How to Check if One Value Exists in Any Rows of Any Columns in Pandas
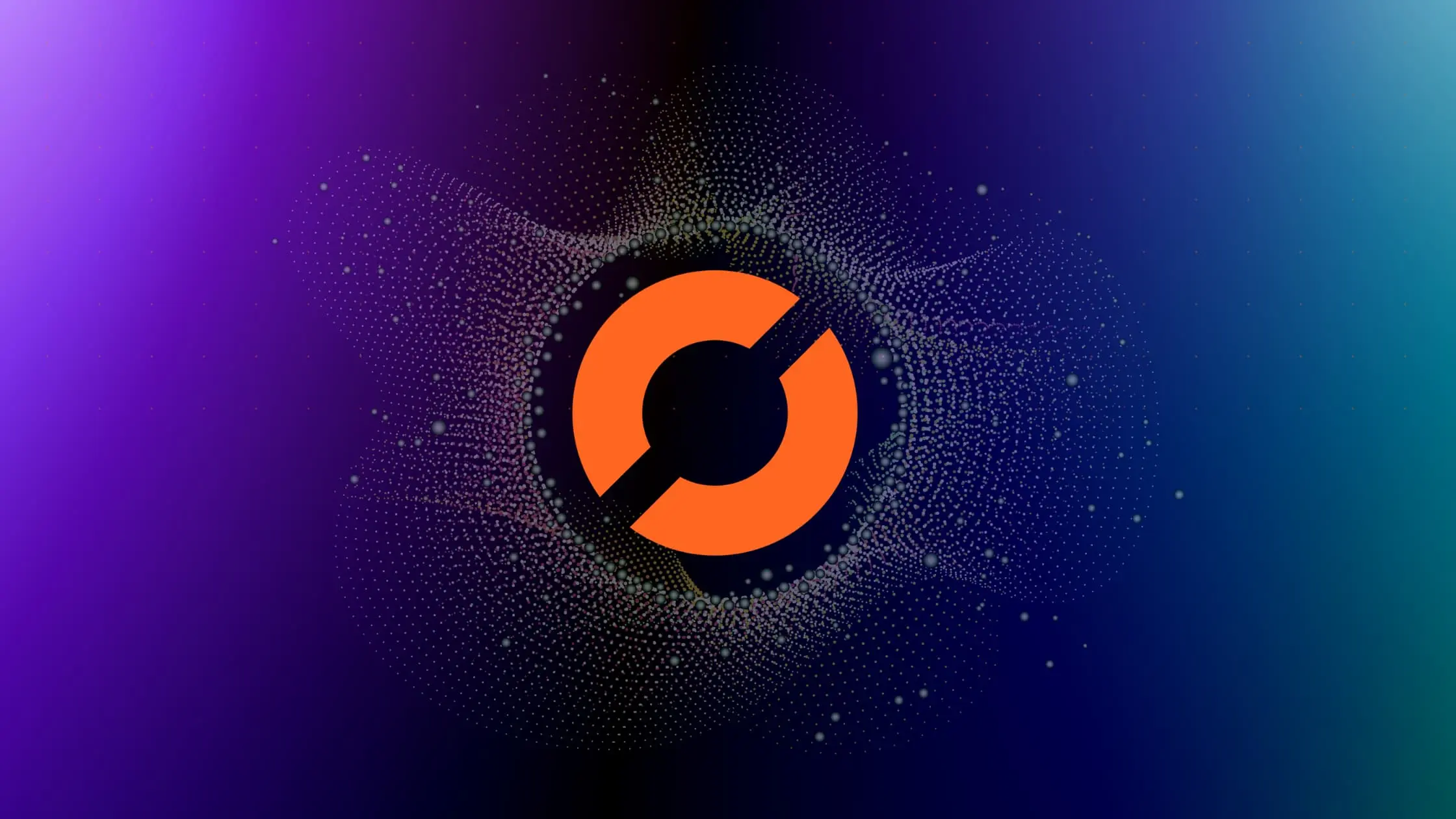
As a data scientist or software engineer, you may have come across a situation where you need to check if one value exists in any rows of any columns in pandas. This is a common task in data analysis and it can be easily accomplished using pandas library. In this blog post, we will discuss different methods to check if a value exists in any rows of any columns in pandas.
Table of Contents
- What is Pandas?
- How to Check if One Value Exists in Any Rows of Any Columns in Pandas?
- Error Handling
- Conclusion
What is Pandas?
Pandas is a Python library used for data manipulation and analysis. It provides data structures for efficiently storing and manipulating large datasets, and tools for data analysis such as filtering, grouping, and merging. Pandas is widely used in data science for tasks such as data cleaning, data transformation, and data visualization.
How to Check if One Value Exists in Any Rows of Any Columns in Pandas?
Pandas provides different methods to check if a value exists in any rows of any columns in a DataFrame. Let’s explore some of the most common methods.
Method 1: Using any() method
The any()
method in pandas returns True if any element in the DataFrame is True, and False otherwise. We can use this method to check if a value exists in any rows of any columns in a DataFrame.
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# Check if value 4 exists in any rows of any columns
if (df == 4).any().any():
print("Value 4 exists in the DataFrame")
else:
print("Value 4 does not exist in the DataFrame")
Output:
Value 4 exists in the DataFrame
In this example, we create a sample DataFrame with three columns A, B, and C. We then check if the value 4 exists in any rows of any columns in the DataFrame using the any()
method. The any()
method is applied twice, once for columns and once for rows, to check if any element in the DataFrame is equal to 4. If the value 4 exists in any rows of any columns, we print “Value 4 exists in the DataFrame”, otherwise, we print “Value 4 does not exist in the DataFrame”.
Method 2: Using isin() method
The isin()
method in pandas returns a Boolean DataFrame showing whether each element in the DataFrame is contained in a list of values. We can use this method to check if a value exists in any rows of any columns in a DataFrame.
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# Check if value 4 exists in any rows of any columns
if df.isin([4]).any().any():
print("Value 4 exists in the DataFrame")
else:
print("Value 4 does not exist in the DataFrame")
Output:
Value 4 exists in the DataFrame
In this example, we create a sample DataFrame with three columns A, B, and C. We then check if the value 4 exists in any rows of any columns in the DataFrame using the isin()
method. We pass a list of values [4] to the isin()
method to check if any element in the DataFrame is equal to 4. If the value 4 exists in any rows of any columns, we print “Value 4 exists in the DataFrame”, otherwise, we print “Value 4 does not exist in the DataFrame”.
Method 3: Using applymap() method
The applymap()
method in pandas applies a function to every element of the DataFrame. We can use this method to check if a value exists in any rows of any columns in a DataFrame.
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# Check if value 4 exists in any rows of any columns
if df.applymap(lambda x: x == 4).any().any():
print("Value 4 exists in the DataFrame")
else:
print("Value 4 does not exist in the DataFrame")
Output:
Value 4 exists in the DataFrame
In this example, we create a sample DataFrame with three columns A, B, and C. We then check if the value 4 exists in any rows of any columns in the DataFrame using the applymap()
method. We apply a lambda function to every element of the DataFrame to check if it is equal to 4. If the value 4 exists in any rows of any columns, we print “Value 4 exists in the DataFrame”, otherwise, we print “Value 4 does not exist in the DataFrame”.
Error Handling
- Case Sensitivity: The comparison operations in Python, including those used in pandas, are case-sensitive. Make sure that the case of the value being searched matches the case of the data in the DataFrame. For example, searching for the value “Value” when the actual data contains “value” might lead to incorrect results.
# Incorrect usage
if (df == 'Value').any().any():
print("Value exists in the DataFrame")
# Correct usage
if (df == 'value').any().any():
print("Value exists in the DataFrame")
- NaN Handling: If your DataFrame contains NaN (Not a Number) values, be aware that comparisons involving NaN can sometimes yield unexpected results. It’s a good practice to handle NaN values explicitly based on your use case.
# Handle NaN values explicitly
if df.isin([4]).any().any() and not pd.isna(4):
print("Value 4 exists in the DataFrame")
else:
print("Value 4 does not exist in the DataFrame")
- Data Types: Ensure that the data types of the DataFrame columns match the data types of the values you are searching for. Unexpected results may occur if you’re comparing, for instance, a string to a numeric value.
# Ensure consistent data types
if df.astype(str).isin(['4']).any().any():
print("Value 4 exists in the DataFrame")
else:
print("Value 4 does not exist in the DataFrame")
- Understanding applymap() Limitations: While the applymap() method is versatile, it might not be the most efficient choice for large datasets. Consider alternative methods like isin() or any() for better performance.
# Efficient alternative to applymap()
if df.isin([4]).any().any():
print("Value 4 exists in the DataFrame")
else:
print("Value 4 does not exist in the DataFrame")
Including notes about these potential issues can help users avoid common pitfalls when working with pandas for data analysis.
Conclusion
In this blog post, we discussed different methods to check if one value exists in any rows of any columns in pandas. We explored the any()
method, isin()
method, and applymap()
method to accomplish this task. These methods are easy to use and can be applied to large datasets efficiently. Pandas provides a rich set of tools for data analysis and data manipulation, which makes it a popular library in the data science community. As a data scientist or software engineer, it is important to have a good understanding of pandas and its capabilities.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.