How to Correctly Measure the Execution Time of a Cell in Jupyter?
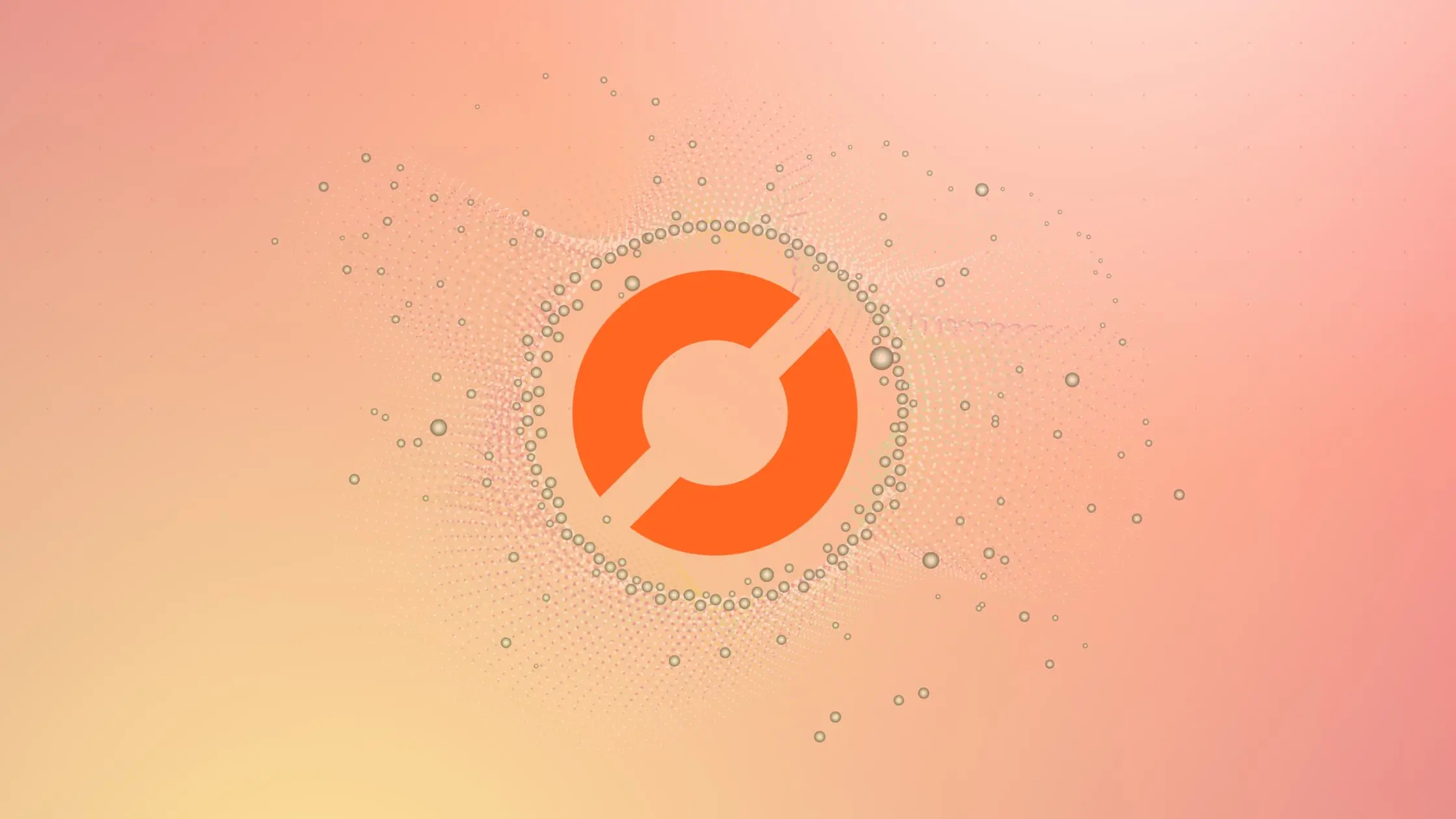
As a data scientist or software engineer, measuring the execution time of code is a crucial part of the development process. In Jupyter notebooks, measuring the execution time of a cell is a common task that helps to optimize code and improve performance. However, measuring execution time is not always straightforward, and there are several factors to consider when doing so. In this post, we will explore how to correctly measure the execution time of a cell in Jupyter notebooks.
Why measure execution time in Jupyter?
The execution time of a cell in Jupyter is the time taken for the code in the cell to run. Measuring execution time is essential for several reasons:
Code optimization: Measuring execution time helps to identify which parts of the code take the most time to execute. By identifying bottlenecks, we can optimize the code and improve performance.
Debugging: Measuring execution time helps to identify which parts of the code are causing performance issues or errors.
Benchmarking: Measuring execution time helps to compare the performance of different algorithms or implementations.
The Problem with Measuring Execution Time in Jupyter
Measuring execution time in Jupyter is not always straightforward. There are several factors to consider:
Cell dependencies: The execution time of a cell can be affected by the execution time of other cells that it depends on. For example, if a cell depends on a cell that takes a long time to execute, the execution time of the dependent cell will also be affected.
External Factors: External factors such as system load, network latency, and disk I/O can affect the execution time of a cell.
Jupyter Notebook Server: The execution time of a cell can be affected by the performance of the Jupyter notebook server.
These factors can make it challenging to accurately measure the execution time of a cell in Jupyter.
How to Measure Execution Time in Jupyter
Despite these challenges, there are several methods to measure the execution time of a cell in Jupyter. Here we will explore some of the most common methods:
Method 1: Using the %time Magic Command
Jupyter provides a %time
magic command that measures the execution time of a single statement. To use this command, simply prefix the code you want to measure with %time
. For example:
%time x = 2 + 2
This command will output the execution time of the statement in seconds.
Output:
CPU times: user 515 µs, sys: 801 µs, total: 1.32 ms
Wall time: 1.45 ms
Method 2: Using the %timeit Magic Command
The %timeit
magic command is similar to %time
, but it runs the statement multiple times and provides a more accurate measurement of the execution time. To use this command, simply prefix the code you want to measure with %timeit
. For example:
%timeit x = 2 + 2
This command will output the average execution time of the statement over multiple runs (100.000.000 in this example).
Output:
5.92 ns ± 0.0569 ns per loop (mean ± std. dev. of 7 runs, 100,000,000 loops each)
Method 3: Using the %%time Cell Magic Command
The %%time
cell magic command measures the execution time of an entire cell. To use this command, simply include the command at the beginning of the cell you want to measure. For example:
%%time
x = 2 + 2
y = x * 2
This command will output the execution time of the entire cell.
Output:
CPU times: user 4 µs, sys: 1 µs, total: 5 µs
Wall time: 9.06 µs
Method 4: Using the time Module
The time
module provides a way to measure the execution time of a block of code in Python. To use this module, simply import it and use the time()
function to record the start and end times of the code block. Subtracting the start time from the end time gives the execution time. For example:
import time
start_time = time.time()
x = 2 + 2
end_time = time.time()
execution_time = end_time - start_time
print(f"Execution time: {execution_time}")
This method provides a more flexible way to measure execution time than the %time
and %timeit
magic commands. However, it requires more code to set up and record the start and end times.
Output:
Execution time: 0.00014090538024902344
Common Errors and Solutions:
1. Incorrect Cell Dependencies:
- Error: If a cell depends on the execution time of another cell, the measured time may be inaccurate, as it doesn’t account for the dependencies' execution time.
- Solution: Ensure that the dependencies are executed and measured appropriately before measuring the target cell’s execution time. Use tools like
%time
or%timeit
individually on each relevant cell.
2. External Factors Impacting Execution Time:
- Error: External factors such as system load, network latency, and disk I/O can introduce variability in execution time.
- Solution: Run the code in an environment with minimal external interference. Consider running experiments multiple times and averaging results to mitigate the impact of external factors. Utilize
%timeit
for more accurate measurements over multiple runs.
3. Jupyter Notebook Server Performance:
- Error: The performance of the Jupyter notebook server can influence the execution time of a cell.
- Solution: Monitor the server’s performance, and if needed, run the code on a dedicated server or use cloud-based platforms like Saturn Cloud. These platforms often provide more consistent performance.
4. Inconsistent Cell Content:
- Error: Cells with varying content or inconsistent code may lead to unreliable execution time measurements.
- Solution: Ensure that the cell content remains consistent during measurements. Avoid changing variables or code structure between measurements to maintain accurate comparisons.
5. Unaccounted Overheads:
- Error: The
%time
and%timeit
commands may not consider certain overheads, leading to inaccurate measurements. - Solution: Consider using the
%prun
command to profile the code and identify potential overheads. This provides a more comprehensive analysis of the code’s performance.
Best Practices:
1. Isolate and Profile Critical Code Sections:
- Best Practice: Identify and isolate critical sections of code for profiling, focusing on the parts that contribute the most to overall execution time.
- Explanation: Profiling specific sections allows for targeted optimization efforts, leading to more efficient code.
2. Use %timeit for Comprehensive Performance Analysis:
- Best Practice: Employ
%timeit
for a more accurate and averaged measurement over multiple runs. - Explanation: Averaging over multiple runs helps to mitigate variability caused by external factors and provides a more reliable estimate of execution time.
3. Consider External Libraries and Parallelization:
- Best Practice: Leverage external libraries or parallelization techniques for computationally intensive tasks.
- Explanation: External libraries often provide optimized implementations, and parallelization can significantly reduce execution time, especially for tasks with parallelizable components.
4. Monitor Resource Utilization:
- Best Practice: Keep track of system resources during code execution to identify potential bottlenecks.
- Explanation: Monitoring CPU, memory, and disk usage helps in understanding and addressing performance issues related to hardware constraints.
5. Document and Version Control:
- Best Practice: Document code changes that impact performance and use version control systems.
- Explanation: Tracking changes helps in understanding the evolution of code performance over time and facilitates reverting to previous versions if necessary.
Conclusion
Measuring the execution time of a cell in Jupyter is an essential part of the development process. By identifying bottlenecks and performance issues, we can optimize code and improve performance. However, measuring execution time is not always straightforward in Jupyter, and there are several factors to consider. By using the methods outlined in this post, we can measure execution time accurately and effectively in Jupyter notebooks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.