How to Check Pandas Dataframe Column for String Type
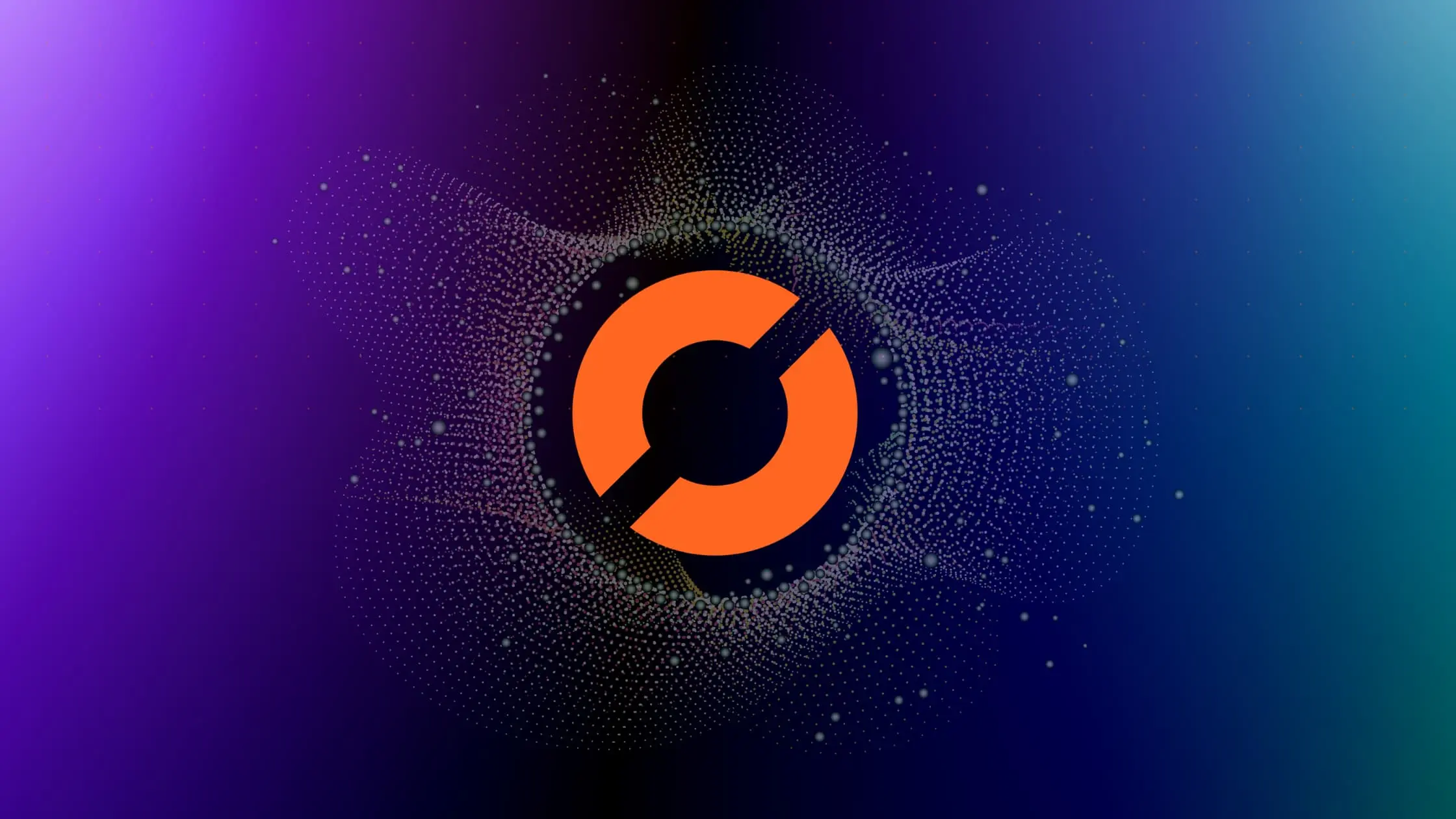
Table of Contents
- 2.1 Using the dtype Attribute
- 2.2 Using the select_dtypes() Method
- 2.3 Using pd.api.types.is_string_dtype
- 2.4 Using the apply() Method
In this post, we will explore various methods to check if a column in a pandas dataframe is of string type.
Introduction to Pandas Dataframe
Before we dive into the details of checking the data types of columns in a pandas dataframe, let’s first define what a pandas dataframe is. A pandas dataframe is a two-dimensional table-like data structure that consists of rows and columns. Each column can have a different data type, such as integers, floats, strings, and so on. Pandas is a popular Python library used for data manipulation and analysis, and it provides a powerful set of tools for working with dataframes.
Checking if a Column Contains String Data
Let’s assume that we have a pandas dataframe df
that contains several columns, and we want to check if a column named column_name
contains string data. There are several ways to achieve this goal, and we will discuss some of them below. Lets define a dataframe to use with our examples below:
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'London', 'Paris'],
'Occupation': ['Data Scientist', 'Software Engineer', 'Data Analyst']
}
df = pd.DataFrame(data)
print(df)
Output:
Name Age City Occupation
0 Alice 25 New York Data Scientist
1 Bob 30 London Software Engineer
2 Charlie 35 Paris Data Analyst
Using the dtype Attribute
One simple way to check the data type of a column in a pandas dataframe is to use the dtype
attribute. This attribute returns the data type of the column as a string. Here’s how you can use it to check for the Name
column in our dataframe:
column_type = df['column_name'].dtype
if column_type == 'object':
print('The column contains string data')
else:
print('The column does not contain string data')
Output:
The column contains string data
In this code snippet, we use the dtype
attribute to get the data type of the Name
column. Since the data type is 'object'
, then the column contains string data. Otherwise, it does not.
Using the select_dtypes() Method
Another way to check if a column contains string data is to use the select_dtypes()
method. This method returns a subset of the dataframe that contains columns of a certain data type. Here’s how you can use it:
string_columns = df.select_dtypes(include=['object']).columns
if 'Age' in string_columns:
print('The column contains string data')
else:
print('The column does not contain string data')
Output:
The column does not contain string data
In this code snippet, we use the select_dtypes()
method to get a subset of the dataframe that contains columns of the 'object'
data type, which is the data type of strings in pandas. We then check if the Age
column is in this subset of columns. Since it doesn’t contain any string data, our output shows that.
Using pd.api.types.is_string_dtype
pd.api.types.is_string_dtype
function checks if the provided dtype is a string type. Both approaches can be used based on your preference.
if pd.api.types.is_string_dtype(df['City']):
print("The column contains strings.")
else:
print("The column does not contain strings.")
Output:
The column contains strings.
Using the apply() Method
A Fourth way to check if a column contains string data is to use the apply()
method. This method applies a function to each element of the column and returns a new series with the results. Here’s how you can use it:
def is_string(x):
return isinstance(x, str)
is_string_series = df['Occupation'].apply(is_string)
if is_string_series.any():
print('The column contains string data')
else:
print('The column does not contain string data')
Output:
The column contains string data
In this code snippet, we define a function is_string()
that returns True
if its argument is a string. We then use the apply()
method to apply this function to each element of the Occupation
column, which returns a new series with boolean values indicating whether each element is a string or not. We then check if any of these values are True
, which indicates that the column contains string data.
Pros and Cons
| --------------------| ----------------------|----------------------|
| Method | Pros | Cons |
| --------------------| ----------------------|----------------------|
| dtype attribute | Simple, direct, | Doesn't identify |
| | efficient for single- | mixed-type columns, |
| | column checks | might not distinguish|
| | | string subtypes |
| --------------------| ----------------------|----------------------|
| select_dtypes() | Handles multiple | Less efficient for |
| | columns, identifies | single-column checks |
| | mixed-type columns | might not distinguish|
| | | string subtypes |
| --------------------| ----------------------|----------------------|
| pd.api.types. | Clear intent, handles | Less commonly |
| is_string_dtype() | mixed-type columns, | used, require |
| | distinguishes string | additional import |
| | subtypes | |
| --------------------| ----------------------|----------------------|
| apply() method | Customizable checks, | Potential perform- |
| | handles complex logic | ance overhead for |
| | | large datasets |
| --------------------| ----------------------|----------------------|
Choosing the Best Method:
- For quick single-column checks: Use
dtype
orpd.api.types.is_string_dtype()
. - For multiple columns or mixed-type checks: Use
select_dtypes()
orpd.api.types.is_string_dtype()
. - For complex logic or customized checks: Use
apply()
. - For clarity and type differentiation: Prefer
pd.api.types.is_string_dtype()
.
Conclusion
In this post, we discussed various methods to check if a column in a pandas dataframe contains string data. These methods include using the dtype
attribute, the select_dtypes()
method, the pd.api.types.is_string_dtype
attribute, and the apply()
method. By using these methods, you can quickly and easily identify columns that contain string data in your data analysis projects.
Remember, having a good understanding of the data you’re working with is crucial in data analysis. By identifying the data types of columns in your dataframes, you can make informed decisions about how to manipulate and analyze your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.