How to Create a Single Row Python Pandas DataFrame
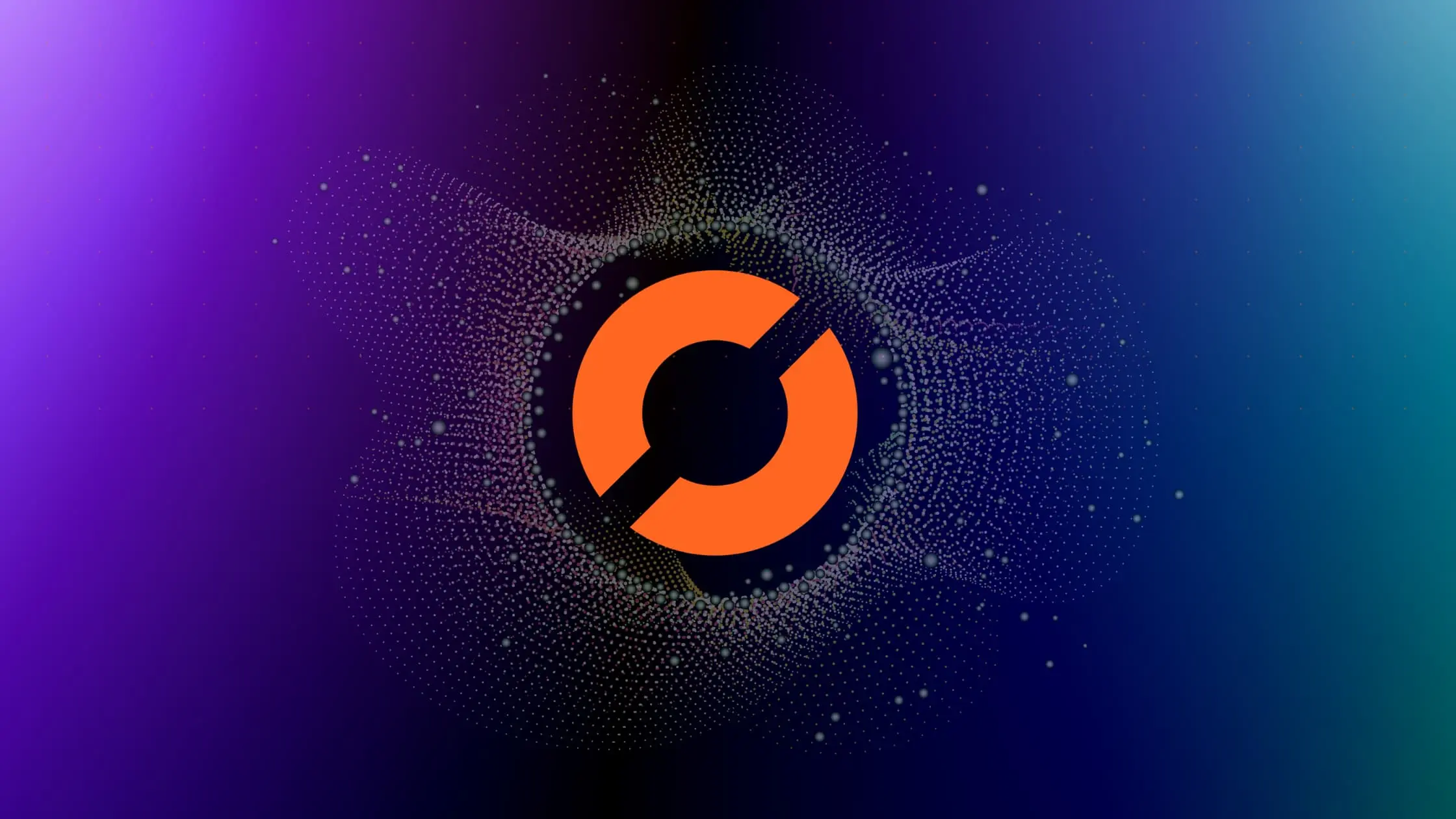
As a data scientist or software engineer, you may often come across scenarios where you need to create a single row Pandas DataFrame in Python. A Pandas DataFrame is a two-dimensional size-mutable, tabular data structure, which can be created using various methods, such as reading data from a CSV file, a database, or by creating a DataFrame from scratch. In this blog post, we will discuss how to create a single row Pandas DataFrame in Python.
Table of Contents
- Introduction
- What is a Pandas DataFrame?
- Creating a Single Row Pandas DataFrame
- 3.1 Using a Dictionary
- 3.2 Using a List
- 3.3 Setting the Index
- Conclusion
What is a Pandas DataFrame?
Before we dive into the details of how to create a single row Pandas DataFrame, let us first understand what a Pandas DataFrame is. A Pandas DataFrame is a two-dimensional size-mutable, tabular data structure with labeled axes (rows and columns). It is similar to a spreadsheet or a SQL table, where data is organized in rows and columns. Each column in a DataFrame can have a different data type, such as integers, floats, strings, etc.
Pandas is a popular Python library used for data manipulation and data analysis. It provides powerful tools for data cleaning, data transformation, and data visualization. Pandas DataFrame is one of the most commonly used data structures in Pandas.
Creating a Single Row Pandas DataFrame
To create a single row Pandas DataFrame, we can use the DataFrame()
constructor provided by the Pandas library. The DataFrame()
constructor takes several arguments, including data, index, and columns.
Let’s start by importing the Pandas library:
import pandas as pd
Using a Dictionary
One of the simplest ways to create a single row Pandas DataFrame is by using a Python dictionary. In this method, we create a dictionary with keys as column names and values as data for the row. We then pass this dictionary as an argument to the DataFrame()
constructor.
Let’s create a dictionary with a single row data:
data = {'Name': ['John'], 'Age': [25], 'Country': ['USA']}
In the above dictionary, we have defined three columns - Name, Age, and Country, with their respective values. Now, let’s create a Pandas DataFrame using this dictionary:
df = pd.DataFrame(data)
This will create a single row Pandas DataFrame with the following output:
Name | Age | Country | |
---|---|---|---|
0 | John | 25 | USA |
Using a List
Another way to create a single row Pandas DataFrame is by using a Python list. In this method, we create a list with a single row data, and then pass this list as an argument to the DataFrame()
constructor.
Let’s create a list with a single row data:
data = [['John', 25, 'USA']]
In the above list, we have defined three columns - Name, Age, and Country, with their respective values. Now, let’s create a Pandas DataFrame using this list:
df = pd.DataFrame(data, columns=['Name', 'Age', 'Country'])
This will create a single row Pandas DataFrame with the following output:
Name | Age | Country | |
---|---|---|---|
0 | John | 25 | USA |
Setting the Index
By default, the DataFrame()
constructor creates a numeric index starting from 0 for each row. However, we can also set a custom index for our single row Pandas DataFrame. We can do this by passing an index
argument to the DataFrame()
constructor.
Let’s create a dictionary with a single row data and a custom index:
data = {'Name': ['John'], 'Age': [25], 'Country': ['USA']}
index = ['Row1']
In the above dictionary, we have defined three columns - Name, Age, and Country, with their respective values. We have also defined a custom index Row1. Now, let’s create a Pandas DataFrame using this dictionary and index:
df = pd.DataFrame(data, index=index)
This will create a single row Pandas DataFrame with the following output:
Name | Age | Country | |
---|---|---|---|
Row1 | John | 25 | USA |
Conclusion
In this blog post, we have discussed how to create a single row Pandas DataFrame in Python. We have seen two methods for creating a single row Pandas DataFrame - using a dictionary and using a list. We have also seen how to set a custom index for our single row Pandas DataFrame.
Pandas DataFrame is a powerful tool for data manipulation and data analysis. With the ability to create a single row Pandas DataFrame, we can easily add or update data in our data structure. I hope this blog post has helped you in creating a single row Pandas DataFrame in Python.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.