How to Compute Row Average in Pandas
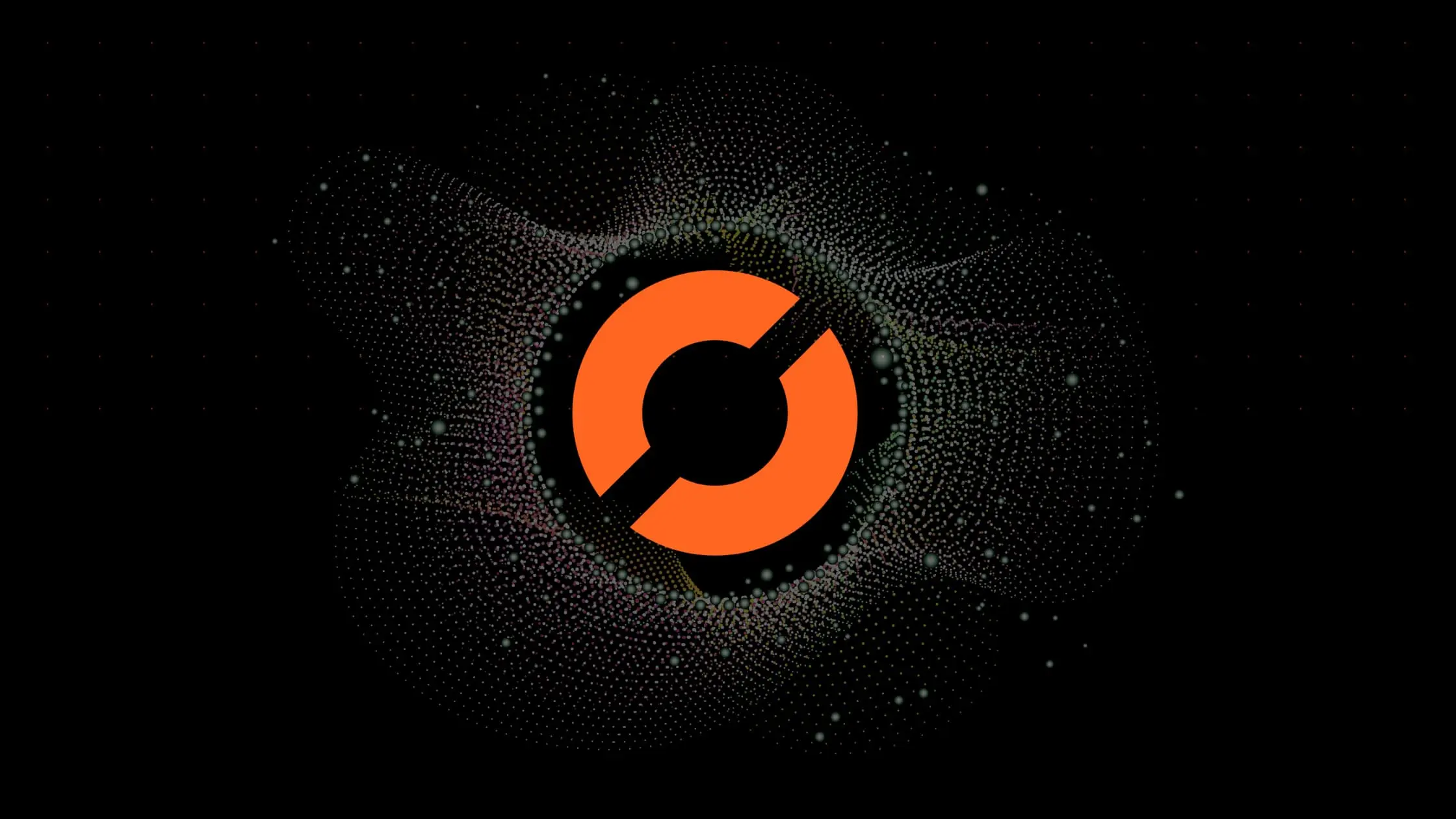
As a data scientist or software engineer, you may often need to compute row averages when working with data in pandas. Pandas is a powerful and popular Python library for data manipulation and analysis, and it provides several ways to compute row averages.
In this article, we will explore different methods to compute row averages in pandas and provide examples for each method. We will also discuss the advantages and disadvantages of each method to help you choose the best approach for your use case.
What Is Pandas?
Before we dive into computing row averages in pandas, let’s briefly review what pandas is and why it is so popular among data scientists and software engineers.
Pandas is a Python library designed for data manipulation and analysis. It provides data structures for efficiently storing and manipulating large datasets, as well as tools for cleaning, merging, filtering, and transforming data. With pandas, you can easily load data from various sources, such as CSV files, Excel spreadsheets, SQL databases, and web APIs, and perform complex calculations and visualizations on the data.
Pandas is widely used in data science, machine learning, finance, and other fields where data analysis is critical. Its popularity is due to its ease of use, flexibility, and performance. Pandas is built on top of NumPy, another popular Python library for scientific computing, and it takes advantage of the fast and efficient array operations provided by NumPy.
How to Compute Row Average in Pandas
Now that we have reviewed what pandas is, let’s focus on how to compute row averages in pandas. There are several ways to do this, and we will cover three common methods: using the mean()
method, using the apply()
method, and using the aggregate()
method.
Method 1: Using the mean() Method
The simplest way to compute row averages in pandas is to use the mean()
method. This method calculates the mean (i.e., average) of a DataFrame or Series along a specified axis, which can be either rows or columns. To compute row averages, we need to set the axis
parameter to 1, which indicates that we want to compute the mean across rows.
Here is an example of how to use the mean()
method to compute row averages in pandas:
import pandas as pd
# create a sample DataFrame
data = {'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]}
df = pd.DataFrame(data)
# compute row averages using the mean() method
row_avg = df.mean(axis=1)
# print the row averages
print(row_avg)
Output:
0 4.0
1 5.0
2 6.0
dtype: float64
In this example, we first create a sample DataFrame with three columns and three rows. We then use the mean()
method to compute the row averages, and store the result in the row_avg
variable. Finally, we print the row averages to the console.
Note that the mean()
method returns a Series object, where each element corresponds to the row average of the corresponding row in the original DataFrame.
Method 2: Using the apply() Method
Another way to compute row averages in pandas is to use the apply()
method. This method applies a function to each row or column of a DataFrame or Series, and returns the result as a new DataFrame or Series.
To compute row averages using the apply()
method, we need to define a function that calculates the row average, and then apply this function to each row of the DataFrame using the apply()
method.
Here is an example of how to use the apply()
method to compute row averages in pandas:
import pandas as pd
# create a sample DataFrame
data = {'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]}
df = pd.DataFrame(data)
# define a function to compute row average
def row_average(row):
return row.mean()
# apply the row_average function to each row using the apply() method
row_avg = df.apply(row_average, axis=1)
# print the row averages
print(row_avg)
Output:
0 4.0
1 5.0
2 6.0
dtype: float64
In this example, we first create a sample DataFrame with three columns and three rows. We then define a function row_average()
that calculates the row average of a given row. We apply this function to each row of the DataFrame using the apply()
method with axis=1
. Finally, we store the row averages in the row_avg
variable and print them to the console.
Note that the apply()
method returns a Series object, where each element corresponds to the result of applying the function to the corresponding row in the original DataFrame.
Method 3: Using the aggregate() Method
The third method to compute row averages in pandas is to use the aggregate()
method. This method allows us to apply multiple aggregation functions to a DataFrame or Series at once, and returns the result as a new DataFrame or Series.
To compute row averages using the aggregate()
method, we need to define a dictionary that maps the column names to the aggregation functions we want to apply. In this case, we only need to apply the mean()
function to each row, so we can use the shorthand notation {'column_name': 'mean'}
.
Here is an example of how to use the aggregate()
method to compute row averages in pandas:
import pandas as pd
# create a sample DataFrame
data = {'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]}
df = pd.DataFrame(data)
# compute row averages using the aggregate() method
row_avg = df.aggregate('mean', axis=1)
# print the row averages
print(row_avg)
Output:
0 4.0
1 5.0
2 6.0
dtype: float64
In this example, we first create a sample DataFrame with three columns and three rows. We then use the aggregate()
method to compute the row averages, and store the result in the row_avg
variable. Finally, we print the row averages to the console.
Note that the aggregate()
method returns a Series object, where each element corresponds to the result of applying the aggregation functions to the corresponding row in the original DataFrame.
Conclusion
In this article, we have explored different methods to compute row averages in pandas. We have covered three common methods: using the mean()
method, using the apply()
method, and using the aggregate()
method. Each method has its own advantages and disadvantages, and the best approach depends on your specific use case.
The mean()
method is the simplest and fastest method to compute row averages, but it may not be flexible enough for more complex calculations. The apply()
method allows you to define custom functions and apply them to each row, but it may be slower than the other methods for large datasets. The aggregate()
method allows you to apply multiple aggregation functions at once, but it may be less intuitive than the other methods for simple calculations.
Regardless of the method you choose, pandas provides a powerful and flexible toolkit for data manipulation and analysis, and computing row averages is just one of the many tasks you can accomplish with pandas.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.