How to Divide Multiple Columns by Another Column in Pandas
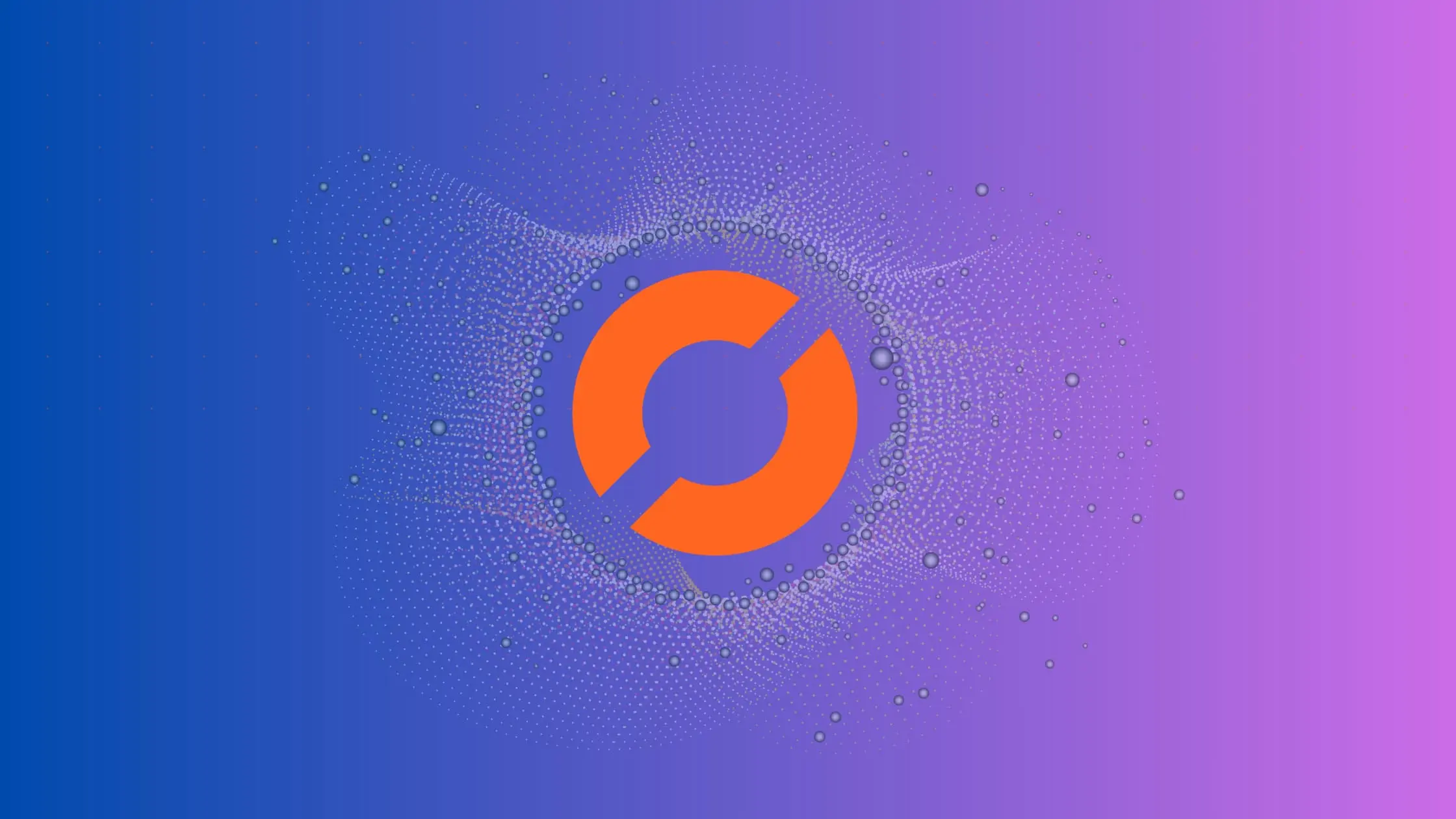
Table of Contents
Introduction to Pandas
Pandas is a popular data manipulation library for Python used extensively in data science and machine learning. It provides powerful tools for data preprocessing, cleaning, and analysis. Pandas is built on top of the NumPy library and provides data structures like DataFrame and Series that make it easy to work with tabular data.
Dividing Multiple Columns by Another Column in Pandas
Let’s start by creating a sample dataset that we can use to demonstrate how to divide multiple columns by another column in pandas.
import pandas as pd
data = {
'A': [1, 2, 3, 4],
'B': [5, 6, 7, 8],
'C': [9, 10, 11, 12],
'D': [13, 14, 15, 16]
}
df = pd.DataFrame(data)
This will create a DataFrame with four columns (A, B, C, and D) and four rows.
A B C D
0 1 5 9 13
1 2 6 10 14
2 3 7 11 15
3 4 8 12 16
Now, let’s say we want to divide columns A, B, and C by column D. We can do this by using either the div()
method or the DataFrame.assign
method in pandas.
Method 1: div()
method
The div()
method handles alignment automatically. Here is how you can use it:
df[['A', 'B', 'C']] = df[['A', 'B', 'C']].div(df['D'], axis=0)
In this code, we select the columns A, B, and C using df[['A', 'B', 'C']]
and divide them by column D using df['D']
. The axis=0
parameter specifies that we want to divide row-wise.
After running this code, our DataFrame will look like this:
A B C D
0 0.076923 0.384615 0.692308 13
1 0.142857 0.428571 0.714286 14
2 0.200000 0.466667 0.733333 15
3 0.250000 0.500000 0.750000 16
As you can see, columns A, B, and C have been divided by column D.
Method 2: df.assign
method
# Create a new DataFrame with divided columns
divided_df = df.assign(**{col: df[col] / df['D'] for col in ['A', 'B', 'C']})
print(divided_df)
This creates a new DataFrame with the columns divided. divided_df
would look like this:
A B C D
0 0.076923 0.384615 0.692308 13
1 0.142857 0.428571 0.714286 14
2 0.200000 0.466667 0.733333 15
3 0.250000 0.500000 0.750000 16
Pros and Cons:
Method 1: div() Method
Pros: Concise and offers a compact and readable way to perform division. Also handles alignment of DataFrames and Series correctly, reducing errors. Lastly does In-Place Modification meaning it changes the original DataFrame, often desirable for efficiency.
Cons: Limited Customization, offers fewer options for specific division scenarios.
Method 2: DataFrame.assign Method
Pros: Allows for custom calculations and transformations within the assignment. Also,can be chained with other methods for efficient DataFrame manipulations. Lastly, preserves original data meaning it creates a new DataFrame, keeping the original intact for reference.
Cons: Can lead to longer code expressions for simple divisions.
Choosing the Right Method:
- Preference for Conciseness and In-Place Modification: Use
div()
. - Need for Flexibility, Chaining, or Preserving Original Data: Use
assign()
. - Significant Customizations: Consider alternatives like list comprehensions for greater control.
Conclusion
Dividing multiple columns by another column in pandas is a simple task that can be accomplished using the div()
method. By following the steps outlined in this post, you should be able to divide multiple columns by another column in pandas with ease. Pandas is a powerful library that provides many tools for data manipulation and analysis, and knowing how to use these tools can save you a lot of time and effort in your data science and machine learning projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.