How to Drop Pandas DataFrame Rows Based on a Condition: A Guide
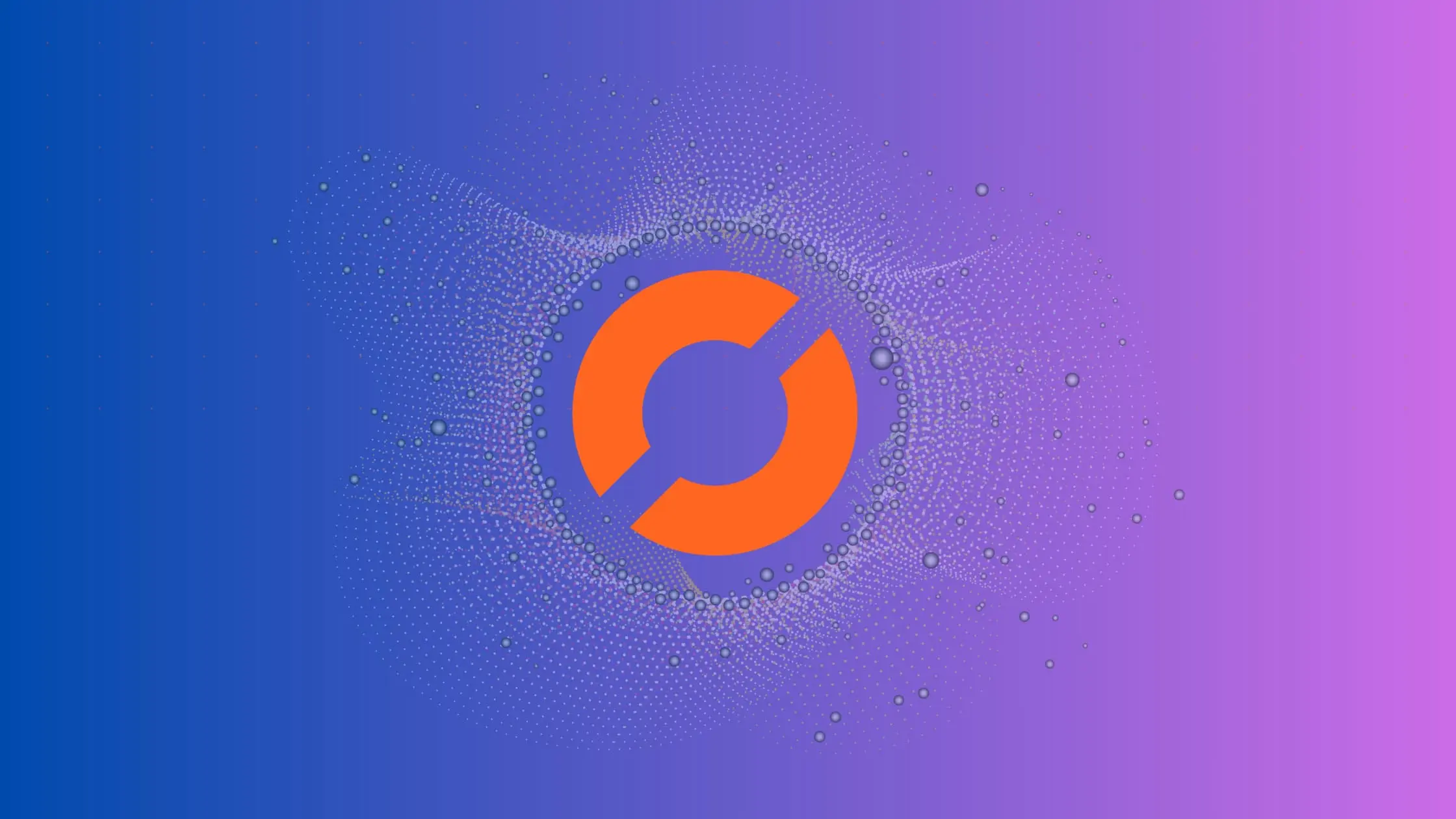
Data manipulation is a crucial part of data science. One of the most common tasks is filtering data based on certain conditions. In this blog post, we’ll explore how to drop rows from a Pandas DataFrame based on a condition. This is an essential skill for any data scientist working with Python and Pandas.
Table of Contents
- What is Pandas?
- Why Drop Rows in a DataFrame?
- Dropping Rows Based on a Single Condition
- Dropping Rows Based on Multiple Conditions
- Comparison of Methods
- Conclusion
What is Pandas?
Pandas is a powerful open-source data analysis and manipulation library for Python. It provides data structures and functions needed to manipulate structured data, including functionality for manipulating and analyzing dataframes.
Why Drop Rows in a DataFrame?
There are many reasons why you might want to drop rows from a DataFrame. You might have missing or incorrect data, outliers that are skewing your analysis, or you might simply want to focus on a subset of your data. Whatever the reason, Pandas provides several methods to help you achieve this.
Dropping Rows Based on a Single Condition
Method 1: Using Boolean Indexing
One of the simplest ways to drop rows is by using boolean indexing. This method involves creating a boolean mask based on the condition and then using it to filter the DataFrame.
import pandas as pd
# Create a DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [25, 30, 22, 35]}
df = pd.DataFrame(data)
# Drop rows where Age is less than 30
df = df[df['Age'] >= 30]
Method 2: DataFrame.query()
The query()
method allows you to filter rows using a query expression, providing a more concise syntax.
# Drop rows where Age is less than 30 using query
df = df.query('Age >= 30')
Method 3: DataFrame.drop()
The drop()
method enables you to remove rows based on index labels or conditions.
# Drop rows where Age is less than 30 using drop
df = df.drop(df[df['Age'] < 30].index)
Method 4: DataFrame.loc[]
Using loc[]
, you can drop rows based on labels and conditions simultaneously.
# Drop rows where Age is less than 30 using loc
df = df.loc[df['Age'] >= 30]
Output:
Name Age
1 Bob 30
3 David 35
Dropping Rows Based on Multiple Conditions
Method 1: Combining Multiple Conditions
import pandas as pd
# Create a DataFrame
data = {'A': [1, 2, 3, 4], 'B': [5, 6, 7, 8]}
df = pd.DataFrame(data)
# Drop rows where column 'A' is greater than 2 and column 'B' is less than 7
df = df[(df['A'] <= 2) & (df['B'] >= 6)]
Method 2: Using the query
Function for Complex Conditions
import pandas as pd
# Create a DataFrame
data = {'A': [1, 2, 3, 4], 'B': [5, 6, 7, 8]}
df = pd.DataFrame(data)
# Drop rows where column 'A' is greater than 2 and column 'B' is less than 7 using query
df = df.query('A <= 2 and B >= 6')
Output:
A B
1 2 6
Comparison of Methods
Let’s compare these methods based on various criteria to help you choose the most suitable one for your needs.
Method | Pros | Cons |
---|---|---|
Boolean Indexing | Simple syntax, intuitive | Creates a new DataFrame |
DataFrame.query() | Concise, supports complex queries | Requires additional quoting in queries |
DataFrame.drop() | Versatile, allows index-based drops | Modifies the original DataFrame |
DataFrame.loc[] | Combines label and condition-based drops | Slightly more verbose syntax |
Conclusion
Dropping Pandas DataFrame rows based on conditions is a common task in data analysis. In this guide, we explored various methods for single and multiple conditions, discussed their pros and cons, and provided examples to illustrate their usage. Choose the method that best fits your specific scenario and be mindful of common errors that may arise during implementation.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.