How to Convert a Float64 Column to Int64 in Pandas
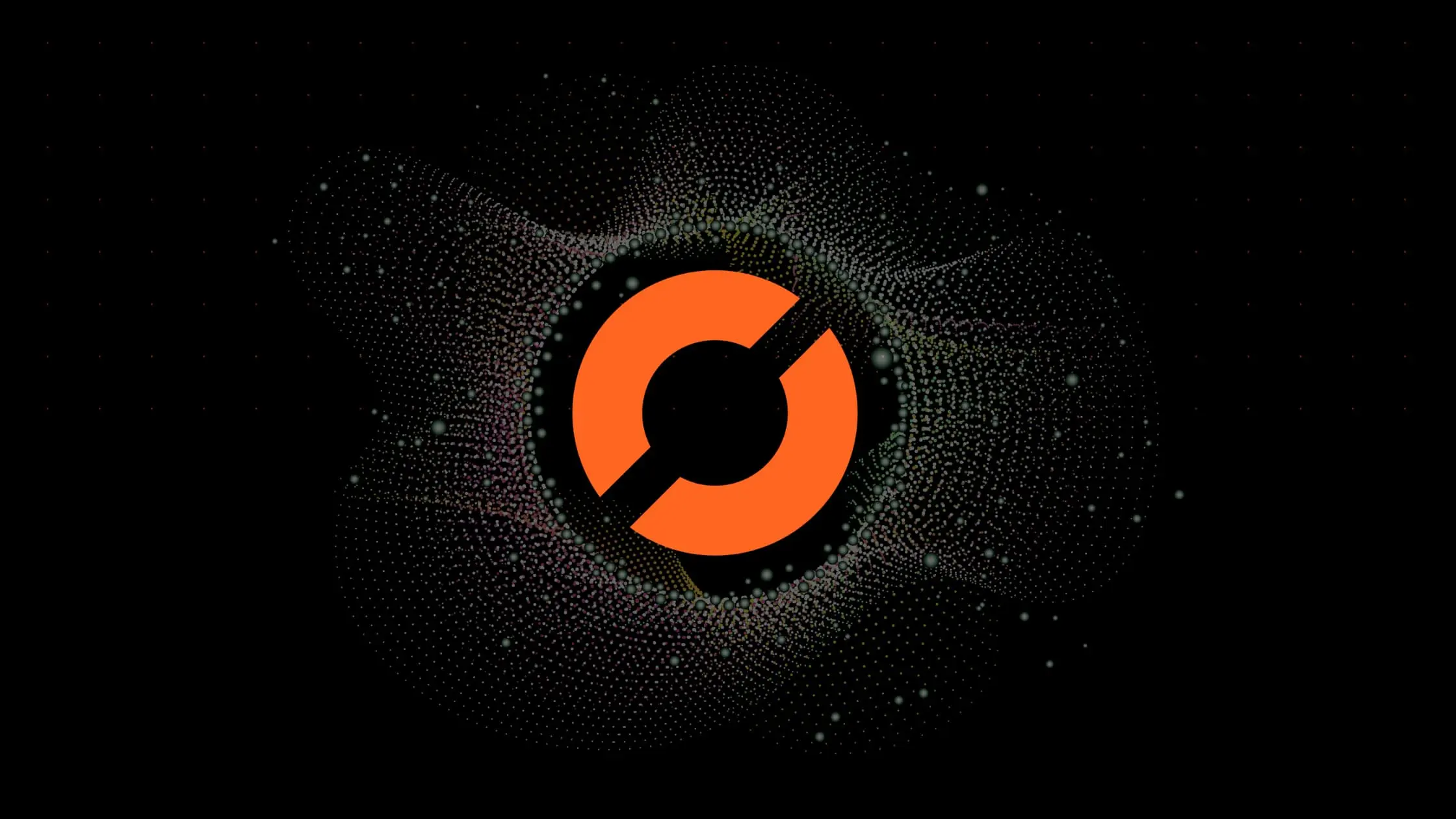
As a data scientist or software engineer, you often work with data that requires cleaning and manipulation. Pandas is a popular Python library for data manipulation and analysis, and it provides a variety of functions to help you clean and transform your data. One common task is to convert a float64 column to an int64 column. In this article, we’ll explore how to do this in Pandas.
Table of Contents
- What is a float64 column?
- Why convert a float64 column to an int64 column?
- How to convert a float64 column to an int64 column
- What if the float64 column contains missing values?
- Conclusion
What is a float64 column?
Before we dive into the conversion process, let’s briefly explain what a float64 column is. In Pandas, a float64 column is a column that contains floating-point numbers with 64-bit precision. This means that the numbers can have up to 15 decimal places. For example, the following code creates a Pandas DataFrame with a float64 column:
import pandas as pd
data = {'A': [1.23, 4.56, 7.89],
'B': [10.11, 12.13, 14.15]}
df = pd.DataFrame(data)
print(df.dtypes)
In this DataFrame, column A is a float64 column because it contains floating-point numbers.
Output:
A float64
B float64
dtype: object
Why convert a float64 column to an int64 column?
There are several reasons why you might want to convert a float64 column to an int64 column. One common reason is to save memory. Because float64 columns have 64-bit precision, they take up more memory than int64 columns, which have 64-bit integer precision. If you don’t need the decimal places in your data, converting to an int64 column can save memory without losing any information.
Another reason to convert a float64 column to an int64 column is for compatibility with other functions or libraries. Some functions or libraries may only accept integer data types, so converting your data to int64 can make it easier to work with.
How to convert a float64 column to an int64 column
Now that we’ve covered the basics, let’s dive into the conversion process. The process is actually quite simple in Pandas. You can use the astype()
method to convert a column to a different data type. In this case, we’ll convert a float64 column to an int64 column.
import pandas as pd
data = {'A': [1.23, 4.56, 7.89],
'B': [10.11, 12.13, 14.15]}
df = pd.DataFrame(data)
df['A'] = df['A'].astype('int64')
print(df.dtypes)
In this code, we first create a Pandas DataFrame with a float64 column. We then use the astype()
method to convert column A to an int64 column. Finally, we print the data types of the columns to confirm that column A is now an int64 column.
Output:
A int64
B float64
dtype: object
What if the float64 column contains missing values?
If your float64 column contains missing values, you may encounter an error when you try to convert it to an int64 column. This is because Pandas does not allow missing values in integer columns.
To handle missing values, you can use the fillna()
method to fill them with a default value before converting the column to an int64 column. For example:
import pandas as pd
import numpy as np
data = {'A': [1.23, np.nan, 7.89],
'B': [10.11, 12.13, 14.15]}
df = pd.DataFrame(data)
df['A'] = df['A'].fillna(-1).astype('int64')
print(df)
In this code, we first create a Pandas DataFrame with a float64 column that contains a missing value. We then use the fillna()
method to fill the missing value with -1. Finally, we use the astype()
method to convert column A to an int64 column.
Output:
A B
0 1 10.11
1 -1 12.13
2 7 14.15
Conclusion
Converting a float64 column to an int64 column in Pandas is a simple process that can save memory and make your data easier to work with in certain situations. By using the astype()
method, you can quickly and easily convert your data to a different data type. If your float64 column contains missing values, you can use the fillna()
method to handle them before converting to an int64 column.
I hope this article has been helpful in explaining how to convert a float64 column to an int64 column in Pandas. With this knowledge, you’ll be able to clean and manipulate your data more efficiently and effectively.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.