How to Check if Pandas Column Has Value from List of Strings
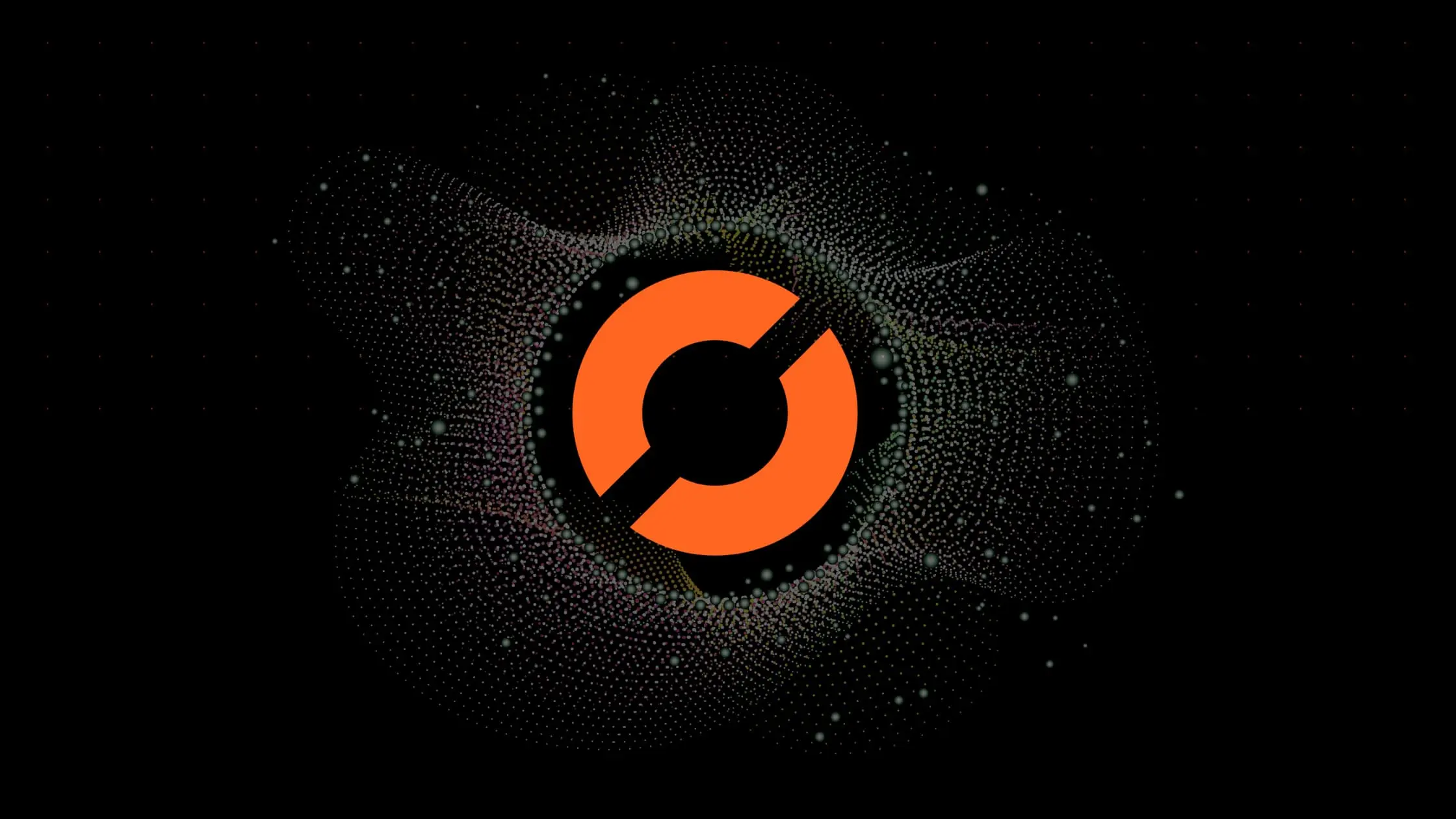
How to Check if Pandas Column Has Value from List of Strings
As a data scientist or software engineer working with Pandas, it’s important to know how to efficiently check whether a column contains any value from a given list of strings. In this article, we’ll go through a few methods to accomplish this task and discuss their pros and cons.
The Problem
Suppose we have a Pandas DataFrame with a column called fruit
that contains various types of fruits. We also have a list of fruits we are interested in, say ['apple', 'banana', 'orange']
. Our goal is to check whether the fruit
column contains any of these fruits.
Method 1: Using .isin()
One simple and efficient way to check if a Pandas column has a value from a list of strings is to use the .isin()
method. This method returns a boolean Series indicating whether each element in the column is contained in the given list.
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'fruit': ['apple', 'banana', 'pear', 'kiwi', 'orange']})
# create a list of fruits we are interested in
fruits_to_check = ['apple', 'banana', 'orange']
# check if the 'fruit' column contains any of the fruits we are interested in
mask = df['fruit'].isin(fruits_to_check)
# print the resulting DataFrame, containing only the rows that match the mask
print(df[mask])
Output:
fruit
0 apple
1 banana
4 orange
As you can see, the resulting DataFrame only contains the rows where the fruit
column matches one of the fruits in the fruits_to_check
list.
The .isin()
method is very fast and efficient, especially for large DataFrames. However, it has a few limitations. One limitation is that it only checks for exact matches, so it won’t work if we want to check for substrings or case-insensitive matches.
Method 2: Using a List Comprehension
Another way to check if a Pandas column has a value from a list of strings is to use a list comprehension. This method involves iterating over each element in the column and checking if it is contained in the given list.
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'fruit': ['apple', 'banana', 'pear', 'kiwi', 'orange']})
# create a list of fruits we are interested in
fruits_to_check = ['apple', 'banana', 'orange']
# check if the 'fruit' column contains any of the fruits we are interested in
mask = [fruit in fruits_to_check for fruit in df['fruit']]
# print the resulting DataFrame, containing only the rows that match the mask
print(df[mask])
Output:
fruit
0 apple
1 banana
4 orange
The list comprehension method works similarly to the .isin()
method, but it gives us more flexibility in terms of matching criteria. For example, we can easily check for substrings or case-insensitive matches by modifying the list comprehension.
However, the list comprehension method can be slower and less efficient than the .isin()
method, especially for large DataFrames. It also requires more code and is less readable.
Conclusion
In this article, we’ve learned two ways to check if a Pandas column has a value from a list of strings: using the .isin()
method and using a list comprehension. Both methods have their pros and cons, and the choice depends on the specific requirements of the task at hand.
If you need to check for exact matches and efficiency is a concern, the .isin()
method is the way to go. If you need more flexibility in matching criteria or have a smaller DataFrame, a list comprehension might be a better fit.
In any case, Pandas provides many powerful tools for manipulating and analyzing data, and knowing how to efficiently check for values in a column is an essential skill for any data scientist or software engineer.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.