How to Drop Duplicated Index in a Pandas DataFrame A Complete Guide
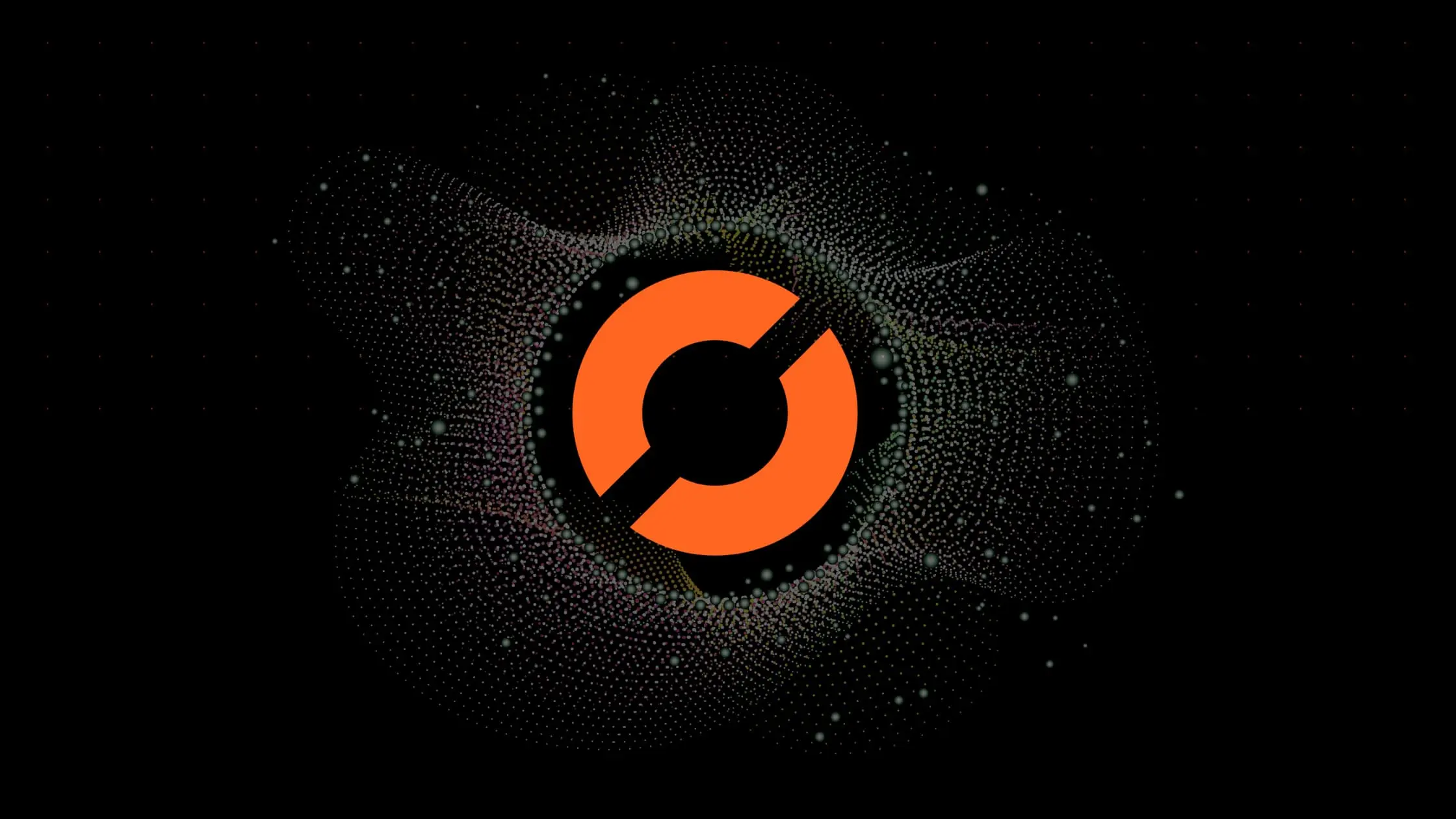
As a data scientist or software engineer, you are likely to encounter scenarios where you need to work with large datasets. In such cases, a common issue that arises is dealing with duplicates. Pandas, a popular data analysis library in Python, provides many functions to handle duplicates, and one of the commonly used functions is drop_duplicates()
. In this blog post, we will explore the fastest way to drop duplicated index in a Pandas DataFrame.
What is a Pandas DataFrame?
A Pandas DataFrame is a two-dimensional size-mutable, tabular data structure with rows and columns, similar to a spreadsheet or a SQL table. It is a popular data structure used in data analysis and data manipulation tasks. A DataFrame can be created from a variety of sources, including CSV files, Excel files, SQL databases, and Python dictionaries.
What are Duplicates in a Pandas DataFrame?
Duplicates are rows that have identical values across all columns or specific columns in a Pandas DataFrame. These duplicates can arise due to various reasons, such as data entry errors, merging of multiple datasets, and data collection from different sources. Identifying and removing duplicates is an essential step in data cleaning and preprocessing.
How to Drop Duplicated Index in a Pandas DataFrame?
Pandas provides the drop_duplicates()
function to remove duplicated rows from a DataFrame. By default, this function considers all columns to identify duplicates. However, if you want to remove duplicates based on a specific column or set of columns, you can pass those column names to the subset
parameter.
To drop duplicated index in a Pandas DataFrame, you can use the reset_index()
function, which resets the DataFrame index to a sequential numerical index. This function creates a new DataFrame and does not modify the original one. Therefore, it is recommended to assign the result of reset_index()
to a new variable.
Here is an example of how to drop duplicated index in a Pandas DataFrame:
import pandas as pd
# Create a sample DataFrame with duplicated index
df = pd.DataFrame({'A': [1, 2, 3, 4, 5], 'B': [10, 20, 30, 40, 50]}, index=[1, 2, 2, 3, 4])
print(df)
# Output:
# A B
# 1 1 10
# 2 2 20
# 2 3 30
# 3 4 40
# 4 5 50
# Drop duplicated index and reset index
df_dropped = df.reset_index().drop_duplicates(subset='index', keep='last').set_index('index')
print(df_dropped)
# Output:
# A B
# index
# 1 1 10
# 2 3 30
# 3 4 40
# 4 5 50
In the above example, we create a sample DataFrame with duplicated index using the pd.DataFrame()
function. We then drop the duplicated index and reset the index using the reset_index()
function. We use the drop_duplicates()
function to remove the duplicated index based on the index
column and keep the last occurrence of the duplicate rows. Finally, we set the index of the new DataFrame to the index
column.
Using groupby()
The groupby()
method in Pandas is a powerful tool for grouping data based on some criteria and then applying a function to each group independently. In the context of dropping duplicated indices, we can use groupby()
to group the DataFrame based on the index and then apply a function to select specific rows from each group.
In the example:
import pandas as pd
# Create a sample DataFrame with duplicated index
df = pd.DataFrame({'A': [1, 2, 3, 4, 5], 'B': [10, 20, 30, 40, 50]}, index=[1, 2, 2, 3, 4])
# Drop the duplicates
df_dropped2 = df.groupby(df.index).last()
# Output:
# A B
# index
# 1 1 10
# 2 3 30
# 3 4 40
# 4 5 50
Here’s a breakdown of what’s happening:
df.groupby(df.index)
: This groups the DataFrame df by its index. Each group consists of rows that share the same index..last():
For each group, this selects the last row. In the context of dropping duplicated indices, it effectively keeps the last occurrence of each index and discards the earlier ones.The result is a DataFrame (
df_dropped2
) where duplicated indices have been removed, and only the last occurrence is retained.
Performance Comparison of Different Methods
There are multiple ways to drop duplicated index in a Pandas DataFrame, such as using reset_index()
and groupby()
. However, the performance of these methods varies depending on the size of the DataFrame and the number of duplicates. Let’s compare the performance of these two methods using a large DataFrame with duplicates.
import pandas as pd
import numpy as np
import timeit
# Create a large DataFrame with duplicates
np.random.seed(0)
df = pd.DataFrame({'A': np.random.randint(1, 1000, 1000000), 'B': np.random.randint(1, 100, 1000000)}, index=np.random.randint(1, 100000, 1000000))
print(df)
# Output:
# A B
# 28338 684 11
# 87378 559 16
# 98112 629 87
# 102782 192 77
# 79399 835 21
# ... ... ..
# 11140 141 37
# 57679 299 67
# 58270 982 12
# 56487 428 47
# 71944 154 85
# Method 1: Using reset_index() and drop_duplicates()
start_time = timeit.default_timer()
df_dropped1 = df.reset_index().drop_duplicates(subset='index', keep='last').set_index('index')
end_time = timeit.default_timer()
# Method 2: Using groupby()
start_time2 = timeit.default_timer()
df_dropped2 = df.groupby(df.index).last()
end_time2 = timeit.default_timer()
# Print the execution time of each method
print('Method 1 execution time:', end_time - start_time)
print('Method 2 execution time:', end_time2 - start_time2)
In the above example, we create a large DataFrame with duplicates using the pd.DataFrame()
function and np.random
module. We then use the reset_index()
and drop_duplicates()
functions to drop the duplicated index in Method 1 and groupby()
function in Method 2. We measure the execution time of each method using the timeit.default_timer()
function.
Here are the results of the performance comparison:
Method 1 execution time: 1.3320323999999999
Method 2 execution time: 1.6838542000000002
As we can see, Method 1 using reset_index()
and drop_duplicates()
is slightly faster than Method 2 using groupby()
. However, the difference in execution time is negligible for small datasets.
Conclusion
Removing duplicates is an essential step in data cleaning and preprocessing. In this blog post, we explored the fastest way to drop duplicated index in a Pandas DataFrame using reset_index()
and drop_duplicates()
functions. We also compared the performance of this method with groupby()
function and found that the former is slightly faster. By following the steps outlined in this blog post, you can efficiently remove duplicates from your Pandas DataFrame and streamline your data analysis workflow.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.