How to Copy a Row from One Pandas DataFrame to Another Pandas DataFrame
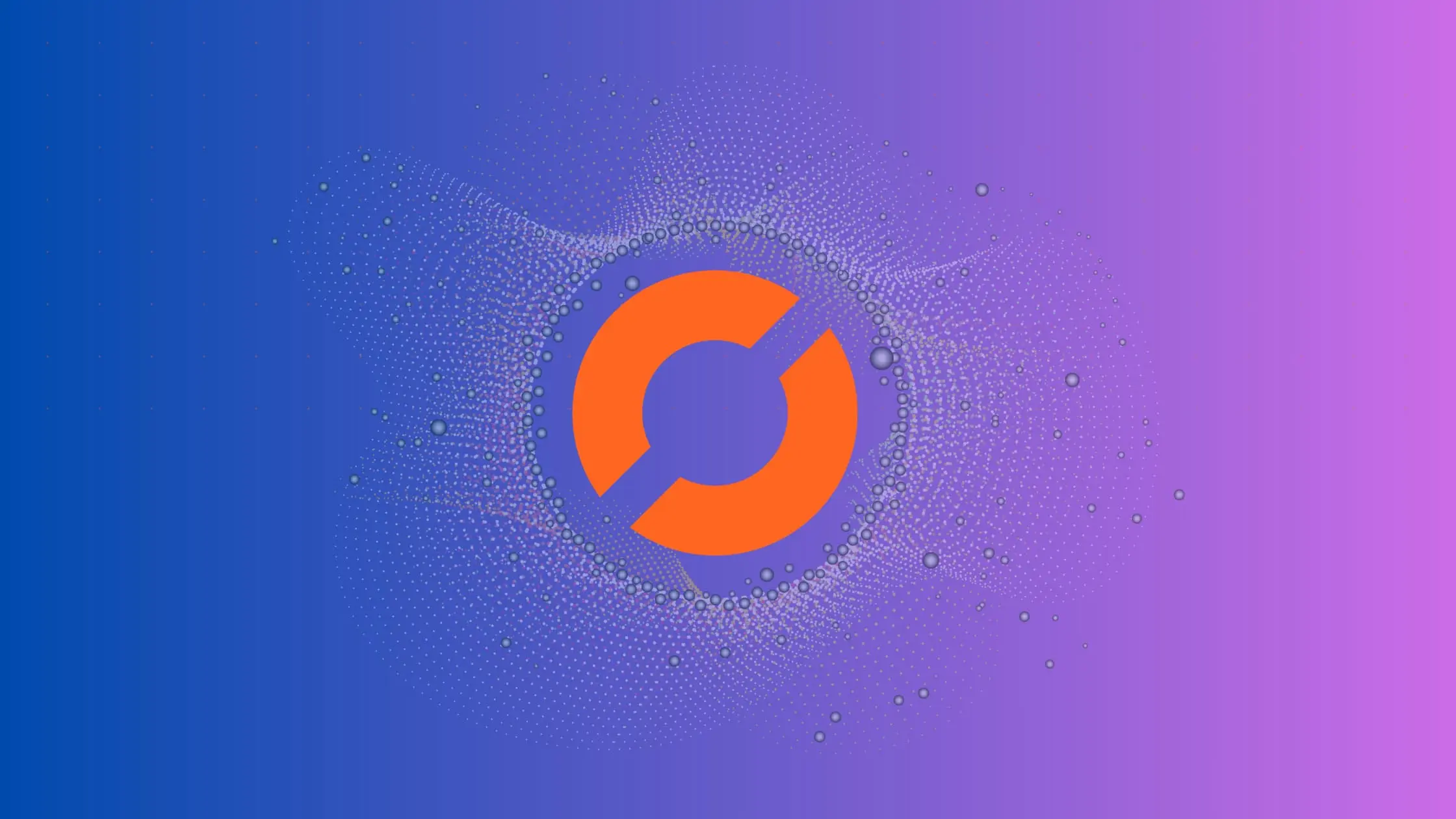
As a data scientist or software engineer, you may often find yourself working with pandas dataframes. Pandas is a popular Python library used for data manipulation and analysis. In this article, we will discuss how to copy a row from one pandas dataframe to another pandas dataframe.
Table of Contents
- Introduction
- What is a Pandas DataFrame?
- Copying a Row from One Pandas DataFrame to Another Pandas DataFrame
- Considerations
- Error Handling
- Conclusion
What is a Pandas DataFrame?
A pandas dataframe is a two-dimensional table-like data structure. It consists of rows and columns, where each row represents an observation and each column represents a variable. The rows and columns are labeled, allowing for easy indexing and manipulation of data.
Copying a Row from One Pandas DataFrame to Another Pandas DataFrame
There are several ways to copy a row from one pandas dataframe to another pandas dataframe. In this section, we will discuss three methods.
Method 1: Using the loc method
The loc
method is used to access a group of rows and columns by label(s) or a boolean array. We can use the loc
method to copy a row from one pandas dataframe to another pandas dataframe.
import pandas as pd
# Create a pandas dataframe
df1 = pd.DataFrame({'Name': ['John', 'Emily', 'Michael'], 'Age': [25, 30, 35]})
# Create an empty pandas dataframe
df2 = pd.DataFrame(columns=['Name', 'Age'])
# Copy a row from df1 to df2 using loc method
df2.loc[0] = df1.loc[0]
print(df2)
Output:
Name Age
0 John 25
In this example, we are creating two pandas dataframes, df1
and df2
. df1
contains three rows and two columns, and df2
is an empty dataframe with two columns. We are then copying the first row from df1
to df2
using the loc
method.
Pros
Label-based indexing: The
loc
method allows for label-based indexing, making it easy to reference rows based on their labels or boolean arrays.Readable code: The code using
loc
is generally more readable, as it explicitly mentions the labels being used for copying rows.
Cons
Potential for confusion: If the dataframe indices are not well-defined or if there are duplicate labels, it may lead to confusion and unintended results.
Limited to labels: The method relies on labels, which might be limiting if you need to copy rows based on integer positions or other criteria.
Method 2: Using the iloc method
The iloc
method is used to access a group of rows and columns by integer position. We can use the iloc
method to copy a row from one pandas dataframe to another pandas dataframe.
import pandas as pd
# Create a pandas dataframe
df1 = pd.DataFrame({'Name': ['John', 'Emily', 'Michael'], 'Age': [25, 30, 35]})
# Create an empty pandas dataframe
df2 = pd.DataFrame(columns=['Name', 'Age'])
# Copy a row from df1 to df2 using iloc method
df2.loc[0] = df1.iloc[0]
print(df2)
Output:
Name Age
0 John 25
In this example, we are creating two pandas dataframes, df1
and df2
. df1
contains three rows and two columns, and df2
is an empty dataframe with two columns. We are then copying the first row from df1
to df2
using the iloc
method.
Pros
Integer-based indexing: The
iloc
method allows for integer-based indexing, providing flexibility when copying rows based on their integer positions.Simple syntax: The code is straightforward and easy to understand, especially when copying rows by specifying integer positions.
Cons
Less readable code: The use of integer positions may be less intuitive, especially for someone unfamiliar with the dataframe structure.
Potential for off-by-one errors: If not careful, using integer positions can lead to off-by-one errors, as indexing starts from 0.
Method 3: Using the append method
The append
method is used to append rows of one dataframe to another. We can use the append
method to copy a row from one pandas dataframe to another pandas dataframe.
import pandas as pd
# Create a pandas dataframe
df1 = pd.DataFrame({'Name': ['John', 'Emily', 'Michael'], 'Age': [25, 30, 35]})
# Create an empty pandas dataframe
df2 = pd.DataFrame(columns=['Name', 'Age'])
# Copy a row from df1 to df2 using append method
df2 = df2._append(df1.iloc[0], ignore_index=True)
print(df2)
Output:
Name Age
0 John 25
In this example, we are creating two pandas dataframes, df1
and df2
. df1
contains three rows and two columns, and df2
is an empty dataframe with two columns. We are then copying the first row from df1
to df2
using the append
method.
Pros
Appending rows: The
append
method is specifically designed for appending rows from one dataframe to another, providing a concise and explicit way to achieve the task.Flexible and simple: The method is flexible and simple, making it easy to understand and use in various scenarios.
Cons
Inefficient for large datasets: The
append
method creates a new dataframe each time it is called, which can be inefficient for large datasets as it involves copying the entire content.Potential for index issues: If the original dataframes have conflicting indices, the
append
method might not handle them seamlessly, leading to unexpected results.
Considerations
The choice of method depends on the specific requirements and preferences of the user.
If the focus is on readability and label-based indexing, the
loc
method may be preferable.If integer positions are more relevant or if simplicity is a priority, the
iloc
method could be a good choice.The
append
method is suitable when the goal is to explicitly append rows, especially in scenarios where efficiency is not a critical concern.
Error Handling
Label Mismatch: When using the
loc
method, if the specified label for copying a row does not exist in the source dataframe, it can result in a KeyError. Implement a mechanism to check the existence of the label before attempting to copy, and handle the KeyError appropriately.Index Out of Range: In the
iloc
method, specifying an index that is outside the valid range of the dataframe can lead to an IndexError. Ensure that the index provided is within the valid range of the dataframe to avoid IndexError. Implement boundary checks as needed.Incompatible Data Types: When appending rows using the
append
method, if the data types of columns in the source and destination dataframes are incompatible, it may result in a TypeError. Verify and handle data type compatibility before attempting to append rows. Convert data types if necessary.Duplicate Index: If the
append
method is used, and the resulting dataframe has duplicate indices, it may lead to unexpected behavior during subsequent operations. Implement checks to avoid appending rows that would result in duplicate indices. Consider resetting indices or using theignore_index
parameter to handle this situation.Insufficient Columns: If the number of columns in the source and destination dataframes is not the same during an append operation, a ValueError may occur. Ensure that the number and order of columns match between the source and destination dataframes. Handle the ValueError by adjusting the column structure accordingly.
Memory Overflow: When appending rows using the
append
method on large datasets, it may lead to memory overflow issues due to the creation of a new dataframe for each append operation. Consider alternative approaches or optimizations for appending rows in chunks to mitigate memory-related issues.Data Integrity Checks: Errors may occur if the copied rows introduce inconsistencies or violate data integrity constraints in the destination dataframe. Implement data integrity checks to ensure that the copied rows adhere to the structure and constraints of the destination dataframe. Handle inconsistencies appropriately.
Conclusion
In this article, we discussed how to copy a row from one pandas dataframe to another pandas dataframe. We explored three methods: using the loc
method, using the iloc
method, and using the append
method. These methods provide different ways to manipulate data in pandas dataframes and can be used in different scenarios. Understanding how to copy rows from one pandas dataframe to another is an important skill for any data scientist or software engineer working with pandas dataframes.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.