How to Convert a CSV File to a Dictionary in Python using the CSV and Pandas Modules
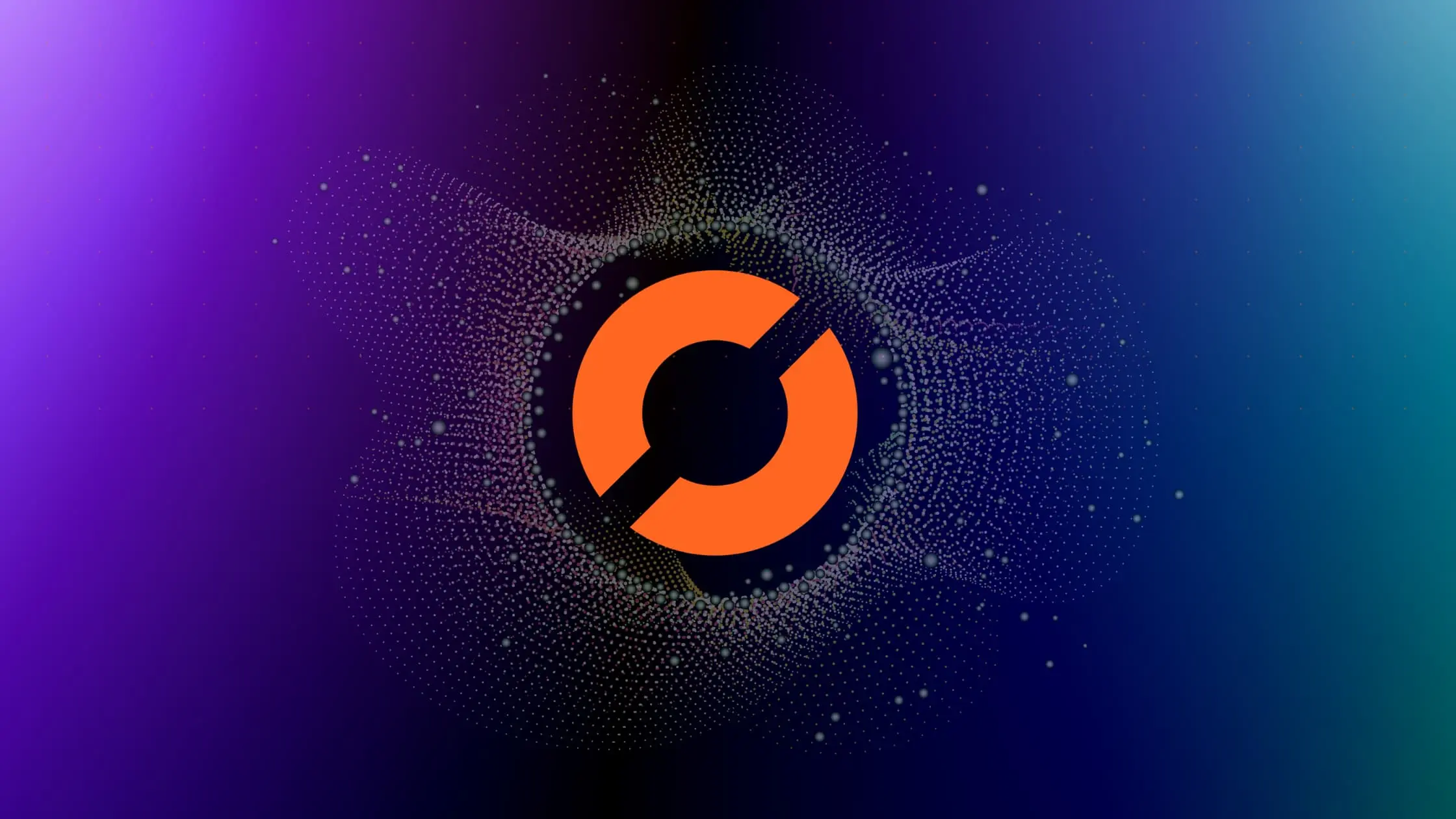
As a data scientist or software engineer, you often encounter situations where you need to work with CSV files. CSV (Comma Separated Values) files are a popular format for storing tabular data. They are used in a wide range of applications, from storing data in spreadsheets to exchanging data between systems.
In this article, we will discuss how to convert a CSV file to a dictionary in Python using the CSV and Pandas modules. We will cover the following topics:
- What is a CSV file?
- Why convert a CSV file to a dictionary?
- How to convert a CSV file to a dictionary using the CSV module.
- How to convert a CSV file to a dictionary using the Pandas module.
- Conclusion.
What is a CSV file?
A CSV file is a plain text file that stores tabular data in a structured format. Each row of the file represents a single record, and each column represents a field in the record. The fields are separated by a delimiter, which is usually a comma, but can also be a tab, semicolon, or any other character.
Why convert a CSV file to a dictionary?
In some cases, it may be more convenient to work with a CSV file as a dictionary. A dictionary is a data structure that stores key-value pairs. In the context of a CSV file, the keys could be the column headers, and the values could be the values in each row.
Converting a CSV file to a dictionary can make it easier to manipulate the data and perform calculations or analysis. For example, you may want to group the data by a particular field, or calculate summary statistics for each group. Let’s say we have a csv file like this:
Name,Age,City
Alice,28,New York
Bob,32,Los Angeles
Charlie,24,Chicago
How to convert a CSV file to a dictionary using the CSV module.
The CSV module is a built-in module in Python that provides functionality for working with CSV files. The module provides a reader object that can be used to read a CSV file row by row.
To convert a CSV file to a dictionary using the CSV module, we need to do the following steps:
- Open the CSV file using the
open()
function. - Create a
csv.reader
object using thecsv.reader()
function. - Read the header row to get the column names.
- Loop through the remaining rows and create a dictionary for each row.
- Append each dictionary to a list.
Here is an example code snippet:
# import csv
import csv
# read csv file to a list of dictionaries
with open('data.csv', 'r') as file:
csv_reader = csv.DictReader(file)
data = [row for row in csv_reader]
print(data)
Output:
[
{'Name': 'Alice', 'Age': '28', 'City': 'New York'},
{'Name': 'Bob', 'Age': '32', 'City': 'Los Angeles'},
{'Name': 'Charlie', 'Age': '24', 'City': 'Chicago'}
]
In this example, we’re using a CSV file named data.csv
in read (r
) mode. We create a csv.DictReader
object to read the file and automatically convert each row into a dictionary.
The data
variable then holds a list of dictionaries, where each dictionary represents a row from the CSV file. The keys of the dictionaries are the column headers, and the values are the corresponding data.
How to convert a CSV file to a dictionary using the Pandas module.
The Pandas module is a popular third-party library for data analysis in Python. It provides a high-level interface for working with tabular data, including CSV files.
To convert a CSV file to a dictionary using the Pandas module, we need to do the following steps:
- Import the Pandas module.
- Use the
read_csv()
function to read the CSV file into a Pandas DataFrame. - Use the
to_dict()
method to convert the DataFrame to a dictionary.
Here is an example code snippet:
# import pandas
import pandas as pd
# read csv
data = pd.read_csv('data.csv')
# Convert the DataFrame to a Dictionary
data_dict = data.to_dict(orient='records')
print(data_dict)
Output:
[
{'Name': 'Alice', 'Age': '28', 'City': 'New York'},
{'Name': 'Bob', 'Age': '32', 'City': 'Los Angeles'},
{'Name': 'Charlie', 'Age': '24', 'City': 'Chicago'}
]
In this example, we import the Pandas module using the import
statement. We then use the read_csv()
function to read the CSV file data.csv
into a Pandas DataFrame data
.
Finally, we use the to_dict()
method of the DataFrame to convert it to a dictionary dict_data
. The to_dict()
method takes an argument orient
that specifies the orientation of the output dictionary. In this case, we set orient='records'
to get a list of dictionaries, where each dictionary represents a row in the DataFrame.
Conclusion.
In this article, we have discussed how to convert a CSV file to a dictionary in Python using the CSV and Pandas modules. We have covered the basic concepts of CSV files and dictionaries, and provided code examples for both the CSV and Pandas approaches.
Converting a CSV file to a dictionary can be a useful technique in data analysis and manipulation. By using the techniques discussed in this article, you can easily read and manipulate CSV data in Python.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.