How to Drop Rows with all Zeros in Pandas DataFrame
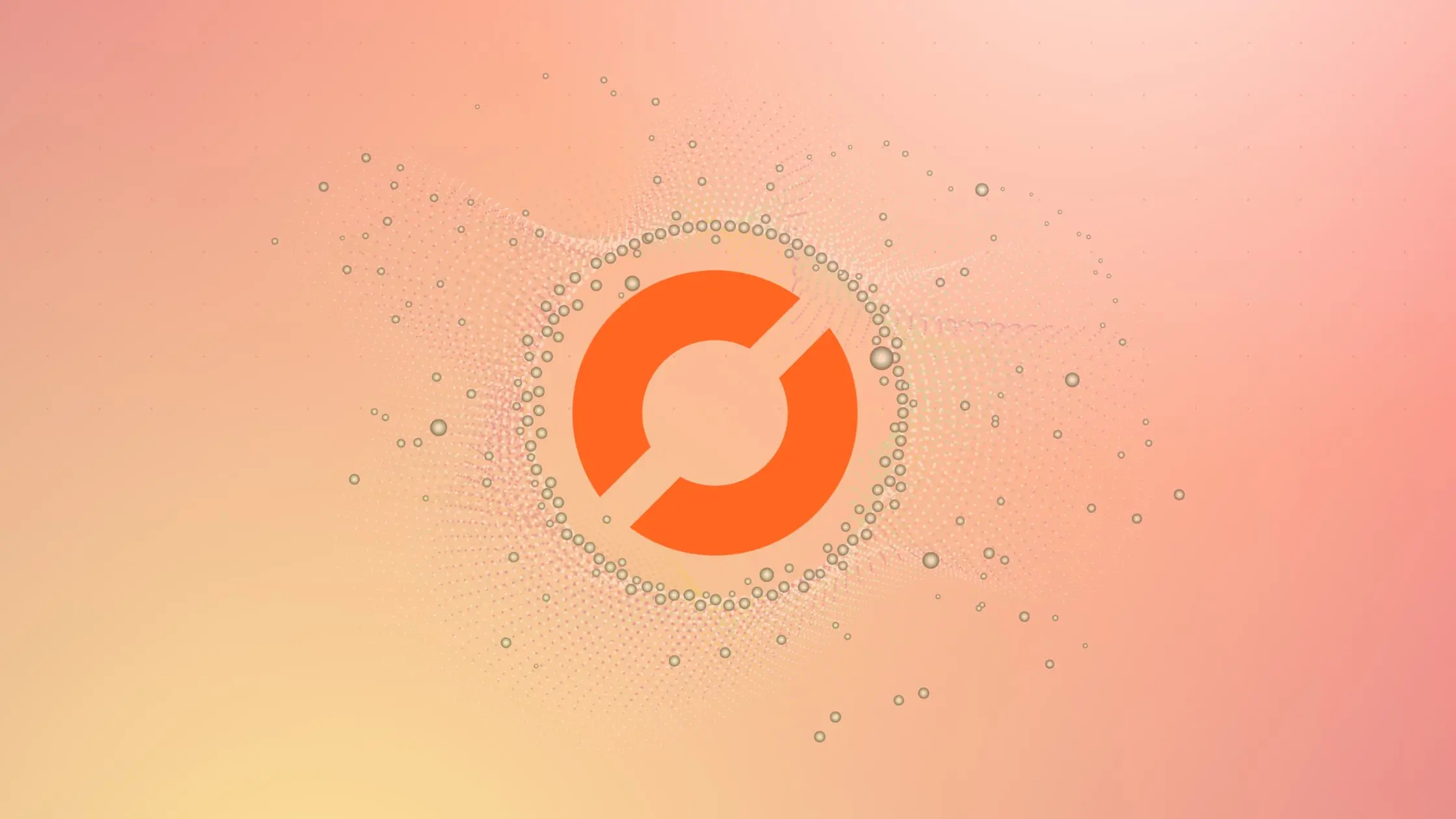
As a data scientist or software engineer, you may come across situations where you need to remove rows from a Pandas DataFrame that contain all zeros. This can be necessary if you are working with large datasets and want to eliminate any rows that do not contain any useful information. In this article, we will explore how to drop rows with all zeros in a Pandas DataFrame.
What is Pandas?
Pandas is an open-source library for data manipulation and analysis in Python. It provides easy-to-use data structures and data analysis tools for handling numerical tables and time series data. Pandas is widely used in data science and machine learning for tasks such as data cleaning, data wrangling, and data visualization.
How to Drop Rows with all Zeros in Pandas DataFrame
To drop rows with all zeros in a Pandas DataFrame, we can use the drop()
method along with the axis
parameter. The axis
parameter specifies whether to drop rows or columns. To drop rows, set axis=0
. To drop columns, set axis=1
.
Here’s an example of how to drop rows with all zeros in a Pandas DataFrame:
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'A': [1, 0, 0, 3, 0], 'B': [0, 0, 0, 0, 0], 'C': [0, 0, 2, 0, 4]})
# drop rows with all zeros
df = df.loc[(df!=0).any(axis=1)]
print(df)
Output:
A B C
0 1 0 0
2 0 0 2
3 3 0 0
4 0 0 4
In this example, we created a sample DataFrame with five rows and three columns. We then used the loc[]
method to select only the rows that contain at least one non-zero value. The (df!=0).any(axis=1)
condition returns a boolean mask with True
for rows that contain at least one non-zero value and False
for rows that contain all zeros.
By using the loc[]
method with the boolean mask, we select only the rows that contain at least one non-zero value and assign the resulting DataFrame back to the variable df
. The resulting DataFrame contains only the rows that contain at least one non-zero value.
Conclusion
Dropping rows with all zeros in a Pandas DataFrame is a common task in data science and machine learning. By using the drop()
method with the axis
parameter, we can easily remove rows that contain all zeros in a Pandas DataFrame. In this article, we demonstrated how to drop rows with all zeros in a Pandas DataFrame using a simple example. We hope this article has been helpful in your data science journey!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.