How to Check if a Variable is either a Python List Numpy Array or Pandas Series
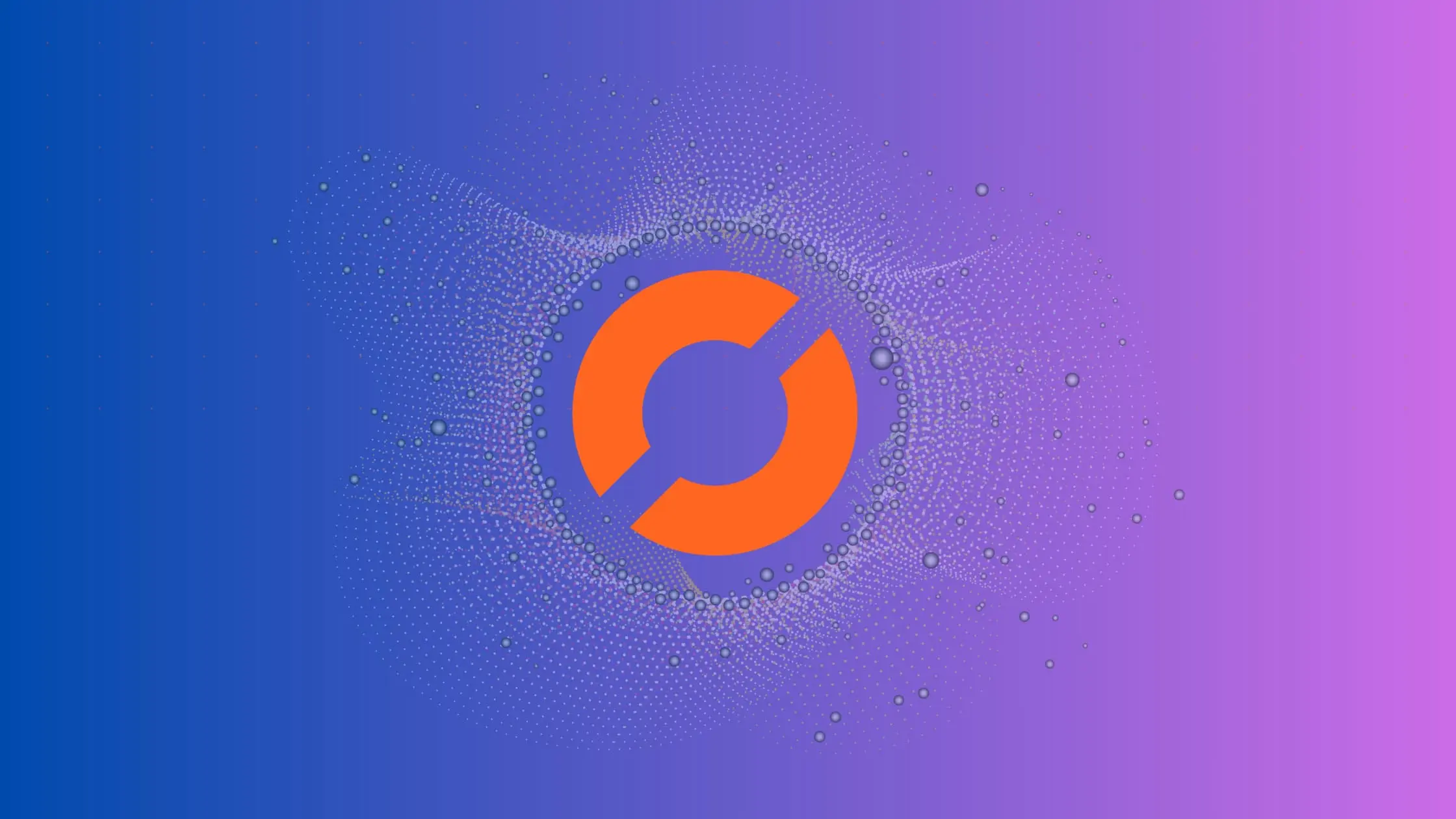
As a data scientist or software engineer, it is common to work with different types of data structures in Python. One of the most frequently used data structures is the list. However, when working with large datasets, it is often necessary to use more efficient data structures such as the numpy array or pandas series. In this article, we will discuss how to check if a variable is either a python list, numpy array, or pandas series.
What is a Python List?
A python list is a built-in data structure that can hold a collection of different data types such as integers, strings, and even other lists. Lists are mutable, meaning that you can add, remove, or modify elements in the list. Lists are created using square brackets []
and separating the elements with commas.
my_list = [1, 2, 3, "four", [5, 6]]
What is a Numpy Array?
A numpy array is a multidimensional array of homogeneous data types. It is more efficient than a python list when working with large datasets because it uses less memory and performs operations faster. Numpy arrays are created using the numpy
library.
import numpy as np
my_array = np.array([1, 2, 3])
What is a Pandas Series?
A pandas series is a one-dimensional labeled array that can hold data of any type. It is built on top of the numpy array and provides additional functionality such as indexing and alignment. Pandas series are created using the pandas
library.
import pandas as pd
my_series = pd.Series([1, 2, 3])
Checking if a Variable is a Python List
To check if a variable is a python list, we can use the isinstance()
function. This function takes two arguments, the variable to check and the type to check against. If the variable is of the specified type, the function returns True
, otherwise, it returns False
.
my_list = [1, 2, 3, "four", [5, 6]]
if isinstance(my_list, list):
print("my_list is a list")
else:
print("my_list is not a list")
Output:
my_list is a list
Checking if a Variable is a Numpy Array
To check if a variable is a numpy array, we can use the isinstance()
function as well as the numpy.ndarray
class. The numpy.ndarray
class is the base class for all numpy arrays.
import numpy as np
my_array = np.array([1, 2, 3])
if isinstance(my_array, np.ndarray):
print("my_array is a numpy array")
else:
print("my_array is not a numpy array")
Output:
my_array is a numpy array
Checking if a Variable is a Pandas Series
To check if a variable is a pandas series, we can use the isinstance()
function as well as the pandas.Series
class. The pandas.Series
class is the base class for all pandas series.
import pandas as pd
my_series = pd.Series([1, 2, 3])
if isinstance(my_series, pd.Series):
print("my_series is a pandas series")
else:
print("my_series is not a pandas series")
Output:
my_series is a pandas series
Checking if a Variable is either a Python List, Numpy Array or Pandas Series
To check if a variable is either a python list, numpy array, or pandas series, we can use the isinstance()
function and check against all three types.
import numpy as np
import pandas as pd
# Define example variables
my_list = [1, 2, 3, "four", [5, 6]]
my_array = np.array([1, 2, 3])
my_series = pd.Series([1, 2, 3])
# Check and display the type of the variable
def check_and_display_type(variable):
if isinstance(variable, list):
print(f"The variable is a Python list: {variable}")
elif isinstance(variable, np.ndarray):
print(f"The variable is a NumPy array: {variable}")
elif isinstance(variable, pd.Series):
print(f"The variable is a Pandas Series: {variable}")
else:
print("The variable is not a recognized type.")
# Test the variables
check_and_display_type(my_list)
check_and_display_type(my_array)
check_and_display_type(my_series)
Output:
The variable is a Python list: [1, 2, 3, 'four', [5, 6]]
The variable is a NumPy array: [1 2 3]
The variable is a Pandas Series: 0 1
1 2
2 3
dtype: int64
Conclusion
In this article, we discussed how to check if a variable is either a python list, numpy array, or pandas series. We first defined what each of these data structures is, then showed how to check if a variable is of a specific type using the isinstance()
function. Finally, we showed how to check if a variable is of any of the three types using the isinstance()
function and checking against all three types. By using these techniques, data scientists and software engineers can ensure that their code works as intended and avoid errors caused by using the wrong data structure.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.