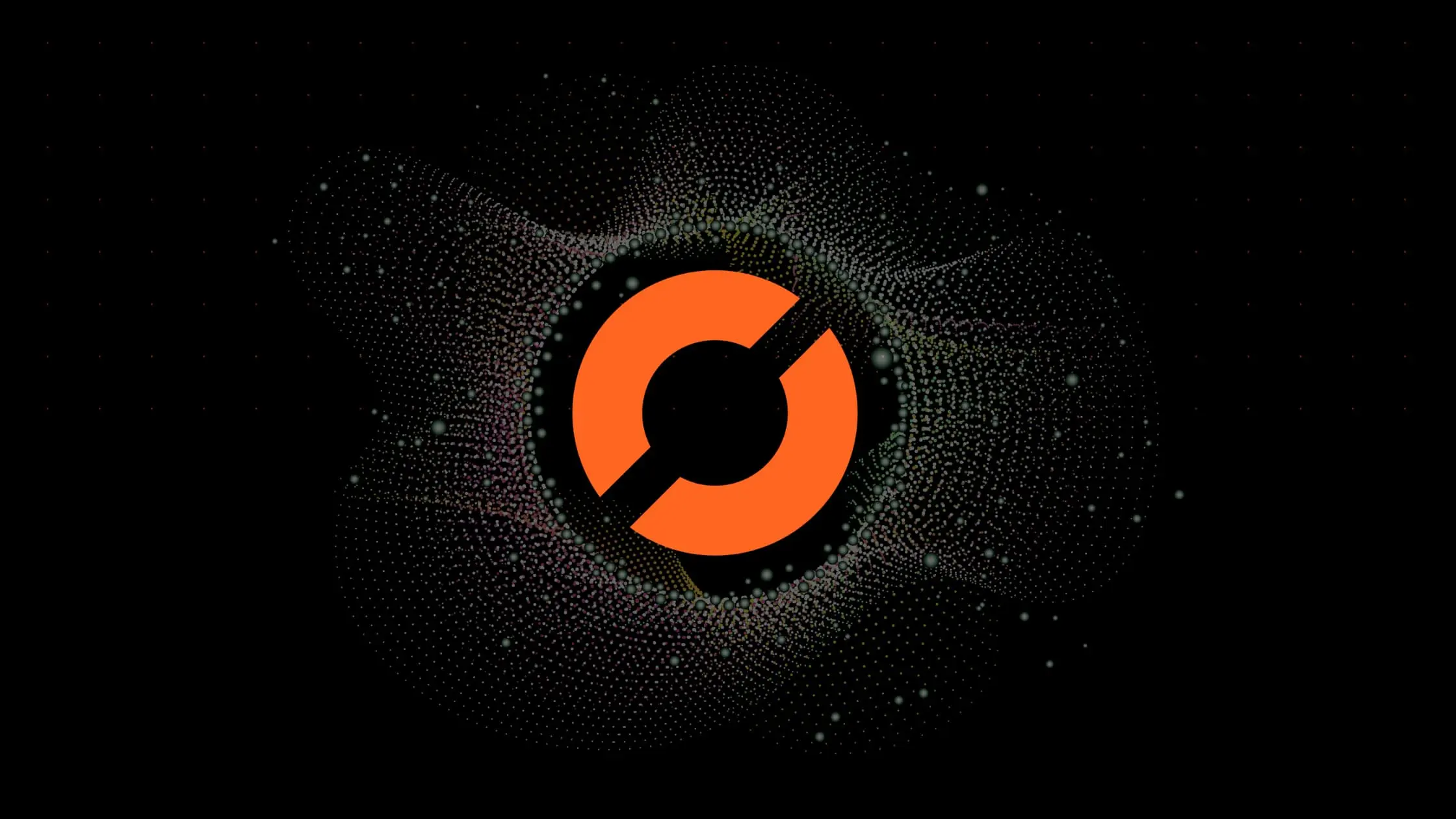
As a data scientist or software engineer, you may come across situations where you need to compare rows in a pandas DataFrame. This can be a challenging task, especially if the DataFrame is large and contains numerous rows. In this blog post, we will discuss how to efficiently compare rows in a pandas DataFrame.
What is a Pandas DataFrame?
A pandas DataFrame is a two-dimensional data structure that is used for data analysis and manipulation. It is similar to a spreadsheet or a database table, where each row represents a record, and each column represents a feature or attribute.
Why Compare Rows in a Pandas DataFrame?
Comparing rows in a pandas DataFrame can be useful for various reasons, such as identifying duplicates, finding anomalies or outliers, or identifying patterns in the data. For example, you may want to compare rows to see if they contain the same values or if they have similar patterns.
How to Compare Rows in a Pandas DataFrame?
There are several ways to compare rows in a pandas DataFrame. In this section, we will discuss some of the most common methods.
Method 1: Using the equals() Method
The equals()
method is a built-in function in pandas that compares two DataFrames and returns a Boolean value indicating whether they are equal or not. To compare rows in a DataFrame, you can create two DataFrames with the rows that you want to compare and use the equals()
method.
import pandas as pd
# Create two DataFrames with the rows to compare
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# Compare the two DataFrames
df1.equals(df2)
This will return True
if the two DataFrames are equal and False
otherwise.
Method 2: Using the isin() Method
The isin()
method is another built-in function in pandas that can be used to compare rows in a DataFrame. It returns a Boolean value indicating whether each element in the DataFrame is contained in a sequence of values.
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# Create a list of values to compare
values = [1, 4, 2, 5]
# Check if each row is in the list of values
df.isin(values)
This will return a DataFrame with True
values where each row is in the list of values and False
otherwise.
Output:
A B
0 True True
1 True True
2 False False
Method 3: Using the apply() Method
The apply()
method can be used to apply a function to each row or column in a DataFrame. You can use this method to create a new column that contains the result of comparing two or more columns.
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [1, 4, 3]})
# Create a new column that compares columns A and C
df['A_C_equal'] = df.apply(lambda row: row['A'] == row['C'], axis=1)
# Create a new column that compares columns A, B, and C
df['A_B_C_equal'] = df.apply(lambda row: row['A'] == row['B'] == row['C'], axis=1)
Output:
A B C A_C_equal
0 1 4 1 True
1 2 5 4 False
2 3 6 3 True
A B C A_C_equal A_B_C_equal
0 1 4 1 True False
1 2 5 4 False False
2 3 6 3 True False
This will create two new columns that contain the result of comparing columns A and C and columns A, B, and C, respectively.
Conclusion
In this blog post, we discussed how to efficiently compare rows in a pandas DataFrame. We covered three methods: using the equals()
method, using the isin()
method, and using the apply()
method. Depending on the specific use case, one method may be more efficient or appropriate than another. By understanding these methods, you can effectively compare rows in a pandas DataFrame and gain insights into your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.