How to Drop Columns with All NaNs in Pandas A Data Scientists Guide
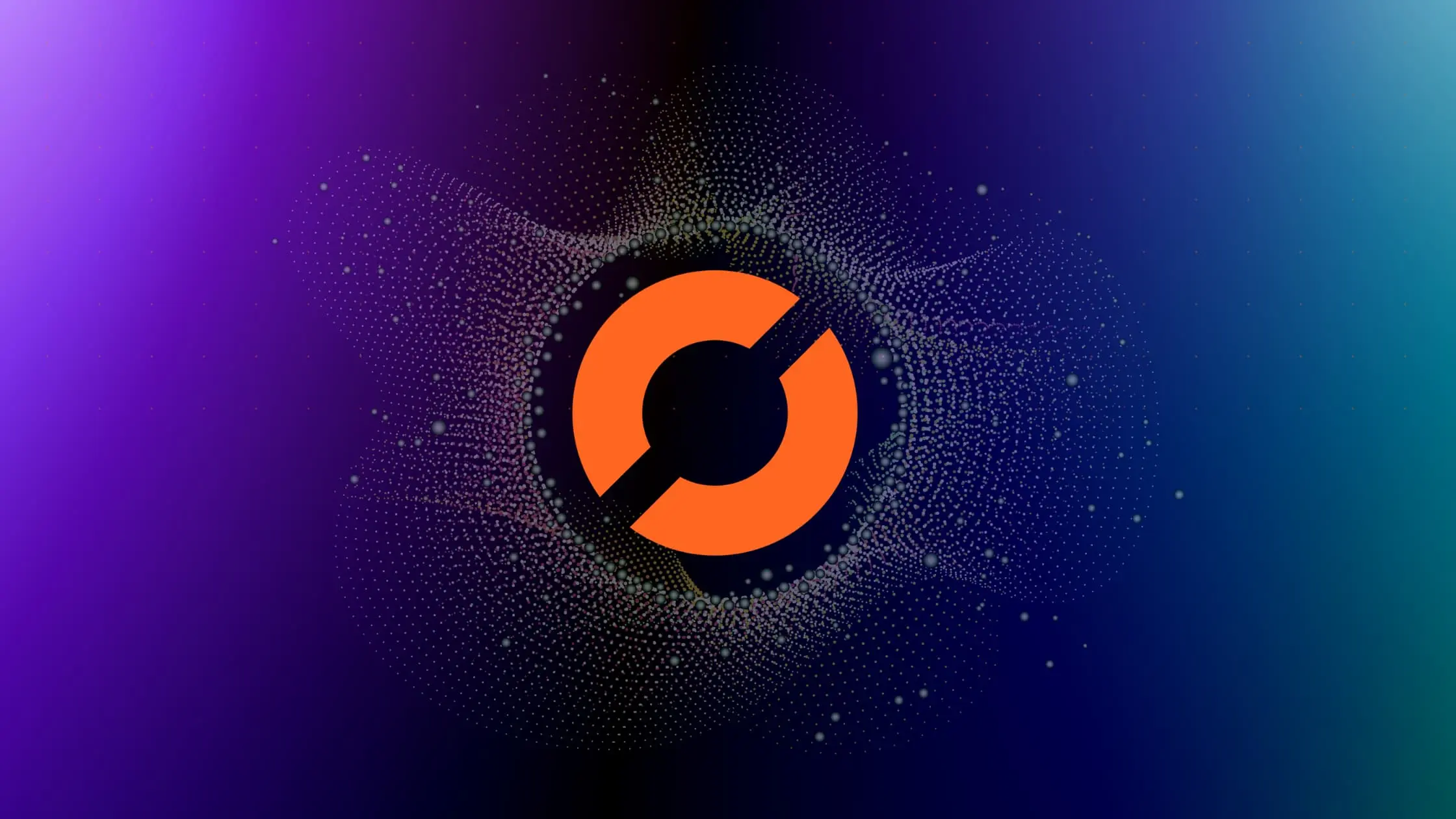
What is Pandas?
Pandas is a popular data analysis library in Python that provides data structures and functions for manipulating numerical tables and time series. It is widely used in data science and machine learning for data preprocessing, cleaning, and analysis. Pandas provides two primary data structures: Series and DataFrame. A Series is a one-dimensional labeled array that can hold any data type, while a DataFrame is a two-dimensional labeled data structure with columns of potentially different types.
How to Drop Columns with All NaN’s
When working with large datasets, it is common to have missing values, represented by NaN or None. These missing values can be problematic for data analysis and modeling since many functions and algorithms cannot handle them. One solution is to drop the rows or columns containing missing values.
To drop columns with all NaN’s in Pandas, we can use the dropna()
function with the axis
parameter set to 1. The axis
parameter specifies whether to drop rows or columns. When set to 1, it drops columns, and when set to 0, it drops rows. Additionally, we can set the how
parameter to 'all'
to drop only columns that contain all NaN’s.
Here is an example:
import numpy as np
import pandas as pd
# create a sample DataFrame with a column containing all NaN's
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [np.nan, np.nan, np.nan]})
# drop columns with all NaN's
df = df.dropna(axis=1, how='all')
# print the resulting DataFrame
print(df)
Output:
A B
0 1 4
1 2 5
2 3 6
In this example, we create a sample DataFrame with three columns, one of which contains all NaN values. We then use the dropna()
function to drop the column with all NaN’s. The resulting DataFrame has only two columns, A and B.
Conclusion
In this article, we have explored how to drop columns with all NaN’s in Pandas. We learned that missing values can be problematic for data analysis and modeling and that dropping the rows or columns containing missing values can be a solution. We also learned how to use the dropna()
function with the axis
and how
parameters to drop columns with all NaN’s.
As a data scientist, it is essential to have a good understanding of data preprocessing techniques like this one. With Pandas, we have a powerful tool for cleaning and transforming data, allowing us to focus on the most important parts of our analysis.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.