How to Combine Two Pandas Dataframes with the Same Index
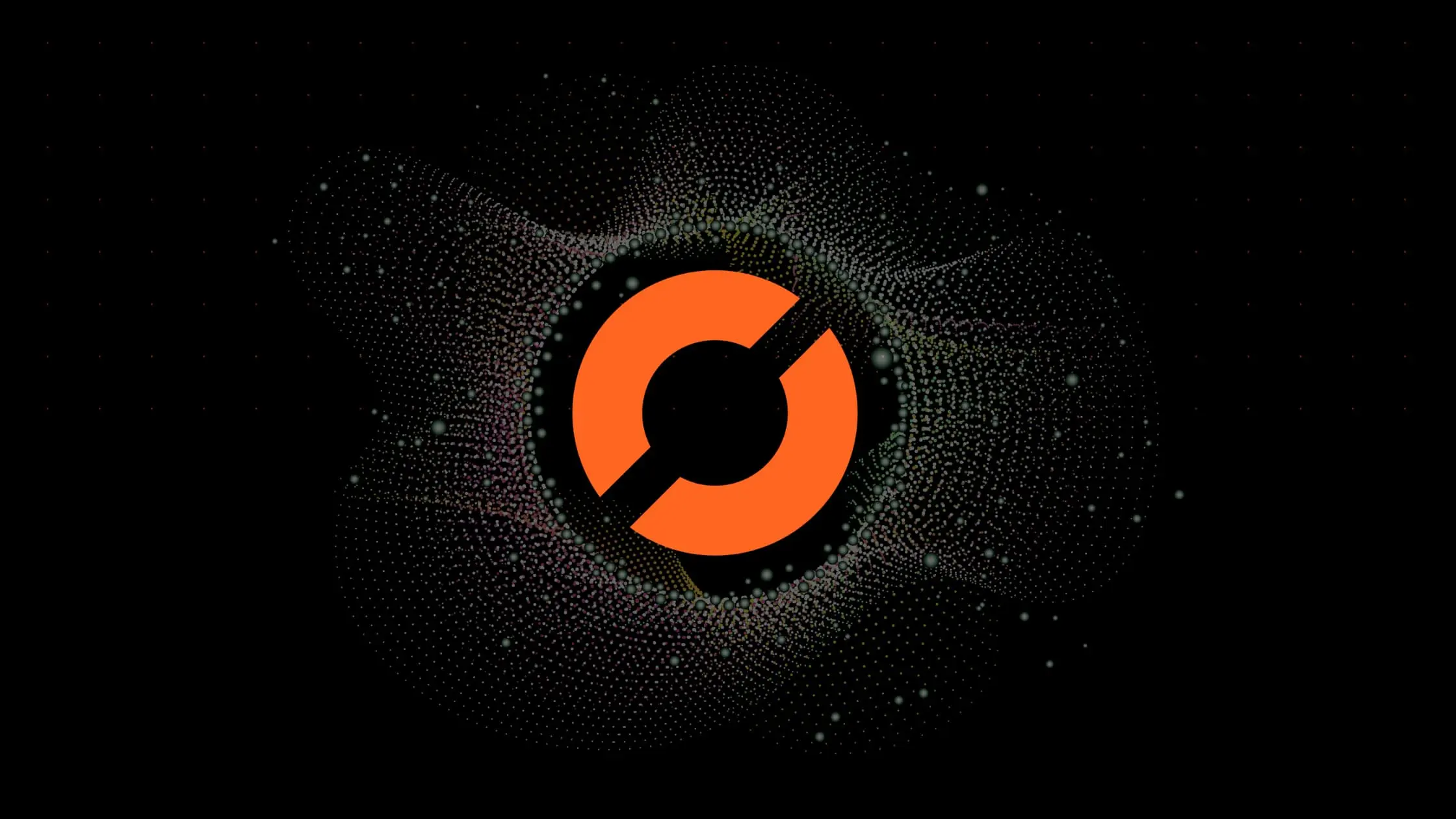
As a data scientist or software engineer, working with large datasets is a common occurrence. One of the most common tasks when working with datasets is combining multiple dataframes. In this article, we will discuss how to combine two Pandas dataframes with the same index.
Table of Contents
- Introduction
- What are Pandas Dataframes?
- Why Combine Two Pandas Dataframes with the Same Index?
- How to Combine Two Pandas Dataframes with the Same Index?
- Conclusion
What are Pandas Dataframes?
Pandas is an open-source data manipulation library for Python. It is used for data analysis and data manipulation. A dataframe is a two-dimensional size-mutable, tabular data structure. It is like a spreadsheet with rows and columns. Each column can have a different data type.
Why Combine Two Pandas Dataframes with the Same Index?
There are many reasons why you might want to combine two dataframes with the same index. For example, you might have two datasets with different columns, and you want to combine them into one dataset. Or you might have two datasets with the same columns, but different values, and you want to merge them into one dataset.
How to Combine Two Pandas Dataframes with the Same Index?
There are several ways to combine two dataframes with the same index. In this article, we will discuss two methods: concat()
and merge()
.
Method 1: Using concat()
The concat()
function is used to concatenate two or more dataframes along a particular axis. It can be used to concatenate dataframes horizontally (along columns) or vertically (along rows).
Here is the syntax for using concat()
:
result = pd.concat([df1, df2], axis=1)
Where df1
and df2
are the dataframes you want to concatenate, and axis=1
specifies that you want to concatenate the dataframes horizontally.
Here is an example:
import pandas as pd
# create two dataframes with the same index
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]}, index=[0, 1, 2])
df2 = pd.DataFrame({'C': [7, 8, 9], 'D': [10, 11, 12]}, index=[0, 1, 2])
# concatenate the dataframes horizontally
result = pd.concat([df1, df2], axis=1)
print(result)
Output:
A B C D
0 1 4 7 10
1 2 5 8 11
2 3 6 9 12
Pros
- Flexibility:
pd.concat()
is very flexible and can concatenate along either axis (rows or columns). It can handle various concatenation scenarios. - Fine-grained control: You have control over the concatenation axis (
axis
parameter), handling duplicate indices, and more.
Cons
- Potential for duplicate indices: If the dataframes being concatenated have overlapping indices,
pd.concat()
might retain duplicate indices, which could be an issue in certain scenarios. - Complexity: The flexibility can lead to more complex syntax and potential confusion, especially for beginners.
Method 2: Using merge()
The merge()
function is used to merge two dataframes based on a common column or index. It can be used to merge dataframes horizontally (along columns) or vertically (along rows).
Here is the syntax for using merge()
:
result = pd.merge(df1, df2, on='index')
Where df1
and df2
are the dataframes you want to merge, and on='index'
specifies that you want to merge the dataframes based on the index.
Here is an example:
import pandas as pd
# create two dataframes with the same index
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]}, index=[0, 1, 2])
df2 = pd.DataFrame({'C': [7, 8, 9], 'D': [10, 11, 12]}, index=[0, 1, 2])
# merge the dataframes based on the index
result = pd.merge(df1, df2, on='index')
print(result)
Output:
A B C D
0 1 4 7 10
1 2 5 8 11
2 3 6 9 12
Pros
- Merging capabilities:
merge()
is powerful for combining dataframes based on common columns or indices. It allows for different types of joins, such as inner, outer, left, and right. - Handling non-matching indices: If the dataframes being merged have non-matching indices,
merge()
provides a way to handle such situations using theleft_index
andright_index
parameters.
Cons
Specific use case: It’s primarily designed for horizontal merging based on columns or indices. Using it for vertical concatenation might lead to confusion.
Complexity: Similar to
pd.concat()
,merge()
can be complex, especially for users who are not familiar with database-style merging.
Method 3: Using append()
The append()
method in Pandas is used to concatenate two dataframes vertically. It is a convenient way to add rows from one dataframe to another.
Here is the syntax for using append():
result = df1.append(df2, ignore_index=True)
df1
anddf2
are the dataframes you want to concatenate.ignore_index=True
ensures that the resulting dataframe has a new, continuous index. Here is an example:
import pandas as pd
# create two dataframes with the same index
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]}, index=[0, 1, 2])
df2 = pd.DataFrame({'A': [4, 5, 6], 'B': [7, 8, 9]}, index=[3, 4, 5])
# concatenate the dataframes vertically
result = df1.append(df2, ignore_index=True)
print(result)
Output:
A B
0 1 4
1 2 5
2 3 6
3 4 7
4 5 8
5 6 9
Pros
- Simplicity:
append()
is a straightforward method for concatenating dataframes vertically. It’s easy to understand and use. - Automatic index handling: The i
gnore_index
parameter helps automatically reindex the resulting dataframe, avoiding potential issues with duplicate indices.
Cons
- Limited flexibility: While suitable for simple vertical concatenation, append() might not offer the same level of flexibility as
pd.concat()
for more complex concatenation scenarios. - Limited control: If you need fine-grained control over the concatenation process,
append()
might not be the best choice.
Conclusion
In this article, we have discussed two methods for combining two Pandas dataframes with the same index: concat()
and merge()
. Both methods are useful, depending on the specific use case. By knowing how to combine dataframes, you can manipulate and analyze large datasets more efficiently.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.