How to Color Cells in Excel with Pandas
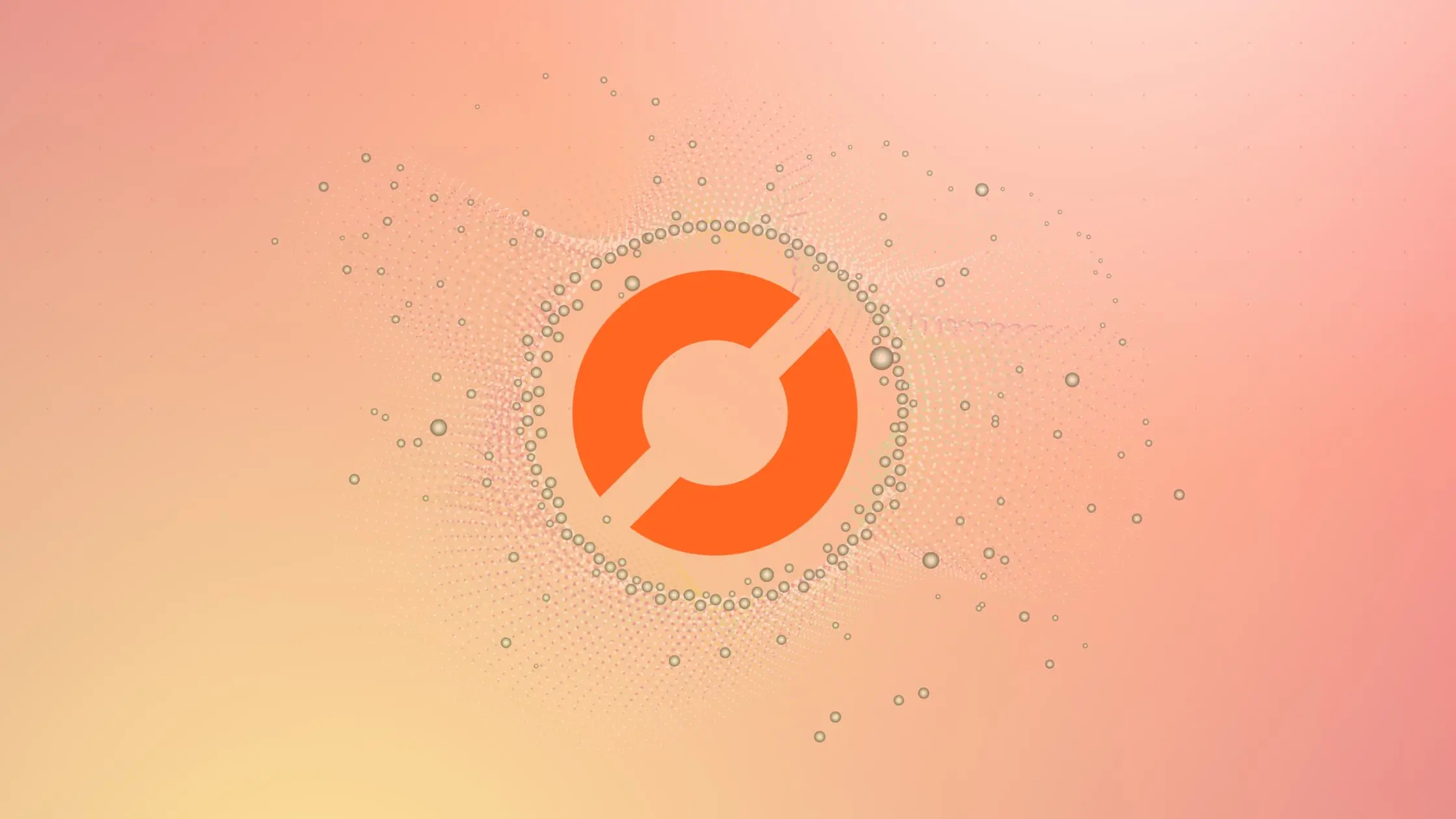
As a data scientist or software engineer, you understand the importance of data visualization. One of the most popular tools for data visualization is Microsoft Excel. However, manually coloring cells in Excel can be a tedious and time-consuming process. Fortunately, pandas, a popular data analysis library in Python, provides an easy way to color cells in Excel.
In this article, we will explain how to color cells in Excel with pandas. We will provide step-by-step instructions for coloring cells in Excel based on specific conditions.
Table of Contents
What is Pandas?
Before we dive into how to color cells in Excel with pandas, let’s briefly discuss what pandas is.
Pandas is an open-source data analysis and manipulation library for Python. It provides data structures for efficiently storing and manipulating large data sets. Pandas is widely used in the data science and machine learning communities for data exploration, cleaning, and preparation.
How to Color Cells in Excel with Pandas
When working with data, it is often useful to highlight specific cells based on certain conditions. For example, you may want to highlight cells with values above a certain threshold or cells with negative values.
Pandas provides a simple and efficient way to color cells in Excel based on specific conditions using the style
attribute of a pandas DataFrame.
Step 1: Import Pandas
The first step is to import pandas into your Python environment. You can do this by running the following command:
import pandas as pd
Step 2: Create a DataFrame
Next, you need to create a pandas DataFrame that contains the data you want to color in Excel. For example, let’s create a DataFrame that contains the following data:
import numpy as np
data = {'Name': ['John', 'Jane', 'Bob', 'Mary'],
'Age': [25, 30, 20, 35],
'Salary': [50000, 60000, 45000, 70000]}
df = pd.DataFrame(data)
This will create a DataFrame that looks like this:
Name Age Salary
0 John 25 50000
1 Jane 30 60000
2 Bob 20 45000
3 Mary 35 70000
Step 3: Color Cells Based on Specific Conditions
Now that you have a DataFrame, you can use the style
attribute to color cells based on specific conditions. For example, let’s highlight cells with salaries above 50000:
def highlight_salary(s):
'''
Highlight cells in Salary column with values above 50000
'''
is_above_threshold = s > 50000
return ['background-color: yellow' if v else '' for v in is_above_threshold]
df.style.apply(highlight_salary, subset=['Salary'])
This will highlight cells in the Salary
column with values above 50000 with a yellow background.
You can also color cells based on other conditions, such as negative values:
def highlight_negative(s):
'''
Highlight cells with negative values
'''
is_negative = s < 0
return ['background-color: red' if v else '' for v in is_negative]
df.style.apply(highlight_negative)
This will highlight cells with negative values with a red background.
Step 4: Export DataFrame to Excel
Finally, you can export the DataFrame to an Excel file using the to_excel
method:
df.style.apply(highlight_salary, subset=['Salary']).to_excel('output.xlsx', index=False)
This will create an Excel file named output.xlsx
with the highlighted cells.
Conclusion
In this article, we have explained how to color cells in Excel with pandas. We have provided step-by-step instructions for coloring cells in Excel based on specific conditions. By using pandas, you can save time and automate the process of coloring cells in Excel.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.