Python Pandas: How to Convert a List into a string in a Pandas DataFrame
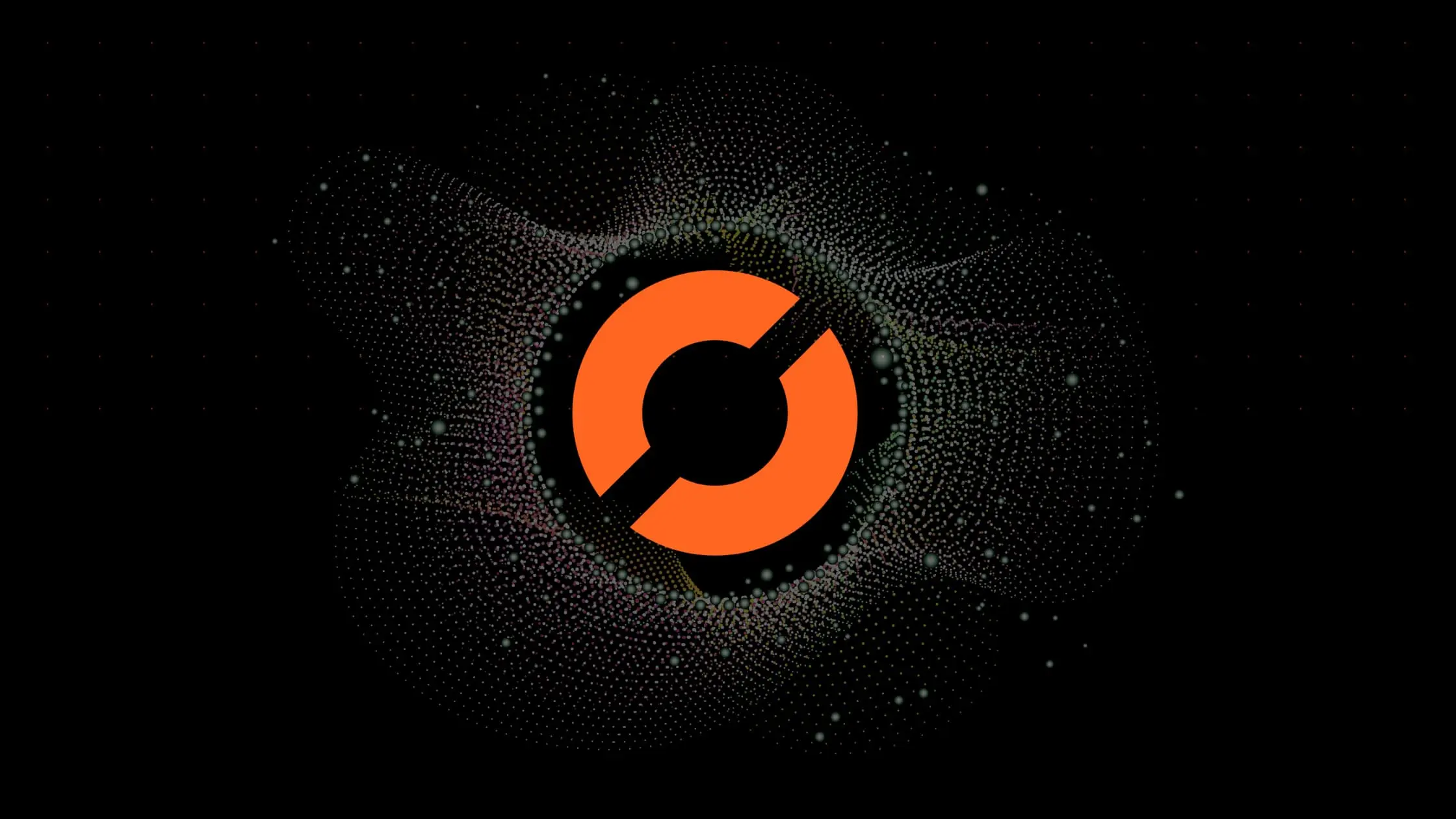
In this blog post, we will explore different methods for converting a list in a Pandas DataFrame into a string.
Method 1: Using str.join()
One of the easiest and most efficient ways to convert a list in a Pandas DataFrame into a string is by using the str.join() method. This method takes a separator string as an argument and concatenates all the elements of the list into a single string separated by the specified separator.
Here’s an example of how you can use the str.join() method to convert a list in a Pandas DataFrame into a string:
import pandas as pd
# create a sample DataFrame with a column containing lists
df = pd.DataFrame({'fruits': [['apple', 'banana'], ['orange', 'kiwi'], ['grape', 'mango']]})
# convert the lists in the 'fruits' column into strings
df['fruits'] = df['fruits'].apply(lambda x: ', '.join(x))
print(df)
Output:
fruits
0 apple, banana
1 orange, kiwi
2 grape, mango
In the code above, we first create a sample DataFrame that contains a column called ‘fruits’, which contains lists of fruits. We then use the apply() function to apply the lambda function to each element of the ‘fruits’ column. The lambda function uses the str.join() method to concatenate the elements of the list into a single string separated by a comma and a space.
Method 2: Using list comprehension and str.join()
Another way to convert a list in a Pandas DataFrame into a string is by using list comprehension and the str.join() method. This method is similar to the previous one, but it uses list comprehension to iterate over the rows of the DataFrame and apply the str.join() method to each element of the ‘fruits’ column.
Here’s an example of how you can use list comprehension and the str.join() method to convert a list in a Pandas DataFrame into a string:
import pandas as pd
# create a sample DataFrame with a column containing lists
df = pd.DataFrame({'fruits': [['apple', 'banana'], ['orange', 'kiwi'], ['grape', 'mango']]})
# convert the lists in the 'fruits' column into strings using list comprehension
df['fruits'] = [', '.join(x) for x in df['fruits']]
print(df)
Output:
fruits
0 apple, banana
1 orange, kiwi
2 grape, mango
In the code above, we first create a sample DataFrame that contains a column called ‘fruits’, which contains lists of fruits. We then use list comprehension to iterate over each element of the ‘fruits’ column and apply the str.join() method to concatenate the elements of the list into a single string separated by a comma and a space.
Method 3: Using str() and replace()
Finally, another way to convert a list in a Pandas DataFrame into a string is by using the str() function and the replace() method. This method is less efficient than the previous ones, but it can be useful in certain situations where you need to customize the separator or add other characters around the string.
Here’s an example of how you can use the str() function and the replace() method to convert a list in a Pandas DataFrame into a string:
import pandas as pd
# create a sample DataFrame with a column containing lists
df = pd.DataFrame({'fruits': [['apple', 'banana'], ['orange', 'kiwi'], ['grape', 'mango']]})
# convert the lists in the 'fruits' column into strings using str() and replace()
df['fruits'] = df['fruits'].apply(lambda x: str(x).replace('[','').replace(']','').replace('\'', '').replace(' ',''))
print(df)
Output:
fruits
0 apple,banana
1 orange,kiwi
2 grape,mango
In the code above, we first create a sample DataFrame that contains a column called ‘fruits’, which contains lists of fruits. We then use the apply() function to apply the lambda function to each element of the ‘fruits’ column. The lambda function uses the str() function to convert the list into a string and the replace() method to remove the brackets, quotes, and spaces from the string.
Conclusion
In this blog post, we have explored different methods for converting a list in a Pandas DataFrame into a string. The most efficient and recommended method is to use the str.join() method, which concatenates the elements of the list into a single string separated by a specified separator. However, depending on your specific use case, you may find the other methods using list comprehension and str() to be more suitable. Regardless of the method you choose, understanding how to convert a list in a Pandas DataFrame into a string is a valuable tool for any data scientist or software engineer working with Pandas.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.