How to Delete Rows with Null Values in a Specific Column in Pandas DataFrame
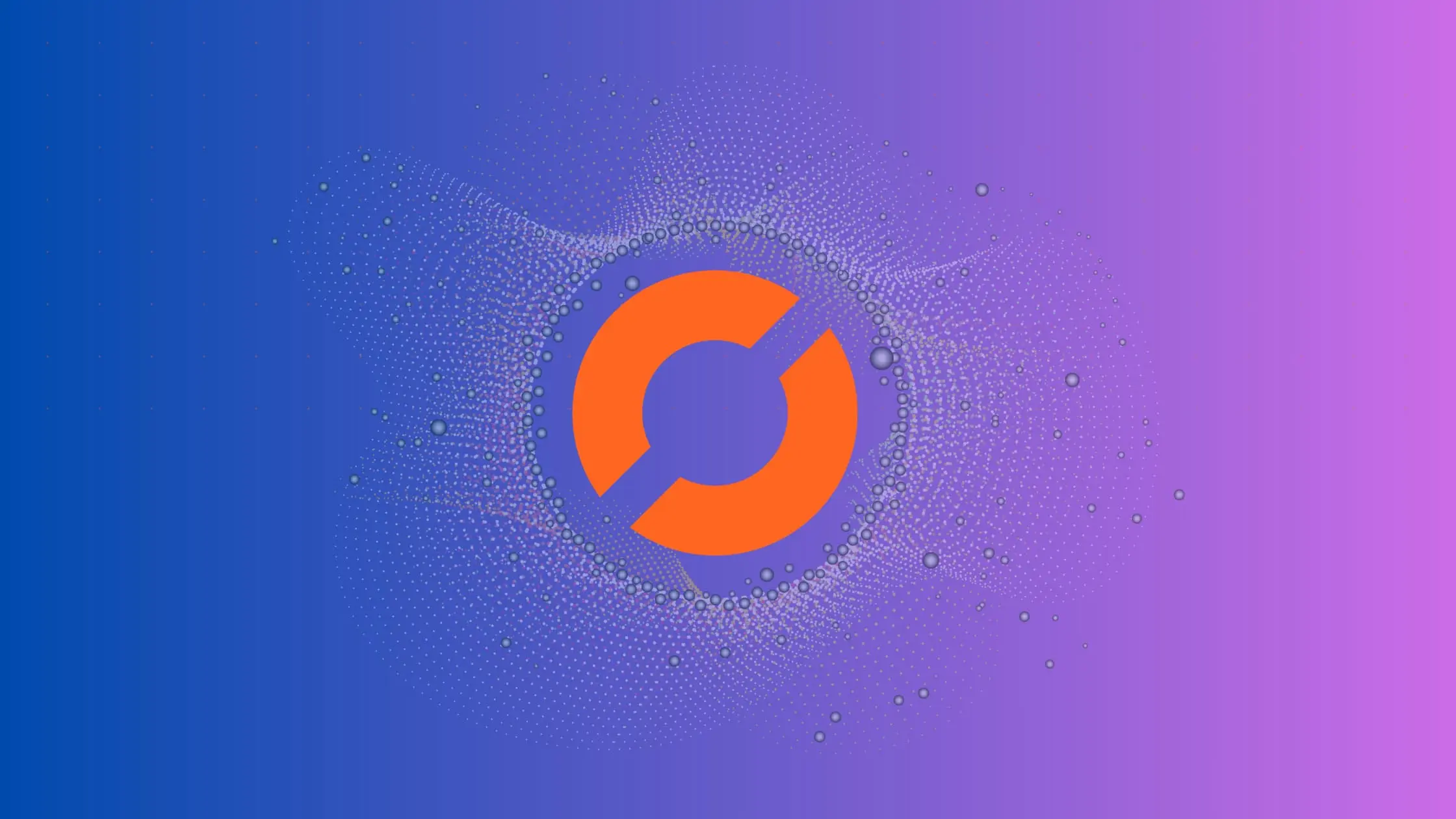
As a data scientist or software engineer, you may encounter datasets that contain null or missing values. These null values can create problems in data analysis, machine learning, and other data-related tasks. One common approach to handle null values is to delete the rows that contain them. In this blog post, we will discuss how to delete rows with null values in a specific column in Pandas DataFrame.
What is Pandas?
Pandas is a popular Python library for data manipulation and analysis. It provides data structures and functions for data cleaning, transformation, and visualization. Pandas DataFrame is a two-dimensional table-like data structure that allows you to store and manipulate data in rows and columns. Pandas DataFrame is widely used in data science and machine learning for data preprocessing and exploratory data analysis.
The Problem of Null Values
Null values, also known as missing values, are values that are not available or unknown in a dataset. Null values can occur due to various reasons such as data entry errors, data corruption, or data collection problems. Null values can create problems in data analysis and modeling because they can affect statistical calculations, data visualization, and machine learning algorithms.
Deleting Rows with Null Values in a Specific Column
One common approach to handle null values is to delete the rows that contain them. Deleting rows with null values in a specific column can be done using the dropna()
method of Pandas DataFrame. The dropna()
method removes all rows that contain null values in the specified column.
Here is the syntax of the dropna()
method:
df.dropna(subset=['column_name'], inplace=True)
df
is the Pandas DataFrame that you want to modify.subset
is the list of column names that you want to check for null values.inplace
is a Boolean value that determines whether to modify the original DataFrame or return a new one. Ifinplace=True
, the original DataFrame is modified, and the method returnsNone
.
Example
Let’s illustrate how to delete rows with null values in a specific column using an example. Suppose we have a Pandas DataFrame that contains information about employees, including their names, ages, and salaries. The DataFrame looks like this:
import pandas as pd
data = {'name': ['Alice', 'Bob', 'Charlie', 'David', 'Eva'],
'age': [25, 30, 35, 40, None],
'salary': [50000, 60000, None, 80000, 90000]}
df = pd.DataFrame(data)
print(df)
name age salary
0 Alice 25.0 50000.0
1 Bob 30.0 60000.0
2 Charlie 35.0 NaN
3 David 40.0 80000.0
4 Eva NaN 90000.0
As you can see, the DataFrame contains null values in the age
and salary
columns. Let’s say we want to delete all rows that contain null values in the salary
column. We can do this using the following code:
df.dropna(subset=['salary'], inplace=True)
print(df)
name age salary
0 Alice 25.0 50000.0
1 Bob 30.0 60000.0
3 David 40.0 80000.0
4 Eva NaN 90000.0
As you can see, the dropna()
method removed the row with a null value in the salary
column.
Handling Null Values in Other Ways
Besides removing rows with null values, you might want to consider other strategies for handling missing data, such as imputation or interpolation. Pandas provides various methods for these purposes, including fillna()
, which allows you to replace null values with specific values or use statistical methods for imputation.
Conclusion
In this blog post, we discussed how to delete rows with null values in a specific column in Pandas DataFrame. We showed how to use the dropna()
method to remove all rows that contain null values in the specified column. Deleting rows with null values can be a useful data cleaning technique to prepare the data for analysis or modeling. However, it is important to be careful when deleting rows because it can affect the representativeness and accuracy of the data. We hope this blog post helps you handle null values effectively in your data analysis and modeling tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.