How to Convert a Tuple of Tuples to a Pandas DataFrame in Python
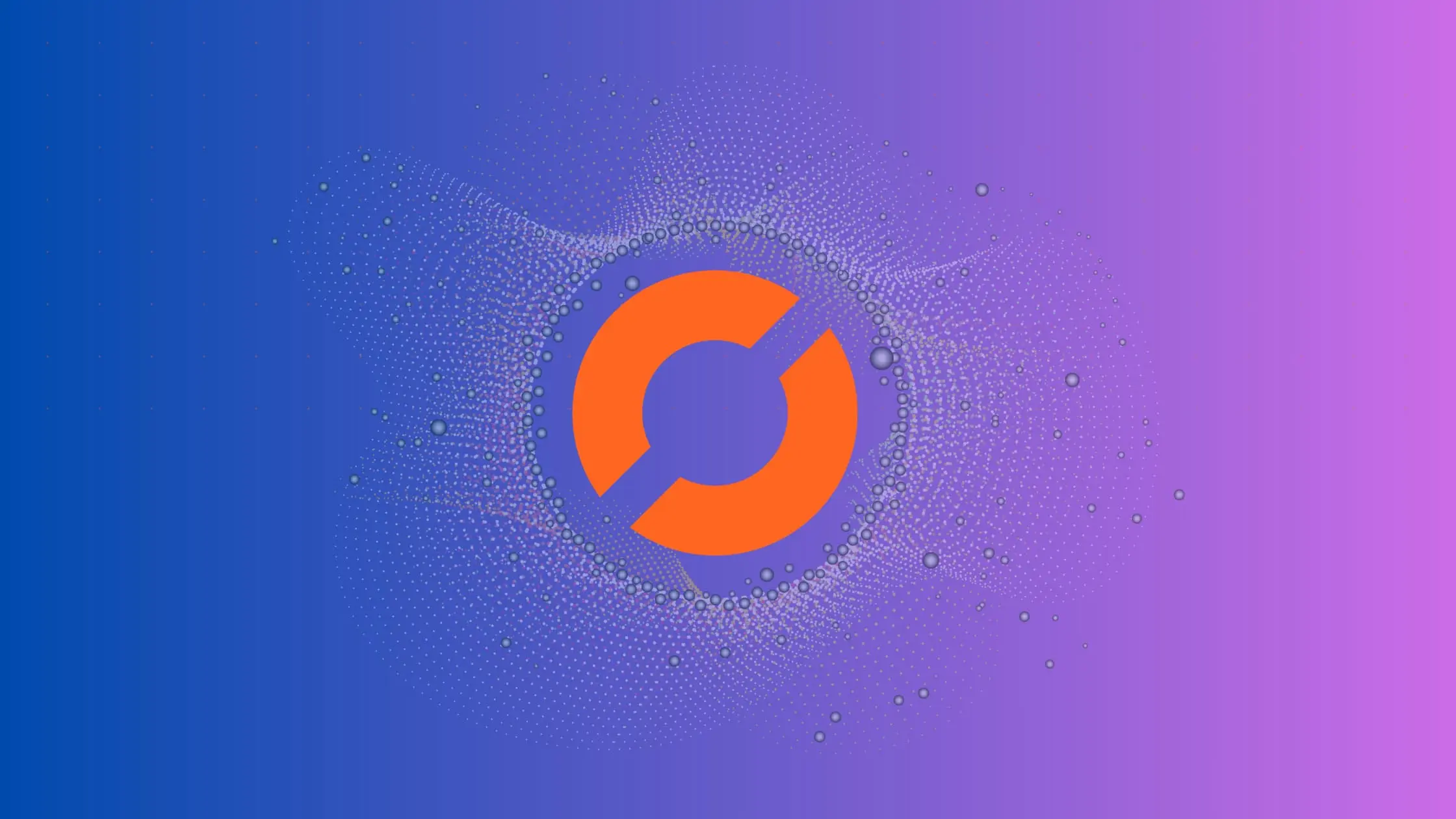
As a data scientist or software engineer, you may come across a situation where you need to convert a tuple of tuples to a Pandas DataFrame in Python. While this may seem like a daunting task at first, it is actually a straightforward process that can be accomplished using just a few lines of code.
In this article, we will walk through the steps to convert a tuple of tuples to a Pandas DataFrame in Python. We will cover the basics of Pandas, tuples, and data frames, and then dive into the process of converting a tuple of tuples to a Pandas DataFrame.
Table of Contents
- What is a Tuple in Python?
- What is Pandas in Python?
- Converting a Tuple of Tuples to a Pandas DataFrame in Python
- Common Errors and Solutions
- Conclusion
What is a Tuple in Python?
In Python, a tuple is an ordered collection of elements, which can be of any data type. Tuples are similar to lists, but they cannot be modified once they are created. Tuples are denoted by enclosing the elements within parenthesis.
Here is an example of a tuple in Python:
my_tuple = (1, 2, 3, 'four', 'five')
What is Pandas in Python?
Pandas is a popular data analysis library in Python that provides tools for data manipulation and analysis. It is built on top of the NumPy library and provides data structures for efficiently storing and manipulating large datasets.
Pandas provides two main data structures: Series and DataFrame. A Series is a one-dimensional array-like object that can store any data type. A DataFrame is a two-dimensional table-like object that can store multiple data types.
Converting a Tuple of Tuples to a Pandas DataFrame in Python
Using pandas.DataFrame()
:
To convert a tuple of tuples to a Pandas DataFrame in Python, we will use the pandas.DataFrame()
constructor. This constructor takes in several arguments, including data, columns, and index.
Here is an example of how to convert a tuple of tuples to a Pandas DataFrame:
import pandas as pd
# create a tuple of tuples
my_tuple = ((1, 'John', 25), (2, 'Sam', 30), (3, 'Sarah', 28))
# convert the tuple of tuples to a Pandas DataFrame
df = pd.DataFrame(my_tuple, columns=['ID', 'Name', 'Age'])
# print the DataFrame
print(df)
In this example, we first create a tuple of tuples containing three records. Each record contains an ID, a name, and an age.
Next, we use the pd.DataFrame()
constructor to convert the tuple of tuples to a Pandas DataFrame. We pass in the my_tuple
variable as the data argument, and we specify the column names using the columns
argument.
Finally, we print the resulting DataFrame using the print()
function.
Output:
ID Name Age
0 1 John 25
1 2 Sam 30
2 3 Sarah 28
Method 2: Using a List of Dictionaries
Another method to convert a tuple of tuples to a Pandas DataFrame is by first converting the tuple of tuples into a list of dictionaries and then using the pd.DataFrame()
constructor. Each dictionary in the list represents a row in the DataFrame, and the keys in the dictionaries correspond to the column names.
import pandas as pd
# create a tuple of tuples
my_tuple = ((1, 'John', 25), (2, 'Sam', 30), (3, 'Sarah', 28))
# convert the tuple of tuples to a list of dictionaries
list_of_dicts = [{'ID': i, 'Name': name, 'Age': age} for i, name, age in my_tuple]
# convert the list of dictionaries to a Pandas DataFrame
df = pd.DataFrame(list_of_dicts)
# print the DataFrame
print(df)
In this method, a list comprehension is used to create a list of dictionaries where each dictionary corresponds to a tuple in the original tuple of tuples. The resulting list of dictionaries is then passed to the pd.DataFrame()
constructor to create the DataFrame.
Output:
ID Name Age
0 1 John 25
1 2 Sam 30
2 3 Sarah 28
Method 3: Using the from_records
Method
The pd.DataFrame.from_records()
method provides another way to convert a tuple of tuples to a Pandas DataFrame. This method is specifically designed for creating DataFrames from a sequence of tuples.
import pandas as pd
# create a tuple of tuples
my_tuple = ((1, 'John', 25), (2, 'Sam', 30), (3, 'Sarah', 28))
# convert the tuple of tuples to a Pandas DataFrame using from_records
df = pd.DataFrame.from_records(my_tuple, columns=['ID', 'Name', 'Age'])
# print the DataFrame
print(df)
In this method, the from_records()
method is called on the pd.DataFrame
class, and the tuple of tuples is passed as the data. The columns
argument is used to specify the column names.
Output:
ID Name Age
0 1 John 25
1 2 Sam 30
2 3 Sarah 28
Common Errors and Solutions
Common Error 1: Mismatched Number of Columns
Error: If the number of columns specified in the DataFrame constructor does not match the number of elements in each tuple, a ValueError
will be raised.
Solution: Ensure that the number of columns specified in the columns
parameter matches the number of elements in each tuple.
Common Error 2: Incorrect Column Names
Error: If the column names specified in the columns
parameter do not match the number or order of elements in the tuples, a KeyError
will occur.
Solution: Double-check and verify that the column names provided in the columns
parameter accurately represent the data in the tuples.
Conclusion
Conclusion
In conclusion, transforming a tuple of tuples into a Pandas DataFrame in Python is a straightforward task that can be achieved with minimal code. By grasping the fundamentals of tuples, Pandas, and data frames, you gain the capability to efficiently manipulate and analyze extensive datasets in Python.
Moreover, we explored three methods for achieving this conversion, offering flexibility based on your preference and the nature of your data. Whether using the conventional pd.DataFrame()
constructor, employing a list of dictionaries, or utilizing the from_records
method, you have options tailored to your specific requirements.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.