How to Create an Empty DataFrame with Only Column Names in Pandas
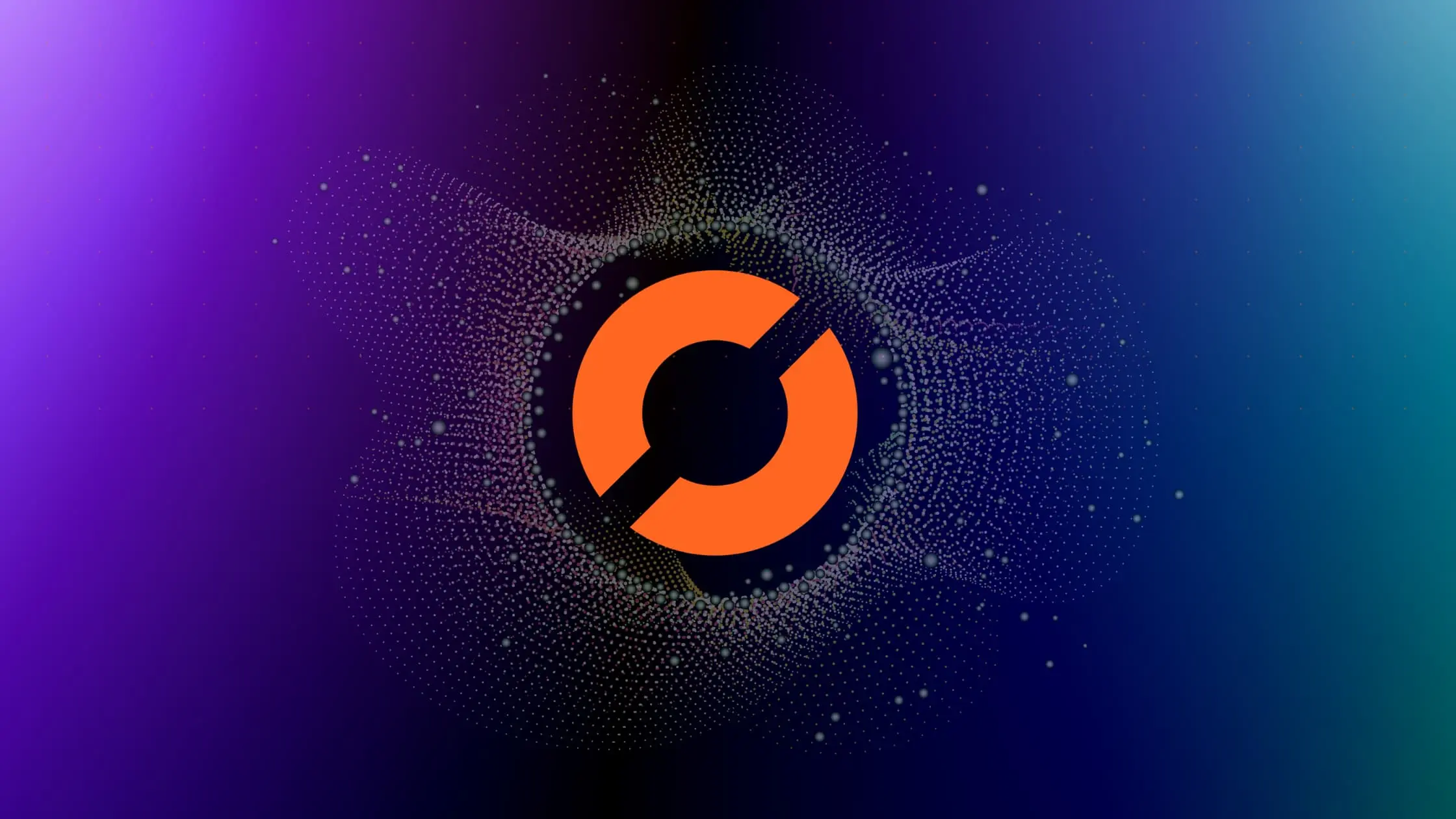
As a data scientist or software engineer, it’s important to know how to create and manipulate data in various formats. One common format is a DataFrame, which is a two-dimensional labeled data structure with columns of potentially different types. In this article, we’ll explore how to create an empty DataFrame with only column names using the Python library, Pandas.
What is Pandas?
Pandas is an open-source data manipulation library for Python that provides fast, flexible, and expressive data structures designed to make working with relational
or labeled
data both easy and intuitive. It is built on top of the NumPy package and its key data structure is a DataFrame, which is a two-dimensional table with columns of potentially different types.
Creating an Empty DataFrame with Only Column Names
Sometimes, you might want to create an empty DataFrame with just the column names, without any data. This can be useful when you want to define the structure of your DataFrame before filling it with data. To create an empty DataFrame with only column names, you can use the pandas.DataFrame()
constructor and specify the column names as a list.
import pandas as pd
# Create an empty DataFrame with column names
df = pd.DataFrame(columns=['Column 1', 'Column 2', 'Column 3'])
print(df)
Output:
Empty DataFrame
Columns: [Column 1, Column 2, Column 3]
Index: []
In the code above, we import the Pandas library and then create an empty DataFrame called df
with the column names Column 1
, Column 2
, and Column 3
. Note that we specify the column names as a list inside the pd.DataFrame()
constructor.
You can also create an empty DataFrame with a specific data type for each column by passing a dictionary of column names and data types to the dtype
parameter. For example, to create an empty DataFrame with two columns, Column 1
and Column 2
, where Column 1
is of type int64
and Column 2
is of type float64
, you can use the following code:
# Create an empty DataFrame with column names and data types
schema={'Column 1': 'int64', 'Column 2': 'float64'}
df = pd.DataFrame(columns=schema.keys()).astype(schema)
print(df.dtypes)
Output:
Column 1 int64
Column 2 float64
dtype: object
In the code above, we first specify the data types for the columns using a dictionary schema
. The keys of the dictionary correspond to the column names, and the values correspond to the data types.
Conclusion
Creating an empty DataFrame with only column names can be useful when you want to define the structure of your DataFrame before filling it with data. In this article, we explored how to create an empty DataFrame with column names using the Pandas library in Python. We also showed how to specify data types for each column when creating an empty DataFrame. With these techniques, you can easily create empty DataFrames with the structure you need for your data analysis or machine learning tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.