How to Convert a Column in Pandas DataFrame from String to Float
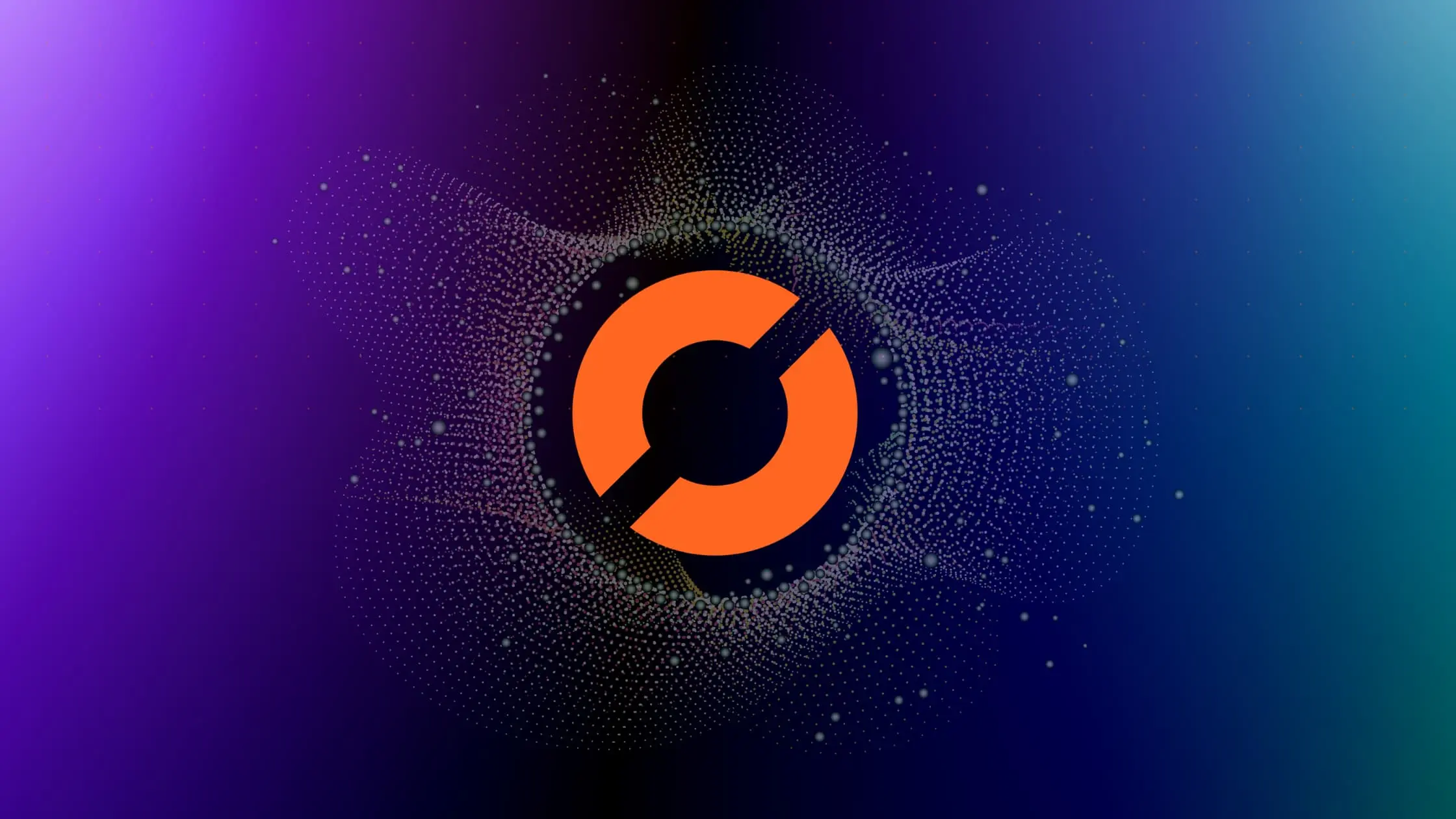
As a data scientist or software engineer, you may encounter situations where you need to convert a column in a pandas DataFrame from a string to a float. This can be a common task, especially when dealing with numeric data that has been read in as strings. In this blog post, I will show you how to convert a column in a pandas DataFrame from a string to a float.
What is a Pandas DataFrame?
Before we dive into the details of converting a column in a pandas DataFrame from a string to a float, let’s first define what a pandas DataFrame is. A pandas DataFrame is a two-dimensional data structure that is used for storing tabular data. It is similar to a spreadsheet or a SQL table, where each column represents a variable and each row represents an observation.
How to Convert a Column in Pandas DataFrame from String to Float
Let’s say we have this csv file that has a column of string as follow:
NumericData
0 1.23
1 4.56
2 7.89
3 10.11
4 12.34
To convert a column in a pandas DataFrame from a string to a float, we need to use the astype()
method. The astype()
method is used to cast a pandas object to a specified dtype.
Step 1: Load the Data into a Pandas DataFrame
First, let’s load some sample data into a pandas DataFrame. For this example, we will be using the read_csv()
method to read in a CSV file that contains some sample data.
import pandas as pd
# Load the data into a pandas DataFrame
df = pd.read_csv('data.csv')
Step 2: Check the Data Types of the Columns
Next, let’s check the data types of the columns in the DataFrame using the dtypes
attribute.
# Check the data types of the columns
print(df.dtypes)
This will output the data types of each column in the DataFrame. If we have a column that is stored as a string, it will be displayed as object
.
Output:
NumericData object
dtype: object
Step 3: Convert the Column from String to Float
To convert a column from a string to a float, we can use the astype()
method. We will pass the float
data type as an argument to the astype()
method.
# Convert the 'column_name' column from string to float
df['NumericData_Float'] = df['NumericData'].astype(float)
print(df)
Output:
NumericData NumericData_Float
0 1.23 1.23
1 4.56 4.56
2 7.89 7.89
3 10.11 10.11
4 12.34 12.34
Step 4: Check the Data Types of the Columns Again
Finally, let’s check the data types of the columns in the DataFrame again using the dtypes
attribute.
# Check the data types of the columns again
print(df.dtypes)
Output:
NumericData object
NumericData_Float float64
dtype: object
If we have done everything correctly, the column we converted from a string to a float should now be displayed as float64
.
Conclusion
Converting a column in a pandas DataFrame from a string to a float is a simple task that can be accomplished using the astype()
method. In this blog post, we covered the steps required to convert a column from a string to a float. By following these steps, you can ensure that your data is stored in the correct data type, which is important for performing mathematical operations and generating accurate results.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.