How to Copy a Pandas DataFrame Row to Multiple Other Rows
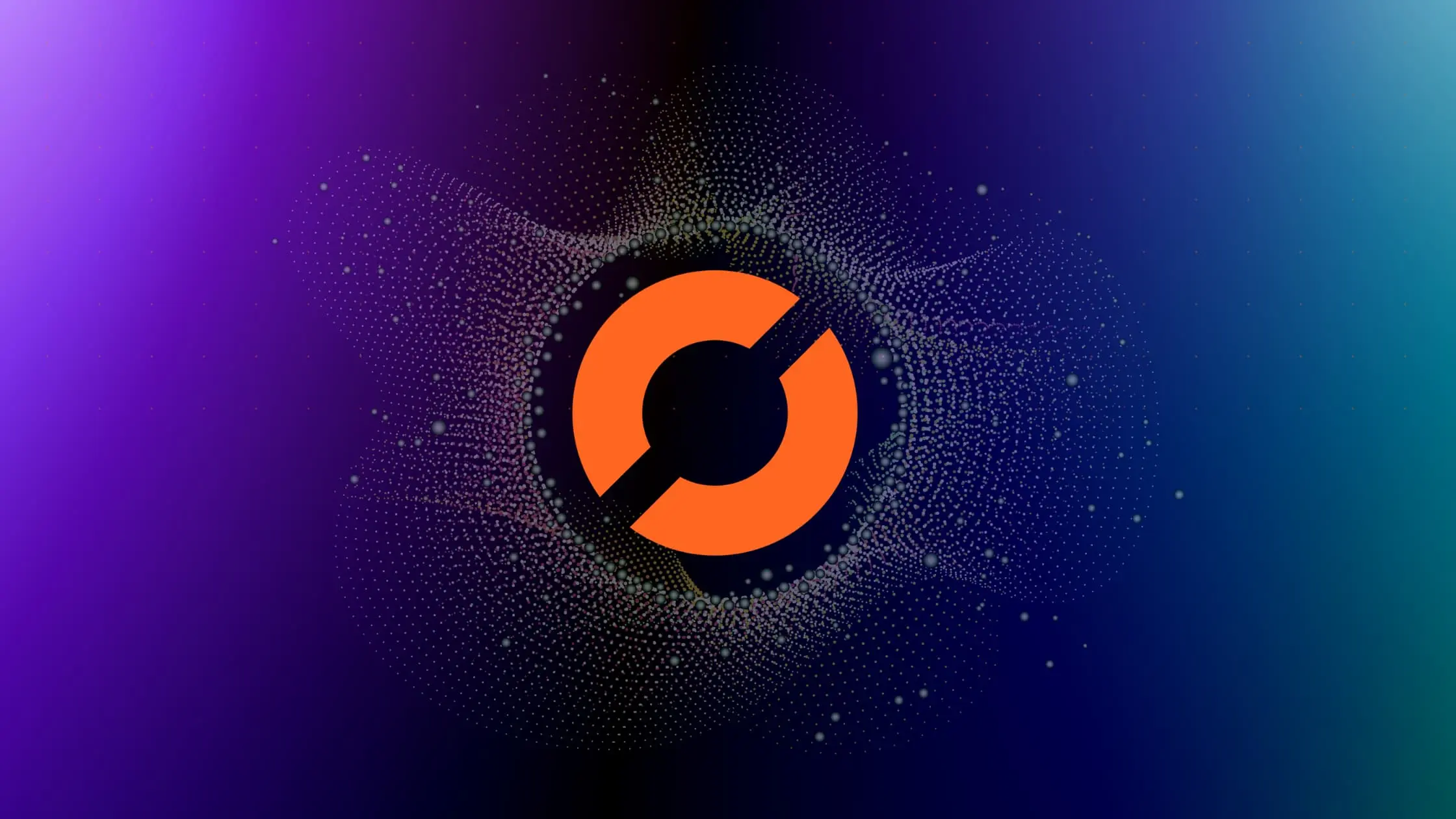
As a data scientist or software engineer, you may often encounter the need to copy a row from a pandas DataFrame to multiple other rows. This can be useful when you need to replicate a certain set of values across multiple rows. In this article, we will explore how to copy a pandas DataFrame row to multiple other rows.
Table of Contents
What is a Pandas DataFrame?
Before we dive into the specifics of copying DataFrame rows, let’s first understand what a pandas DataFrame is. A DataFrame is a two-dimensional table-like data structure that consists of rows and columns. It is used for data manipulation and analysis in Python. A DataFrame can be thought of as a spreadsheet or a SQL table.
How to Copy a Pandas DataFrame Row to Another Row
Copying a pandas DataFrame row to another row is a common operation that can be easily achieved using the copy()
method. The copy()
method creates a new copy of the DataFrame, which we can then manipulate as needed.
Here is an example of how to copy a pandas DataFrame row to another row:
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({'Name': ['John', 'Jane', 'Bob', 'Mary'],
'Age': [25, 30, 45, 35],
'Country': ['USA', 'Canada', 'UK', 'Australia']})
# Copy the second row to the fifth row
df.loc[4] = df.loc[1].copy()
print(df)
Output:
Name Age Country
0 John 25 USA
1 Jane 30 Canada
2 Bob 45 UK
3 Mary 35 Australia
4 Jane 30 Canada
In this example, we created a sample DataFrame with four rows and three columns. We then used the loc[]
method to select the second row and the copy()
method to create a new copy of the row. Finally, we assigned the new copy to the fifth row using df.loc[4]
.
How to Copy a Pandas DataFrame Row to Multiple Other Rows
Copying a pandas DataFrame row to multiple other rows can be achieved using a combination of the copy()
and loc[]
methods. The copy()
method creates a new copy of the row, and the df._append()
method allows us to copy multiple rows in the DataFrame.
Here is an example of how to copy a pandas DataFrame row to multiple other rows:
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({'Name': ['John', 'Jane', 'Bob', 'Mary'],
'Age': [25, 30, 45, 35],
'Country': ['USA', 'Canada', 'UK', 'Australia']})
# Use copy() to duplicate the second row
new_row = df.loc[1].copy()
# Append the new row twice to the original DataFrame
df = df._append([new_row] * 2, ignore_index=True)
print(df)
Output:
Name Age Country
0 John 25 USA
1 Jane 30 Canada
2 Bob 45 UK
3 Mary 35 Australia
4 Jane 30 Canada
5 Jane 30 Canada
In this example, we used the loc[]
method to select the the fifth and sixth rows and assigned them the second row using df._append([new_row] * 2, ignore_index=True)
.
Using numpy to Replicate Rows
Another approach to copy a pandas DataFrame row to multiple other rows involves leveraging the power of numpy
. The idea is to use numpy
functions to create an array of duplicated rows and then append it to the original DataFrame.
Here’s an example:
import pandas as pd
import numpy as np
# Create a sample DataFrame
df = pd.DataFrame({'Name': ['John', 'Jane', 'Bob', 'Mary'],
'Age': [25, 30, 45, 35],
'Country': ['USA', 'Canada', 'UK', 'Australia']})
# Specify the row index to copy
row_to_copy = 1
# Number of times to replicate the row
replication_factor = 2
# Use numpy to replicate the selected row
replicated_rows = np.tile(df.loc[row_to_copy].values, (replication_factor, 1))
# Create a new DataFrame from the replicated rows
new_rows_df = pd.DataFrame(replicated_rows, columns=df.columns)
# Append the new rows to the original DataFrame
df = df._append(new_rows_df, ignore_index=True)
print(df)
Output:
Name Age Country
0 John 25 USA
1 Jane 30 Canada
2 Bob 45 UK
3 Mary 35 Australia
4 Jane 30 Canada
5 Jane 30 Canada
In this example, the numpy.tile()
function is used to replicate the values of the selected row (df.loc[row_to_copy].values
) the specified number of times (replication_factor
). The replicated values are then used to create a new DataFrame (new_rows_df
), which is appended to the original DataFrame using the append()
method.
This approach can be beneficial when dealing with larger datasets, as numpy
operations are often optimized for performance.
Conclusion
Copying a pandas DataFrame row to multiple other rows can be a useful operation when you need to replicate a certain set of values across multiple rows. This can be easily achieved using a combination of the copy()
and loc[]
methods. In this article, we explored how to copy a pandas DataFrame row to multiple other rows. We hope that this article has been helpful in your data analysis and manipulation tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.